Reference picture:
The sketch: I have divided the paper into rectangle pieces to map the approximate position of the sketch & outlined the shapes. Given that the chosen picture is already quite geometrical I did not need to do much geometrization myself. 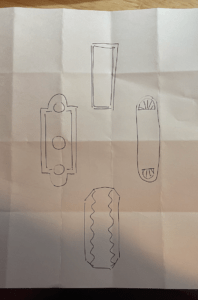
I have utilized quite a lot of shapes in my code, including rect(), circle(), line(), vertex()-based shapes and even sinus function to create the wavy part of the bottom sign.
- Did you prefer exploratory programming, or using the reference? Why? Could you imagine a situation where you would use the other technique?
I was using both! I would usually get some guidelines from the reference website and then continue on changing the parameters of the function myself. I believe this method works best for me as it provides a certain head-start when utilizing new functions.
- In which ways (if any) was drawing on a piece of paper (as we did in our exercise) easier than writing the program?
Well, I didn’t need to think about the positions of the shapes, nor the complexity or color:) so it was definitely easier.
- In which ways (if any) is writing the program easier than drawing on the piece of paper?
I wouldn’t say it is easier but it was way more interesting cause I had color and also the possibility to understand how, for example, the vertex() function works, as well as how I can utilize the sin() when creating the wavy things.
one of the most interesting bits of text would be the wavy part:
noFill();
beginShape();
for (let y = initialY; y <= initialY + 190; y += 1) {
let x = map(sin(y * 0.2), -1, 1, initialX + 5, initialX - 5);
vertex(x, y);
}
endShape();
let initialX1 = width / 2 - sizeOfSign / 2 + 81;
let initialY1 = height / 2 + 70 - 10;
beginShape();
for (let y = initialY1; y <= initialY1 + 190; y += 1) {
let x = map(sin(y * 0.2), -1, 1, initialX1 + 5, initialX1 - 5);
vertex(x, y);
}
endShape();
the shape of the right one (with the lines and stuff):
fill(36, 39, 223);
rect(width / 2 + sizeOfSign, height / 2 - 100, sizeOfSign, 200);
circle(width / 2 + sizeOfSign + 50, height / 2 - 100, 100);
circle(width / 2 + sizeOfSign + 50, height / 2 + 100, 100);
stroke(128, 244, 255);
circle(width / 2 + sizeOfSign + 50, height / 2 - 100, 90);
circle(width / 2 + sizeOfSign + 50, height / 2 + 100, 90);
rect(width / 2 + sizeOfSign + 5, height / 2 - 100, sizeOfSign - 10, 200);
stroke(252, 194, 252);
//upper part
line(
width / 2 + sizeOfSign + 50,
height / 2 - 100,
width / 2 + sizeOfSign + 50 - 40,
height / 2 - 100 - 20
);
line(
width / 2 + sizeOfSign + 50,
height / 2 - 100,
width / 2 + sizeOfSign + 50 - 25,
height / 2 - 100 - 37
);
line(
width / 2 + sizeOfSign + 50,
height / 2 - 100,
width / 2 + sizeOfSign + 50,
height / 2 - 100 - 45
);
line(
width / 2 + sizeOfSign + 50,
height / 2 - 100,
width / 2 + sizeOfSign + 50 + 25,
height / 2 - 100 - 37
);
line(
width / 2 + sizeOfSign + 50,
height / 2 - 100,
width / 2 + sizeOfSign + 50 + 40,
height / 2 - 100 - 20
);
//lower part
line(
width / 2 + sizeOfSign + 50,
height / 2 + 100,
width / 2 + sizeOfSign + 50 - 40,
height / 2 + 100 + 20
);
line(
width / 2 + sizeOfSign + 50,
height / 2 + 100,
width / 2 + sizeOfSign + 50 - 25,
height / 2 + 100 + 37
);
line(
width / 2 + sizeOfSign + 50,
height / 2 + 100,
width / 2 + sizeOfSign + 50,
height / 2 + 100 + 45
);
line(
width / 2 + sizeOfSign + 50,
height / 2 + 100,
width / 2 + sizeOfSign + 50 + 25,
height / 2 + 100 + 37
);
line(
width / 2 + sizeOfSign + 50,
height / 2 + 100,
width / 2 + sizeOfSign + 50 + 40,
height / 2 + 100 + 20
);
and the shape of the left one:
fill(200, 44, 0);
rect(width / 2 - sizeOfSign * 2, height / 2 - 100, sizeOfSign, 200);
circle(width / 2 - sizeOfSign * 1.5, height / 2 - 100 + 5, sizeOfSign - 15);
circle(width / 2 - sizeOfSign * 1.5, height / 2 + 100 - 5, sizeOfSign - 15);
strokeWeight(2);
stroke(255, 255, 0);
rect(
width / 2 - sizeOfSign * 2 + 5,
height / 2 - 100 + 5,
sizeOfSign - 10,
190
);
circle(width / 2 - sizeOfSign * 1.5, height / 2 - 100 + 5, sizeOfSign - 25);
circle(width / 2 - sizeOfSign * 1.5, height / 2 + 100 - 5, sizeOfSign - 25);
noStroke();
fill(200, 44, 0);
rect(
width / 2 - sizeOfSign * 2 + 11,
height / 2 - 100 + 5,
sizeOfSign - 20,
190
);
strokeWeight(2);
stroke(255, 205, 243);
fill(152, 28, 186);
rect(
width / 2 - sizeOfSign * 2 + 12.5,
height / 2 - 90,
sizeOfSign - 25,
180
);
noStroke();
fill(200, 44, 0);
circle(width / 2 - sizeOfSign * 1.5, height / 2 - 100 + 10, sizeOfSign - 35);
circle(width / 2 - sizeOfSign * 1.5, height / 2 - 100 + 100, sizeOfSign - 35);
circle(width / 2 - sizeOfSign * 1.5, height / 2 - 100 + 190, sizeOfSign - 35);
I am pretty satisfied with how this experiment turned out. Even though I spent unreasonable time working on some of the parts, I believe that the outcome was worth it! You can see the screenshots and the code below:) P.S. I could not decide which background color works here) even tried blue, green, red, grey, etc. decided to attach black and white here:)
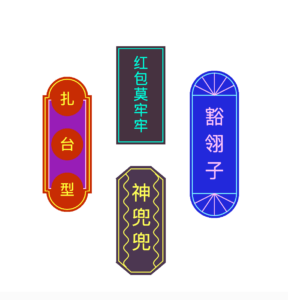
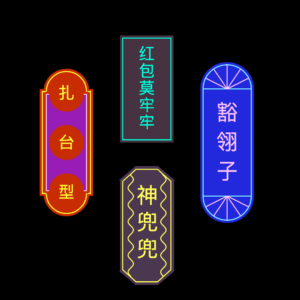
function setup() {
createCanvas(600, 600);
background(255);
}
let sizeOfSign = 100;
function theTopOne() {
fill(70, 50, 65);
noStroke();
rect(width / 2 - sizeOfSign / 2, height / 6, sizeOfSign, 200);
//the outline
strokeWeight(2);
stroke(0, 255, 218);
rect(width / 2 - sizeOfSign / 2 + 5, height / 6 + 5, sizeOfSign - 10, 190);
noStroke();
let s = "红包莫牢牢";
textSize(30);
fill(0, 255, 218);
text(s[0], width / 2 - sizeOfSign / 2 + 35, height / 6 + 20, 100, 200);
text(s[1], width / 2 - sizeOfSign / 2 + 35, height / 6 + 52, 100, 200);
text(s[2], width / 2 - sizeOfSign / 2 + 35, height / 6 + 84, 100, 200);
text(s[3], width / 2 - sizeOfSign / 2 + 35, height / 6 + 116, 100, 200);
text(s[4], width / 2 - sizeOfSign / 2 + 35, height / 6 + 148, 100, 200);
}
function theLeftOne() {
fill(200, 44, 0);
rect(width / 2 - sizeOfSign * 2, height / 2 - 100, sizeOfSign, 200);
circle(width / 2 - sizeOfSign * 1.5, height / 2 - 100 + 5, sizeOfSign - 15);
circle(width / 2 - sizeOfSign * 1.5, height / 2 + 100 - 5, sizeOfSign - 15);
strokeWeight(2);
stroke(255, 255, 0);
rect(
width / 2 - sizeOfSign * 2 + 5,
height / 2 - 100 + 5,
sizeOfSign - 10,
190
);
circle(width / 2 - sizeOfSign * 1.5, height / 2 - 100 + 5, sizeOfSign - 25);
circle(width / 2 - sizeOfSign * 1.5, height / 2 + 100 - 5, sizeOfSign - 25);
noStroke();
fill(200, 44, 0);
rect(
width / 2 - sizeOfSign * 2 + 11,
height / 2 - 100 + 5,
sizeOfSign - 20,
190
);
strokeWeight(2);
stroke(255, 205, 243);
fill(152, 28, 186);
rect(
width / 2 - sizeOfSign * 2 + 12.5,
height / 2 - 90,
sizeOfSign - 25,
180
);
noStroke();
fill(200, 44, 0);
circle(width / 2 - sizeOfSign * 1.5, height / 2 - 100 + 10, sizeOfSign - 35);
circle(width / 2 - sizeOfSign * 1.5, height / 2 - 100 + 100, sizeOfSign - 35);
circle(width / 2 - sizeOfSign * 1.5, height / 2 - 100 + 190, sizeOfSign - 35);
let s = "扎台型";
fill(255, 255, 0);
text(s[0], width / 2 - sizeOfSign * 1.5 - 15, height / 2 - 100 + 17.5);
text(s[1], width / 2 - sizeOfSign * 1.5 - 15, height / 2 - 100 + 110);
text(s[2], width / 2 - sizeOfSign * 1.5 - 15, height / 2 - 100 + 202.5);
}
function theRightOne() {
fill(36, 39, 223);
rect(width / 2 + sizeOfSign, height / 2 - 100, sizeOfSign, 200);
circle(width / 2 + sizeOfSign + 50, height / 2 - 100, 100);
circle(width / 2 + sizeOfSign + 50, height / 2 + 100, 100);
stroke(128, 244, 255);
circle(width / 2 + sizeOfSign + 50, height / 2 - 100, 90);
circle(width / 2 + sizeOfSign + 50, height / 2 + 100, 90);
rect(width / 2 + sizeOfSign + 5, height / 2 - 100, sizeOfSign - 10, 200);
stroke(252, 194, 252);
//upper part
line(
width / 2 + sizeOfSign + 50,
height / 2 - 100,
width / 2 + sizeOfSign + 50 - 40,
height / 2 - 100 - 20
);
line(
width / 2 + sizeOfSign + 50,
height / 2 - 100,
width / 2 + sizeOfSign + 50 - 25,
height / 2 - 100 - 37
);
line(
width / 2 + sizeOfSign + 50,
height / 2 - 100,
width / 2 + sizeOfSign + 50,
height / 2 - 100 - 45
);
line(
width / 2 + sizeOfSign + 50,
height / 2 - 100,
width / 2 + sizeOfSign + 50 + 25,
height / 2 - 100 - 37
);
line(
width / 2 + sizeOfSign + 50,
height / 2 - 100,
width / 2 + sizeOfSign + 50 + 40,
height / 2 - 100 - 20
);
//lower part
line(
width / 2 + sizeOfSign + 50,
height / 2 + 100,
width / 2 + sizeOfSign + 50 - 40,
height / 2 + 100 + 20
);
line(
width / 2 + sizeOfSign + 50,
height / 2 + 100,
width / 2 + sizeOfSign + 50 - 25,
height / 2 + 100 + 37
);
line(
width / 2 + sizeOfSign + 50,
height / 2 + 100,
width / 2 + sizeOfSign + 50,
height / 2 + 100 + 45
);
line(
width / 2 + sizeOfSign + 50,
height / 2 + 100,
width / 2 + sizeOfSign + 50 + 25,
height / 2 + 100 + 37
);
line(
width / 2 + sizeOfSign + 50,
height / 2 + 100,
width / 2 + sizeOfSign + 50 + 40,
height / 2 + 100 + 20
);
let s = "豁翎子";
noStroke();
fill(252, 194, 252);
textSize(40);
text(s[0], width / 2 + sizeOfSign + 30, height / 2 - 100 + 25, 100, 200);
text(s[1], width / 2 + sizeOfSign + 30, height / 2 - 100 + 80, 100, 200);
text(s[2], width / 2 + sizeOfSign + 30, height / 2 - 100 + 135, 100, 200);
}
function theBottomOne() {
fill(76, 55, 82);
stroke(76, 55, 82);
beginShape();
vertex(width / 2 - sizeOfSign / 2, height / 2 + 70);
vertex(width / 2 - sizeOfSign / 2 + 30, height / 2 + 45);
vertex(width / 2 - sizeOfSign / 2 + 70, height / 2 + 45);
vertex(width / 2 - sizeOfSign / 2 + 100, height / 2 + 70);
vertex(width / 2 - sizeOfSign / 2 + 100, height / 2 + 240);
vertex(width / 2 - sizeOfSign / 2 + 70, height / 2 + 265);
vertex(width / 2 - sizeOfSign / 2 + 30, height / 2 + 265);
vertex(width / 2 - sizeOfSign / 2, height / 2 + 240);
endShape();
stroke(254, 254, 70);
strokeWeight(2);
beginShape();
vertex(width / 2 - sizeOfSign / 2 + 5, height / 2 + 70 + 2.5);
vertex(width / 2 - sizeOfSign / 2 + 30, height / 2 + 45 + 5);
vertex(width / 2 - sizeOfSign / 2 + 70, height / 2 + 45 + 5);
vertex(width / 2 - sizeOfSign / 2 + 100 - 5, height / 2 + 70 + 2.5);
vertex(width / 2 - sizeOfSign / 2 + 100 - 5, height / 2 + 240 - 2.5);
vertex(width / 2 - sizeOfSign / 2 + 70, height / 2 + 265 - 5);
vertex(width / 2 - sizeOfSign / 2 + 30, height / 2 + 265 - 5);
vertex(width / 2 - sizeOfSign / 2 + 5, height / 2 + 240 - 2.5);
vertex(width / 2 - sizeOfSign / 2 + 5, height / 2 + 70 + 2.5);
endShape();
// rect(width/2-sizeOfSign/2, height/2+50, sizeOfSign, 200);
let initialX = width / 2 - sizeOfSign / 2 + 20;
let initialY = height / 2 + 70 - 10;
point(initialX, initialY);
noFill();
beginShape();
for (let y = initialY; y <= initialY + 190; y += 1) {
let x = map(sin(y * 0.2), -1, 1, initialX + 5, initialX - 5);
vertex(x, y);
}
endShape();
let initialX1 = width / 2 - sizeOfSign / 2 + 81;
let initialY1 = height / 2 + 70 - 10;
beginShape();
for (let y = initialY1; y <= initialY1 + 190; y += 1) {
let x = map(sin(y * 0.2), -1, 1, initialX1 + 5, initialX1 - 5);
vertex(x, y);
}
endShape();
let s = "神兜兜";
noStroke();
fill(254, 254, 70);
text(s[0], initialX1 - 50, initialY1 + 20, 100, 200);
text(s[1], initialX1 - 50, initialY1 + 70, 100, 200);
text(s[1], initialX1 - 50, initialY1 + 120, 100, 200);
}
function draw() {
theTopOne();
theLeftOne();
theRightOne();
theBottomOne();
}