The kittenBot was quite fun to work with; the instructions were very clear and the setup wasn’t too cumbersome or confusing. The only problem I ran into when I was putting the bot together was the placement of the screws, but that was a minor detail. The end product turned out fine:
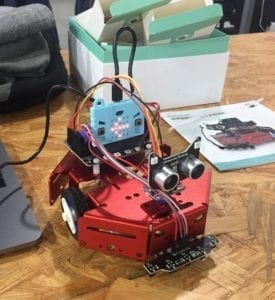
First off, I wanted to start off by playing around with the LED setup, just to see if I connected everything correctly. I displayed a few random icons initially, then utilized a simple code to switch between LED icons using the buttons:
if (input.buttonIsPressed(Button.A)) {
basic.showIcon(IconNames.Happy)
} else if (input.buttonIsPressed(Button.B)) {
basic.showIcon(IconNames.Sad)
}
LED Test:
Then, I programmed a simple motor script to test the motors and make the bot move within a constrained space. If button A is pressed, the bot will move clockwise in a continuous circle, and if button B is pressed, the bot will move counterclockwise.
if (input.buttonIsPressed(Button.A)) {
basic.showIcon(IconNames.Happy)
pins.servoWritePin(AnalogPin.P0, 0)
pins.servoWritePin(AnalogPin.P1, 180)
} else if (input.buttonIsPressed(Button.B)) {
basic.showIcon(IconNames.Sad)
pins.servoWritePin(AnalogPin.P0, 180)
pins.servoWritePin(AnalogPin.P1, 0)
}
Motor Test:
After testing the LEDs, DC motors, and Neopixels, I wanted to utilize the ultrasound sensor to make the bot detect nearby objects, and display reactions according to the distance of the object. The bot would move forward initially, but as it comes within a certain distance to an object, it will display an icon and turn 180 degrees and move forward. Of course, if it is stuck between two objects, it will forever move back and forth between them:
let distance = 0
distance = robotbit.Ultrasonic(DigitalPin.P3)
if (distance > 50) {
pins.servoWritePin(AnalogPin.P0, 0)
pins.servoWritePin(AnalogPin.P1, 0)
basic.showIcon(IconNames.Happy)
} else if (distance < 50) {
basic.showIcon(IconNames.Sad)
for (let i = 0; i < 1; i++) {
pins.servoWritePin(AnalogPin.P0, 180)
pins.servoWritePin(AnalogPin.P1, 0)
}
pins.servoWritePin(AnalogPin.P0, 0)
pins.servoWritePin(AnalogPin.P1, 0)
}
if (input.buttonIsPressed(Button.A)) {
basic.showIcon(IconNames.Yes)
pins.servoWritePin(AnalogPin.P0, 0)
pins.servoWritePin(AnalogPin.P1, 180)
} else if (input.buttonIsPressed(Button.B)) {
basic.showIcon(IconNames.No)
pins.servoWritePin(AnalogPin.P0, 180)
pins.servoWritePin(AnalogPin.P1, 0)
}
Ultimately, the kittenBot was a fun and simple lab; I really liked how all the pieces came together and I thought it was really well designed. I hope to utilize more sensors in the future with the microbit.