InSunday’s recitation, I used two potentiometer to alter the size and speed of a dog video, using serial communication from Arduino to processing. I began the project by writing the processing code to import my video clip, initiate the video loop, and print out the video in a draw loop. In order to make the size and speed of the video contingent on the values sent from two potentiometers, I created a speed variable and a size variable. I mapped sensorValues from index 0 and index 1 to these two variables and sets the speed and size of the video. In Arduino, I double checked that value of sensor 1 will come from pin A0 and value of sensor 2 will come from pin A1.
Connecting with the Computer Vision for Artist and Designers article, technology was used in my project to alter images in ways that cannot simply be observed by the human eyes. Through technology, I was able to see the dog video clip in speed and sizes beyond the scope of my eyes. On one hand, technology expands the limit and possibility of moving images. However, on the other hand, it makes me think about how technology has been altering the way human beings engage and encounter the physical world, through filter of computers. We see the world through a computer vision, as argued in the Computer Vision for Artist and Designers essay, and sometimes we might not even be aware that the way we see the world has been filtered through computer’s “eyes”. It makes me think that the designer and artist using technology should be more intensional when making choices to alter or augment images with the aid from computers.
Below is video and photo documentations of my project
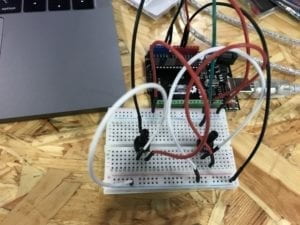
Below is my arduino code
void setup() {
Serial.begin(9600);
}
void loop() {
int sensor1 = analogRead(A0);
int sensor2 = analogRead(A1);
// keep this format
Serial.print(sensor1);
Serial.print(“,”);
Serial.print(sensor2);
Serial.println(); // add linefeed after sending the last sensor value
// too fast communication might cause some latency in Processing
// this delay resolves the issue.
delay(100);
}
Below is my processing code
import processing.serial.*;
import processing.video.*;
Movie dog;
String myString = null;
Serial myPort;
int NUM_OF_VALUES = 2; /** YOU MUST CHANGE THIS ACCORDING TO YOUR PROJECT **/
int[] sensorValues;/** this array stores values from Arduino **/ //Getting sensorValues array
void setup() {
size(1000, 1000);
background(0);
setupSerial();
dog = new Movie(this, “dog.mp4”);
dog.loop();
imageMode(CENTER);
}
void draw() {
background(0);
updateSerial();
printArray(sensorValues);
if (dog.available()) {
dog.read();
}
float s = map(sensorValues[1], 0, 1023, 0, 5); //reading values from index 1 from the array; potentiometor 1
dog.speed(s);
float size = map(sensorValues[0], 0, 1023, 0, width); //reading values from index 0 from the array; potentiometor 2
image(dog, width/2, height/2, size, size);
}
void setupSerial() {
printArray(Serial.list());
myPort = new Serial(this, Serial.list()[ 1 ], 9600);
myPort.clear();
// Throw out the first reading,
// in case we started reading in the middle of a string from the sender.
myString = myPort.readStringUntil( 10 ); // 10 = ‘\n’ Linefeed in ASCII
myString = null;
sensorValues = new int[NUM_OF_VALUES];
}
void updateSerial() {
while (myPort.available() > 0) {
myString = myPort.readStringUntil( 10 ); // 10 = ‘\n’ Linefeed in ASCII
if (myString != null) {
String[] serialInArray = split(trim(myString), “,”);
if (serialInArray.length == NUM_OF_VALUES) {
for (int i=0; i<serialInArray.length; i++) {
sensorValues[i] = int(serialInArray[i]); // [10, 40, 25]
}
}
}
}
}