Then, discuss what you wanted to draw in Processing and your method of achieving that goal. Consider how your final creation is linked to the motif, and in what ways it is similar and different. Do you think that drawing in Processing was a good means of realizing your design?
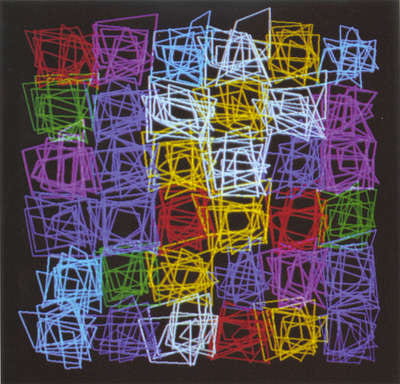
This is a piece of artwork I saw online by Vera Molnar. I browsed through all the suggested website and I picked this one because it looks least like what I previously imagined a computer can draw. Every other digital artwork is symmetric and futuristic, just like the designs and patterns one would see in a fiction movie. For anyone who doesn’t have any knowledge of what computers are really capable of will expect such a pattern to be generated by a computer. However, in this piece of work, through all the cluttered lines laying out in squares but these squares are aligned neatly at the center of the canvas, I can actually feel the emotions in such arrangement as if two feelings are tangling together. One explanation could be that messy lines represent one’s struggling while the aligned squares represent the choices he/she has to make. Therefore, I chose to recreate such a feeling through my own code.
To create such mixed feelings, I decided that I don’t want the lines in the squares to be pre-coded because it would ruin the strong twist the picture contained. They have to be randomized so that they can be in contrast with the well-organized squares. Since I did not know a lot about programming in java, I asked one of the learning assistants. However, she told me a random function can never be called outside of draw() and it has to be called inside another function. According to her, if I ever want to draw a static picture, I never am going to generate anything randomly. However, I don’t really believe so because I thought I was only about a number. So I continued searching and tried assigning the value Math.random() returns to a variable I defined and it worked! I told her that even in a static mode, a random function can be called only once. She realized I was right and called me resilient 🙂 After all, I had to find a way because this is all that matters in this art piece.
Making sure that a line can be generated randomly, I first need to decide how I can color each square with different colors. After noticing that in a single square, all the lines are linked end-to-end, I came up with a program that iterates multiple times. First, the program is going to iterate over the six squares horizontally and vertically, so that all the squares in the pattern will have a go when it comes to generating lines in them. Finally, in every square, it will loop 10 times to select a point and link the last point to it–of course, it will start with selecting 2 points
After coloring, it looked like this:
Now the colors are also randomly generated by the computer, and the whole work looks somewhat like Molnar’s original work. Here is code 1.1:
size(1400, 950); background(200); for(int i=400;i<=900;i = i + 100){ for (int k=175;k<=675;k = k + 100){ int red = (int)(Math.random()*255); int gr = (int)(Math.random()*255); int bl = (int)(Math.random()*255); stroke(red, gr, bl); strokeWeight(2); int a = (int)(Math.random()*150) + i - 25; int b = (int)(Math.random()*150) + k - 25; int c = (int)(Math.random()*150) + i - 25; int d = (int)(Math.random()*150) + k - 25; line(a, b, c, d); for (int j=1;j<=10;j = j + 1){ a = (int)(Math.random()*150) + i - 25; b = (int)(Math.random()*150) + k - 25; line(c, d, a, b); c = (int)(Math.random()*150) + i - 25; d = (int)(Math.random()*150) + k - 25; line(a, b, c, d); }}}
As a reader can see, although I let every square to occupy 100*100 pixels, I am allowing the points to pop out in the 150*150-pixel area around the area of the square because I want the lines of different squares to intersect a bit, or the whole thing would look segmented and boring.
However, when comparing the mine and the original piece, I can see that every square in her work is obviously more “angular” because even if the lines are messy, they are still following the pattern of going around the square in the same direction, while mine is just random lines going around everywhere. So I thought of a way to refine my code.
To make all the lines go in one direction to form a closed square, I figured I could let the computer choose random points on the four sides clockwise:
Following is code 2.0 that includes this feature:
size(1400, 950); background(200); for(int i=400;i<=900;i = i + 100){ for (int k=175;k<=675;k = k + 100){ int red = (int)(Math.random()*255); int gr = (int)(Math.random()*255); int bl = (int)(Math.random()*255); stroke(red, gr, bl); int a = (int)(Math.random()*150) + i - 25; int b = (int)(Math.random()*20) + k - 25; int c = (int)(Math.random()*20) + i + 105; int d = (int)(Math.random()*150) + k - 25; strokeWeight(2); line(a, b, c, d); for (int j=1;j<=10;j = j + 1){ a = (int)(Math.random()*150) + i - 25; b = (int)(Math.random()*20) + k + 105; line(c, d, a, b); c = (int)(Math.random()*20) + i - 25; d = (int)(Math.random()*150) + k - 25; line(a, b, c, d); a = (int)(Math.random()*150) + i - 25; b = (int)(Math.random()*20) + k - 25; line(c, d, a, b); c = (int)(Math.random()*20) + i + 105; d = (int)(Math.random()*150) + k - 25; line(a, b, c, d); }}}
Now if you run the code, the lines will be organized in a shape that look very much like squares, although not every line will necessarily go clockwise.
However, after everything is properly organized, I feel the pattern is too dull. When I look at my “copycat”, I can’t feel the contrary two feelings in Molnar’s work. I think it is because that I overdid the organizing and the lines are not systematically random now. Therefore, I came up with an idea that adds some “rebels” in code 2.1:
size(1400, 950); background(200); for(int i=400;i<=900;i = i + 100){ for (int k=175;k<=675;k = k + 100){ int red = (int)(Math.random()*255); int gr = (int)(Math.random()*255); int bl = (int)(Math.random()*255); stroke(red, gr, bl); strokeWeight(2); int a = (int)(Math.random()*150) + i - 25; int b = (int)(Math.random()*20) + k - 25; int c = (int)(Math.random()*20) + i + 105; int d = (int)(Math.random()*150) + k - 25; line(a, b, c, d); for (int j=1;j<=12;j = j + 1){ if (j==5 || j==10){ a = (int)(Math.random()*250) + i - 75; b = (int)(Math.random()*70) + k + 105; line(c, d, a, b); c = (int)(Math.random()*70) + i - 75; d = (int)(Math.random()*250) + k - 75; line(a, b, c, d); a = (int)(Math.random()*250) + i - 75; b = (int)(Math.random()*70) + k - 75; line(c, d, a, b); c = (int)(Math.random()*70) + i + 105; d = (int)(Math.random()*250) + k - 75; line(a, b, c, d); }else{ a = (int)(Math.random()*150) + i - 25; b = (int)(Math.random()*20) + k + 105; line(c, d, a, b); c = (int)(Math.random()*20) + i - 25; d = (int)(Math.random()*150) + k - 25; line(a, b, c, d); a = (int)(Math.random()*150) + i - 25; b = (int)(Math.random()*20) + k - 25; line(c, d, a, b); c = (int)(Math.random()*20) + i + 105; d = (int)(Math.random()*150) + k - 25; line(a, b, c, d); }}}}
When you run the code, it looks like this:
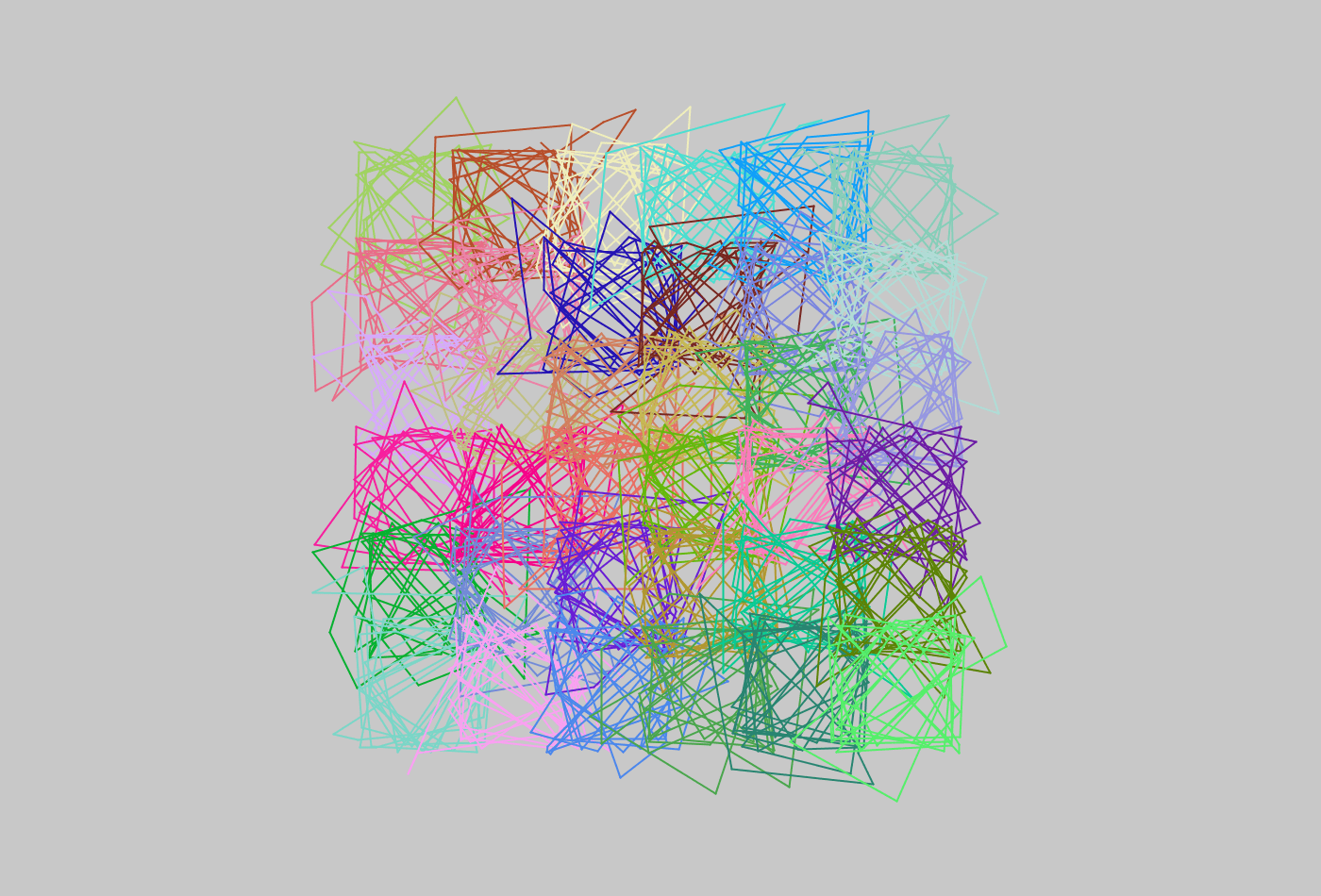
Now, instead of every point has to stay perfectly in the 20*150-pixel box to let the square look recognizable and angular, I am allowing the 5th and the 10th point to get loose a little bit so that the patterns will look a bit wilder. So it makes my final version of the code. I think the final work reflects the emotion I want to express. The messy lines represent the troubling thoughts and the squares imply that while one is experiencing some negative emotions, he/she is also trapped in the social framework or others’ opinions on him/her. One thing that makes this work particularly fun is that when you use the random function in Java, every time you press the run button you’ll get a different result.