Recitation Exercise:
For this week’s project, I simply built on the painting I created last week. Now, the object originally on the right will be able to move around the canvas following my mouse. What the user needs to do is to fit it back into the original position. When they successfully complete this task, the color of the entire painting will change to its contrast (which I find even better than the original colors). Meanwhile, users would be able to see the message of “Perfect Match!” in the console. There is another detail, which is that the other object with the same shape of the object the user is controlling, will not display on the screen at the very beginning. By adjusting the alpha value, I made it more evident when the user is getting closer to the original position. The functions I have used include pushMatrix, translate, popMatrix, mouseX, mouseY etc. You can find the code below.
void setup(){ size (600, 600); colorMode(RGB); noStroke(); } void draw(){ background (133, 97, 50, 150); pushMatrix(); translate(mouseX, mouseY); fill (0, 0, 0); quad (-275, -125, -225, -25, 225, -25, 175, -125); fill (248, 231, 14, 125); quad (-45, -205, 50, 45, 100, 45, 5, -205); fill (215, 215, 215, 150); quad (-105, -85, -255, 105, -35, 105, 115, -85); fill (255, 0, 0, 100); ellipse (65, -125, 100, 100); popMatrix(); fill (100, 100, 100, mouseX-200); quad (45, 350, 65, 385, 250, 385, 225, 350); fill (248, 231, 14, mouseY-200); quad (125, 320, 165, 420, 190, 420, 150, 320); fill (30, 30, 30, mouseX-125); quad (135, 360, 60, 460, 135, 460, 210, 360); fill (255, 0, 0, mouseY-195); ellipse (168, 347, 42, 42); if ((mouseX > 315) && (mouseY > 315) && (mouseX < 335) && (mouseY < 335)){ background (122, 158, 205, 150); fill (255, 255, 255); quad (50, 200, 100, 300, 550, 300, 500, 200); fill (7, 24, 241, 125); quad (280, 120, 375, 370, 425, 370, 330, 120); fill (40, 40, 40, 150); quad (220, 240, 70, 430, 290, 430, 440, 240); fill (0, 255, 255, 100); ellipse (380, 200, 100, 100); fill (155, 155, 155, 125); quad (45, 350, 65, 385, 250, 385, 225, 350); fill (7, 24, 241, 125); quad (125, 320, 165, 420, 190, 420, 150, 320); fill (225, 225, 225, 100); quad (135, 360, 60, 460, 135, 460, 210, 360); fill (0, 255, 255, 130); ellipse (168, 347, 42, 42); println(mouseX, mouseY); println("Perfect Match!"); } }
Additional Recitation Homework:
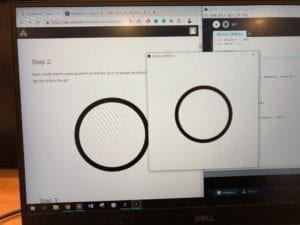
In this homework, it is very important to remember using the HSB volor mode instead of RGB.
The code for homework is here:
float diameter = 100; float speed = 2; int count = 0; int x,y; void setup(){ size (600, 600); frameRate(110); colorMode(HSB); x = width/2; y = height/2; } void keyPressed(){ if (key == CODED){ if (keyCode == UP){ y = y - 10; } if (keyCode == DOWN){ y = y + 10; } if (keyCode == LEFT){ x = x - 10; } if (keyCode == RIGHT){ x = x + 10; } } } void draw(){ background (255); ellipse (x, y, diameter, diameter); strokeWeight (20); stroke(count, 255, 255); count = count + 1; if (count > 255){ count = count - 255; } circleMove(); circleBounce(); } void circleMove(){ diameter = diameter + speed; } void circleBounce(){ if ((diameter > 350) || (diameter < 100)){ speed = -speed; } }
Overall Comments:
The most useful functions I have used in this recitation is pushMatrix/translate/popMatrix. As you can see in my project, I need to move around the parallelograms and circles a whole. This goal is much easier to achieve if I put them in a Matrix and change the center. Also, the HSB color mode is also a very interesting feature because this is the only way to make an object flow in so many different colors. The drawback for HSB is that we cannot tell the components of the color very directly. For a specific color, we should still use the RGB mode. In the future, I would like to try frameRate and frameCount function. I think they will be interesting to use, too.