The motif that I chose for this project was the below image, Penetrating (B) by Josef Albers.
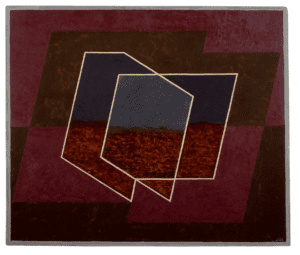
I chose this image because I wanted to draw something that resembles the musician’s album that has city-like mood. In order to do so, instead of parallelogram which gives more of classic look, using the for-loop, I decided to draw a building-like structure below the line above. In addition, I drew one big rectangle as the outline of the album.
In order to maximize the efficiency of time, I used the mouseClicked() function in the processing to select two points to draw lines, and print out the function in the console, therefore the only thing that I would have to do after is copying the code from the console. After drawing all the lines, I copied the code from the console. (The following code bit is found below).
*Caution: while drawing the lines (drawing), the basicBackground() function must be in the draw-loop with the gradient commented out*
void mouseClicked(){
started = true;
if (! clicked){ // if there is no previously selected point
println(“clicked”);
linePos[0] = pmouseX;
linePos[1] = pmouseY;
clicked = true; // because we just selected one point, change the bool val.
} else {
println(“clicked2”);
linePos[2] = pmouseX;
linePos[3] = pmouseY;
clicked = false; // because we are done selecting two points, initialize
println(“line(“, linePos[0], “,”, linePos[1], “,”, linePos[2], “,”, linePos[3], “)”);
}
//println(pmouseX, pmouseY);
}
However, did not really like the placement of the image, therefore, added the shiftX and shiftY to the lines like below
line( 291 +shiftX, 168 +shiftY, 373 +shiftX, 168 +shiftY);
and added the keyPressed function, moved the drawings.
void keyPressed() {
if (key == CODED) {
if (keyCode == UP) {
shiftY -= 5;
} else if (keyCode == DOWN) {
shiftY += 5;
} else if (keyCode == LEFT) {
shiftX -= 5;
} else if (keyCode == RIGHT) {
shiftX += 5;
}
println(shiftX, shiftY);
}
}
In addition, in order to generate bunch of buildings that will be in the photo, I wrote below code bit.
void drawBuilding(){
float buildingPos = 25;
float buildingWidth = 0;
float buildingHeight = 0;
while((buildingPos + buildingWidth) < (width – 25)){
buildingWidth = random(20, 50);
buildingHeight = random(90, 250);
float r = random(0, 150);
float g = random(0, 150);
float b = random(0, 150);
fill(r, g, b, 100);
noStroke();
rect(buildingPos, (height-25)-buildingHeight, buildingWidth, buildingHeight);
buildingPos += buildingWidth;
}
}
Because it still looked little empty, I added the gradient to the background using the below code.
for (int i = 0; i < 960; i++) {
//println(i);
stroke(0, 0, 0, darkness);
line(i, 0, i, height);
darkness += 0.2;
}
drawBuilding();
As a result of the following efforts, I created something like this below.
Adding the building-like structure, and because the height and width of each building will be different every single time user opens the program, the user will be able to generate a unique looking album for himself/herself.
In addition, due to the mouseClicked() function, the user will be able to also draw the firework (after the basicBackground() function is moved to the setup() function.)
As you can see in the video above, the user can draw the firework by clicking on the points.
After all the work is done, this is the finalized image (with my firework on).
Because the user can be interactive with the drawing, I believe that drawing in Processing would be a good means of realizing my design.
Below is the source code for this drawing.
float darkness = 0;
// for clicking
boolean started = false; // for start selecting
boolean clicked = false; // checks if there is previously selected point
// for line selecting
int[] linePos = {0, 0, 0, 0};
//int[][] lineFinalPos = {linePos};
// for ratio
int[] verticalRatio = {291-247, 343-168};
int[] horizontalRatio = {149, 80};
int midPos = 0;
PShape s;
float shiftX = 50;
float shiftY = -100;
void setup(){
size(960, 600);
basicBackground();
frameRate(10);
//println(314+ (verticalRatio[0] * 0.75) , 335-(verticalRatio[1] * 0.75));
//println(horizontalRatio[0], horizontalRatio[1]);
//println(247+(horizontalRatio[0] * 0.9) , 343+ (horizontalRatio[1] * 0.9));
}
void draw(){
//lines
stroke(#F0D3AE);
strokeWeight(2);
// horizontal lines
line( 291 +shiftX, 168 +shiftY, 373 +shiftX, 168 +shiftY); //1
line( 373 +shiftX, 168 +shiftY, 492 +shiftX, 232 +shiftY); //2
line( 347 +shiftX, 204 +shiftY, 578 +shiftX, 204 +shiftY); //3
line( 578 +shiftX, 204 +shiftY, 534 +shiftX, 379 +shiftY); //4
// vertical lines
line( 291 +shiftX, 168 +shiftY, 247 +shiftX, 343 +shiftY); //5
line( 492 +shiftX, 232 +shiftY, 448 +shiftX, 407 +shiftY); //8
line( 247 +shiftX, 343 +shiftY, 381 +shiftX, 415 +shiftY); //6
line( 381 +shiftX, 415 +shiftY, 396 +shiftX, 379 +shiftY); //11
line( 448 +shiftX, 407 +shiftY, 314 +shiftX, 335 +shiftY); //9
line( 314 +shiftX, 335 +shiftY, 347 +shiftX, 204 +shiftY); //7
line( 534 +shiftX, 379 +shiftY, 396 +shiftX, 379 +shiftY); //10
if (clicked && started) {
stroke(255);
line(linePos[0], linePos[1], pmouseX, pmouseY);
} else {
line(linePos[0], linePos[1], linePos[2], linePos[3]);
}
noFill();
rect(25, 25, width-50, height-50);
}
void basicBackground(){
background(#5D1919);
for (int i = 0; i < 960; i++) {
//println(i);
stroke(0, 0, 0, darkness);
line(i, 0, i, height);
darkness += 0.2;
}
drawBuilding();
}
void drawBuilding(){
float buildingPos = 25;
float buildingWidth = 0;
float buildingHeight = 0;
while((buildingPos + buildingWidth) < (width – 25)){
buildingWidth = random(20, 50);
buildingHeight = random(90, 250);
float r = random(0, 150);
float g = random(0, 150);
float b = random(0, 150);
fill(r, g, b, 100);
noStroke();
rect(buildingPos, (height-25)-buildingHeight, buildingWidth, buildingHeight);
buildingPos += buildingWidth;
}
}
void mouseClicked(){
started = true;
if (! clicked){ // if there is no previously selected point
println(“clicked”);
linePos[0] = pmouseX;
linePos[1] = pmouseY;
clicked = true; // because we just selected one point, change the bool val.
} else {
println(“clicked2”);
linePos[2] = pmouseX;
linePos[3] = pmouseY;
clicked = false; // because we are done selecting two points, initialize
println(“line(“, linePos[0], “,”, linePos[1], “,”, linePos[2], “,”, linePos[3], “)”);
}
//println(pmouseX, pmouseY);
}
void keyPressed() {
if (key == CODED) {
if (keyCode == UP) {
shiftY -= 5;
} else if (keyCode == DOWN) {
shiftY += 5;
} else if (keyCode == LEFT) {
shiftX -= 5;
} else if (keyCode == RIGHT) {
shiftX += 5;
}
println(shiftX, shiftY);
}
}