The first exercise was to make a Processing Etch A Scetch. The circuit was easy to build, only using two potentiometers. We first drew an ellipse in Processing and used the values from the potentiometers to control the “x” and “y” of the ellipse. Since we needed two values from Arduino, I used the sample code for multiple values and modified it. But when I tried to draw the line, all I got were dots. Luckily I later fixed it with the others’ help. I think the interaction is that people can use the potentiometers to change the values of “x” and “y” , and the line in processing is drawn accordingly.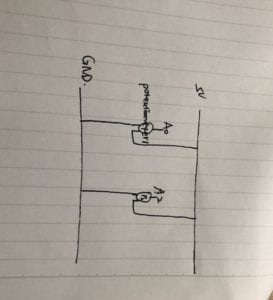
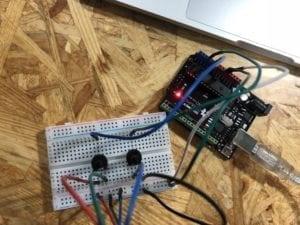
code for Processing
// IMA NYU Shanghai
// Interaction Lab
// For receiving multiple values from Arduino to Processing
/*
* Based on the readStringUntil() example by Tom Igoe
* https://processing.org/reference/libraries/serial/Serial_readStringUntil_.html
*/
import processing.serial.*;
float pX;
float pY;
String myString = null;
Serial myPort;
int NUM_OF_VALUES = 2; /** YOU MUST CHANGE THIS ACCORDING TO YOUR PROJECT **/
int[] sensorValues; /** this array stores values from Arduino **/
void setup() {
size(800, 800);
setupSerial();
background(0);
}
void draw() {
updateSerial();
float x = map (sensorValues[0],0,1023,0,width);
float y = map (sensorValues[1],0,1023,0,height);
strokeWeight(3);
stroke(255);
line(pX,pY,x,y);
pX=x;
pY=y;
// use the values like this!
// sensorValues[0]
// add your code
//
}
void setupSerial() {
printArray(Serial.list());
myPort = new Serial(this, Serial.list()[ 9 ], 9600);
// WARNING!
// You will definitely get an error here.
// Change the PORT_INDEX to 0 and try running it again.
// And then, check the list of the ports,
// find the port "/dev/cu.usbmodem----" or "/dev/tty.usbmodem----"
// and replace PORT_INDEX above with the index number of the port.
myPort.clear();
// Throw out the first reading,
// in case we started reading in the middle of a string from the sender.
myString = myPort.readStringUntil( 10 ); // 10 = '\n' Linefeed in ASCII
myString = null;
sensorValues = new int[NUM_OF_VALUES];
}
void updateSerial() {
while (myPort.available() > 0) {
myString = myPort.readStringUntil( 10 ); // 10 = '\n' Linefeed in ASCII
if (myString != null) {
String[] serialInArray = split(trim(myString), ",");
if (serialInArray.length == NUM_OF_VALUES) {
for (int i=0; i<serialInArray.length; i++) {
sensorValues[i] = int(serialInArray[i]);
}
}
}
}
}
code for Arduino
// IMA NYU Shanghai
// Interaction Lab
// For sending multiple values from Arduino to Processing
void setup() {
Serial.begin(9600);
}
void loop() {
int sensor1 = analogRead(A0);
int sensor2 = analogRead(A2);
//int mappedsensor1 = map(sensor1,0,1023,0,255);
//int mappedsensor2 = map(sensor2,0,1023,0,255);
// keep this format
Serial.print(sensor1);
Serial.print(“,”); // put comma between sensor values
Serial.print(sensor2);
Serial.println(); // add linefeed after sending the last sensor value
// too fast communication might cause some latency in Processing
// this delay resolves the issue.
delay(100);
}
The second exercise was to make a music instrument with Arduino. For this one, I used the sample code for multiple values from P to A. I used the values of mouseX and mouseY to control the frequency and duration in Arduino. The interaction is that people can move the mouse around to change the pitch and length of the melody. As the mouse moves from left to right, the pitch changes from low to high accordingly. Moving the mouse up and down will change the length of the melody.
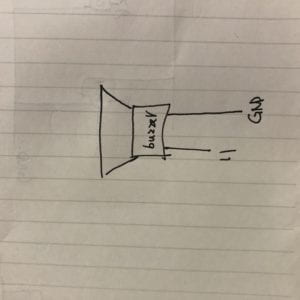
code for Processing
// IMA NYU Shanghai
// Interaction Lab
/**
* This example is to send multiple values from Processing to Arduino.
* You can find the arduino example file in the same folder which works with this Processing file.
* Please note that the echoSerialData function asks Arduino to send the data saved in the values array
* to check if it is receiving the correct bytes.
**/
import processing.serial.*;
int NUM_OF_VALUES = 2; /** YOU MUST CHANGE THIS ACCORDING TO YOUR PROJECT **/
Serial myPort;
String myString;
// This is the array of values you might want to send to Arduino.
int values[] = new int[NUM_OF_VALUES];
void setup() {
size(500, 500);
background(0);
printArray(Serial.list());
myPort = new Serial(this, Serial.list()[ 9], 9600);
// check the list of the ports,
// find the port "/dev/cu.usbmodem----" or "/dev/tty.usbmodem----"
// and replace PORT_INDEX above with the index of the port
myPort.clear();
// Throw out the first reading,
// in case we started reading in the middle of a string from the sender.
myString = myPort.readStringUntil( 10 ); // 10 = '\n' Linefeed in ASCII
myString = null;
}
void draw() {
background(0);
values[0]=mouseX;
values[1]=mouseY;
// changes the values
// sends the values to Arduino.
sendSerialData();
// This causess the communication to become slow and unstable.
// You might want to comment this out when everything is ready.
// The parameter 200 is the frequency of echoing.
// The higher this number, the slower the program will be
// but the higher this number, the more stable it will be.
echoSerialData(200);
}
void sendSerialData() {
String data = "";
for (int i=0; i<values.length; i++) {
data += values[i];
//if i is less than the index number of the last element in the values array
if (i < values.length-1) {
data += ","; // add splitter character "," between each values element
}
//if it is the last element in the values array
else {
data += "n"; // add the end of data character "n"
}
}
//write to Arduino
myPort.write(data);
}
void echoSerialData(int frequency) {
//write character 'e' at the given frequency
//to request Arduino to send back the values array
if (frameCount % frequency == 0) myPort.write('e');
String incomingBytes = "";
while (myPort.available() > 0) {
//add on all the characters received from the Arduino to the incomingBytes string
incomingBytes += char(myPort.read());
}
//print what Arduino sent back to Processing
print( incomingBytes );
}
code for Arduino
// IMA NYU Shanghai
// Interaction Lab
/**
This example is to send multiple values from Processing to Arduino.
You can find the Processing example file in the same folder which works with this Arduino file.
Please note that the echo case (when char c is ‘e’ in the getSerialData function below)
checks if Arduino is receiving the correct bytes from the Processing sketch
by sending the values array back to the Processing sketch.
**/
#define NUM_OF_VALUES 2 /** YOU MUST CHANGE THIS ACCORDING TO YOUR PROJECT **/
/** DO NOT REMOVE THESE **/
int tempValue = 0;
int valueIndex = 0;
/* This is the array of values storing the data from Processing. */
int values[NUM_OF_VALUES];
void setup() {
Serial.begin(9600);
pinMode(11, OUTPUT);
}
void loop() {
getSerialData();
tone(11, values[0],values[1]*10);
// add your code here
// use elements in the values array
// values[0]
// values[1]
}
//recieve serial data from Processing
void getSerialData() {
if (Serial.available()) {
char c = Serial.read();
//switch – case checks the value of the variable in the switch function
//in this case, the char c, then runs one of the cases that fit the value of the variable
//for more information, visit the reference page: https://www.arduino.cc/en/Reference/SwitchCase
switch (c) {
//if the char c from Processing is a number between 0 and 9
case ‘0’…’9′:
//save the value of char c to tempValue
//but simultaneously rearrange the existing values saved in tempValue
//for the digits received through char c to remain coherent
//if this does not make sense and would like to know more, send an email to me!
tempValue = tempValue * 10 + c – ‘0’;
break;
//if the char c from Processing is a comma
//indicating that the following values of char c is for the next element in the values array
case ‘,’:
values[valueIndex] = tempValue;
//reset tempValue value
tempValue = 0;
//increment valuesIndex by 1
valueIndex++;
break;
//if the char c from Processing is character ‘n’
//which signals that it is the end of data
case ‘n’:
//save the tempValue
//this will b the last element in the values array
values[valueIndex] = tempValue;
//reset tempValue and valueIndex values
//to clear out the values array for the next round of readings from Processing
tempValue = 0;
valueIndex = 0;
break;
//if the char c from Processing is character ‘e’
//it is signalling for the Arduino to send Processing the elements saved in the values array
//this case is triggered and processed by the echoSerialData function in the Processing sketch
case ‘e’: // to echo
for (int i = 0; i < NUM_OF_VALUES; i++) {
Serial.print(values[i]);
if (i < NUM_OF_VALUES – 1) {
Serial.print(‘,’);
}
else {
Serial.println();
}
}
break;
}
}
}