During this week’s recitation we were required to control an image or video by manipulating that media’s attributes using a physical controller made with Arduino. I decided to choose my image from Creativity103.com which was given to use in the resource file. I chose a “Christmas Light” image and decided I would use the Filter example, specifically using the “blur” function. On my Arduino board, I used a Potentiometer to manipulate the “blurriness” of the picture I had chosen.
I began by opening the provided Serial Communication example code given to us. I used the Serial One Value codes as I was only sending a single value. I then opened up the “filter blur” example and typed in the code onto the ‘one value from AtoP processing’ code. I then went onto the Arduino Serial One Value code and included the map function to transmit my Potentiometer values from Arduino to Processing. To do this, I included an int value: int picture = map(sensorValue, 0, 1023, 0, 300). I then made sure I included ‘picture’ in the Serial.write code. On my Processing code, I had to include PImage and under void setup() I loaded the photo I had used which I named “colors.jpg.”. I wanted to control the range of blurriness depending on which values my Potentiometer was projecting so I included an If statement under void draw() which stated that if(valueFromArduino > 150) then it would filter(BLUR, 2); but if(valueFromArduino <100 then it would filter(BLUR, 5). Find below video.
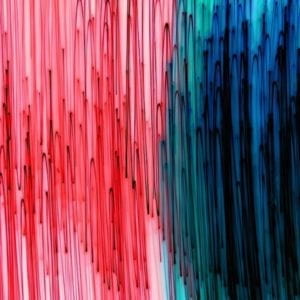
Reading
The article Computer Vision for Artists and Designers draws light to the importance of computer vision through vision-based detection and tracking techniques to show how computer vision can be used in society to benefit its people. Most people assume “computer vision” has no greater aim or purpose and cannot really do anything that will contribute to the betterment of individuals in society, however this article proves this assumption wrong. One of the examples provided that I found was a really smart way to incorporate technology was Rafael LozanoHemmer’s installation: Standards and Double Standards (2004). This installation consisted of fifty leather belts which were suspended at waist height with the help of servo-motors located on the ceiling. Through computer vision tracking system, the belts would turn towards an individual depending on their movement. This project metaphorically illustrates the power of the patriarchy in an artistic manner. This had me thinking about how we could use Arduino and Processing to distort/illustrate images to reveal a deeper meaning and convey a deeper message to society. Art through computer vision is an interactive and engaging way to capture the attention of the public and allow them to see the pressing issues faced within society that need to be addressed.
Resources: