- Describe what values were stored in the array(s) and how you utilized them. Share your experience while using the array(s).
I used arrays to store colors, because it made it easier to then utilize loops to assign the colors to the shapes. I had a lot of colors (to create gradients) so it was the most optimal way to do that.
- Explain when/why translate(), push() and pop().
I used these to center the composition. It made it much more easier to have the center of the canvas as a relative position.
- What is the effectiveness of User-Defined Functions?
- they are reusable (if the function has parameters)
- they make the code look cleaner as it breaks down your process into smaller pieces of code
- you can easily experiment by choosing to call the function multiple times/not to call it
- What parameters did you added to your functions? How were they manipulate the effects of the functions?
Mostly the parameters were the position variables. As I also used translate() it made it easier to calculate the position of the shapes.
- Are you able to identify methods/processes in your programming that are radically changed because of the uses of the functions you defined?
I used the UDF before so I would not say much changed, however I did find translate() very useful when working with composition and passing the position variables into the functions.
This is what i created: 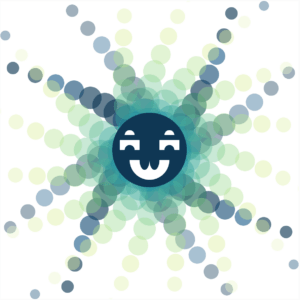
Here is the link: https://editor.p5js.org/zhuanya28/sketches/vIDc_V0OY
There is the code:
function setup() {
createCanvas(600, 600);
}
function draw() {
background(255);
drawCreature(width / 2, height / 2);
}
let tentacleSize = 80;
let yChange = 6;
let colors = [];
let colors2 = [];
function drawTentacle2(x, y, angle) {
rotate(angle);
push();
let currentColor = 0;
for (let i = x; i < x + 200; i += 15) {
colors[currentColor].setAlpha(100);
fill(colors[currentColor]);
if (currentColor < colors.length - 1) {
currentColor++;
} else {
currentColor = 0;
}
noStroke();
circle(i, y, tentacleSize);
y += yChange;
yChange += 4;
tentacleSize -= 5;
}
yChange = 5;
tentacleSize = 80;
pop();
}
function drawTentacle(x, y, angle) {
rotate(angle);
push();
let currentColor = 0;
tentacleSize = 80;
for (let i = x; i < x + 200; i += 15) {
colors2[currentColor].setAlpha(100);
fill(colors2[currentColor]);
if (currentColor < colors2.length - 1) {
currentColor++;
} else {
currentColor = 0;
}
noStroke();
circle(i, y, tentacleSize);
y -= yChange;
yChange += 4;
tentacleSize -= 5;
}
yChange = 5;
tentacleSize = 80;
pop();
}
function colorsDefine() {
// colors[0] = color("#c9e4ca");
// colors[1] = color("#87bba2");
// colors[2] = color("#55828b");
// colors[3] = color("#3b6064");
// colors[4] = color("#364958");
// colors[0] = color("#99e2b4");
// colors[1] = color("#88d4ab");
// colors[2] = color("#78c6a3");
// colors[3] = color("#67b99a");
// colors[4] = color("#56ab91");
// colors[5] = color("#469d89");
// colors[6] = color("#358f80");
// colors[7] = color("#248277");
// colors[8] = color("#14746f");
// colors[9] = color("#036666");
colors[11] = color("#EBF5CA");
colors[10] = color("#DFF0A6");
colors[9] = color("#d9ed92");
colors[8] = color("#b5e48c");
colors[7] = color("#99d98c");
colors[6] = color("#76c893");
colors[5] = color("#52b69a");
colors[4] = color("#34a0a4");
colors[3] = color("#168aad");
colors[2] = color("#1a759f");
colors[1] = color("#1e6091");
colors[0] = color("#184e77");
colors2[0] = color("#103755");
colors2[1] = color("#0D3655");
colors2[2] = color("#0E3757");
colors2[3] = color("#194E77");
colors2[4] = color("#22608F");
// colors[5] = color("PaleVioletRed");
}
function drawHead(x, y) {
colors2[0].setAlpha(255);
fill(colors2[0]);
circle(x, y, 150);
}
function drawFace(x, y) {
noStroke();
fill(255);
rect(x - 60, y - 20, 40, 20);
rect(x + 20, y - 20, 40, 20);
arc(x + 40, y - 30, 20, 20, PI, 0);
arc(x - 40, y - 30, 20, 20, PI, 0);
arc(x, y + 20, 80, 80, 0, PI);
fill(colors2[0]);
arc(x - 40, y, 20, 20, PI, 0);
arc(x + 40, y, 20, 20, PI, 0);
arc(x + 10, y + 20, 20, 40, 0, PI);
arc(x - 10, y + 20, 20, 40, 0, PI);
}
function drawCreature(x, y) {
colorsDefine();
translate(x, y);
for (let i = 0; i < 2 * PI; i += PI / 6) {
drawTentacle(0, 0, i);
}
for (let i = PI / 8; i < 2 * PI; i += PI / 8) {
drawTentacle2(0, 0, i);
}
drawHead(0, 0);
drawFace(0, 0);
}