Exercises
1.Make a Processing Etch A Sketch
Prompt: For this exercise, use Arduino to send two analog values to Processing via serial communication. To do this, build a circuit with two potentiometers and write an Arduino sketch that reads their values and sends them serially. Then write a Processing sketch that draws an ellipse and reads those two analog values from Arduino. This sketch should modify the ellipseās x and y values based on the input from Arduino. Just like an Etch A Sketch, one potentiometer should control the ellipseās x-axis movement, and the other should control the ellipseās y-axis movement.
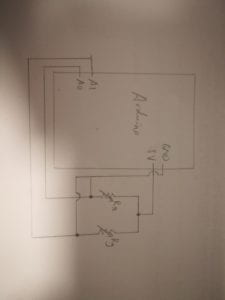
My code for Arduino:
void setup() {
Serial.begin(9600);
}
void loop() {
int sensor1 = analogRead(A0);
int sensor2 = analogRead(A1);
Serial.print(sensor1);
Serial.print(“,”);
Serial.print(sensor2);
Serial.println();
delay(100);
}
My code for Processing:
int x = 0;
int y = 0;
import processing.serial.*;
String myString = null;
Serial myPort;
int NUM_OF_VALUES = 2;
int[] sensorValues;
void setup() {
size(500, 500);
background(255);
setupSerial();
}
void draw() {
updateSerial();
printArray(sensorValues);
fill(#2C6EF0);
ellipse(x, y, 100, 100);
x = sensorValues[0];
y = sensorValues[1];
}
void setupSerial() {
printArray(Serial.list());
myPort = new Serial(this, Serial.list()[ 1 ], 9600);
myPort.clear();
myString = myPort.readStringUntil( 10 ); // 10 = ‘\n’ Linefeed in ASCII
myString = null;
sensorValues = new int[NUM_OF_VALUES];
}
void updateSerial() {
while (myPort.available() > 0) {
myString = myPort.readStringUntil( 10 ); // 10 = ‘\n’ Linefeed in ASCII
if (myString != null) {
String[] serialInArray = split(trim(myString), “,”);
if (serialInArray.length == NUM_OF_VALUES) {
for (int i=0; i<serialInArray.length; i++) {
sensorValues[i] = int(serialInArray[i]);
}
}
}
}
}
2. Make a musical instrument with Arduino
Prompt: Write a Processing sketch that sends values to your Arduino based on your mouseās x and y positions and/or keyboard interactions. Then, make a circuit with your Arduino and a buzzer. The corresponding Arduino code should read the serial values from Processing and translate them into frequency and duration for a tone, which will be sounded by the buzzer.
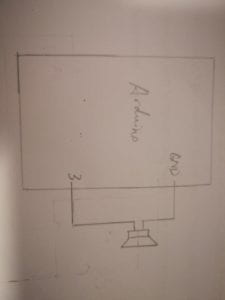
My code for Arduino:
char valueFromProcessing;
void setup() {
Serial.begin(9600);
pinMode(3, OUTPUT);
}
void loop() {
while (Serial.available()) {
valueFromProcessing = Serial.read();
}
if (valueFromProcessing == ‘1’) {
tone(3, 16.35);
} else if (valueFromProcessing == ‘2’) {
tone(3, 18.35);
} else if (valueFromProcessing == ‘3’) {
tone(3, 20.60);
}
delay(10);
My code for Processing:
import processing.serial.*;
Serial myPort;
int valueFromArduino;
void setup() {
size(500, 500);
background(0);
printArray(Serial.list());
myPort = new Serial(this, Serial.list()[ 1 ], 9600);
}
void draw() {
if (keyPressed) {
if (key == ‘c’ || key == ‘C’);{
myPort.write(‘1’);
}
if (key == ‘d’ || key == ‘D’) {
myPort.write(‘2’);
}
if (key == ‘e’ || key == ‘E’);{
myPort.write(‘3’);
}
}
}