Interactive Projects
Interaction is reciprocated influence. In both the Group Project and the Midterm project, I thought of it as a conversation- an exchange of data with an end goal. The “Heal-o-matic 5000” assessed its patient and listened to their concerns so as to provide optimal care. “Ask Alice” is an experience disguised as a simple slot machine: It betrays the user’s expectations in order to convey a message.
The Concept
“Ask Alice” is played like any regular slot machine. The player rolls the slots and hopes to achieve a certain pattern (in our machine, the goal was to roll three white rabbits). When the player wins, a white rabbit candy is dispensed from the machine, as the prize. Except it’s not a candy- it’s a fact about the effect of gambling in a community. Users unwrap it expecting a sweet, but instead they are met with a small roll of paper with a quote or word like “domestic abuse” (which tends to increase or worsen when there is a gambling addiction). We thus play with the user’s expectations to create an experience, in which the user feels like they’ve gained insight. This is what makes our project interactive- not necessarily the machine itself.
The Statement
The purpose of this product is to make our users think about the negative impact of addictions in society. We chose gambling instead of alcoholism of drug abuse because not many people of our generation and socio-economic background seem to think about it’s impact, as they haven’t personally been affected by it, and it’s negative effects aren’t always as obvious as those of other addictions. It’s certainly not in the media the way alcohol and drugs are- no one raps about their gambling issues. And yet it also has harmful byproducts, like increased rates of domestic abuse, bankruptcy and suicide. We wanted our project to be educational- but more than that, we wanted it to make people think about serious problems they couldn’t relate to, and don’t give much thought to.
The Original Design
Originally, instead of slots decorated to resemble those in traditional slot machines, we were going to use three 8-segmented LEDS. So instead of trying to roll three white rabbits, the player would have rolled for three sevens. We decided against this, because of the feedback from the user test. Users told us that maybe the message would come across most powerfully when the slot machine looked like one you could find in a real Casino. Below is some the wiring we did for the 8-segmented LEDs.
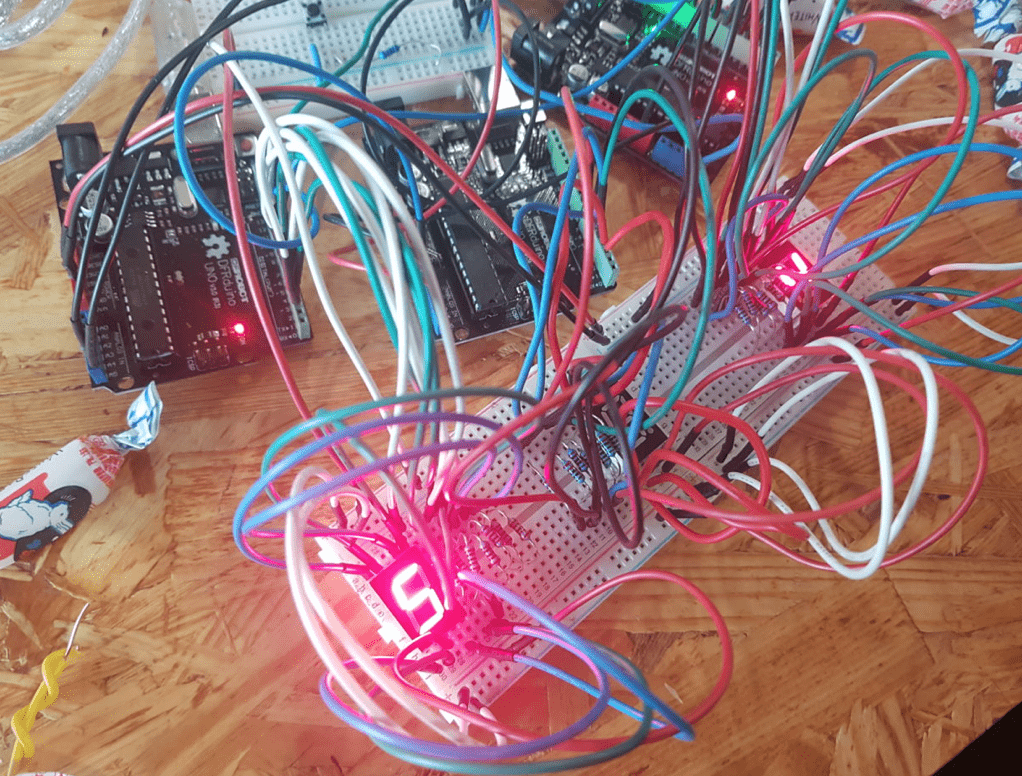
We ultimately changed our design because of the results of the user test, and because the classic icons you see on slot machines are more aesthetically pleasing. In our project, aesthetics are important as they help get the message across.
The Final Design
The physical product and it’s aesthetics are a key part of the experience. The machine had to be flashy yet unassuming. Luring, but not so much so that it was an obvious trap. We wanted to make the experience simulate the real-deal up to the moment they unwrapped their prize, to increase the user’s surprise. Bellow is a picture we found online that we used as inspiration for our design, as well as some of our sketches from the ideation phase:
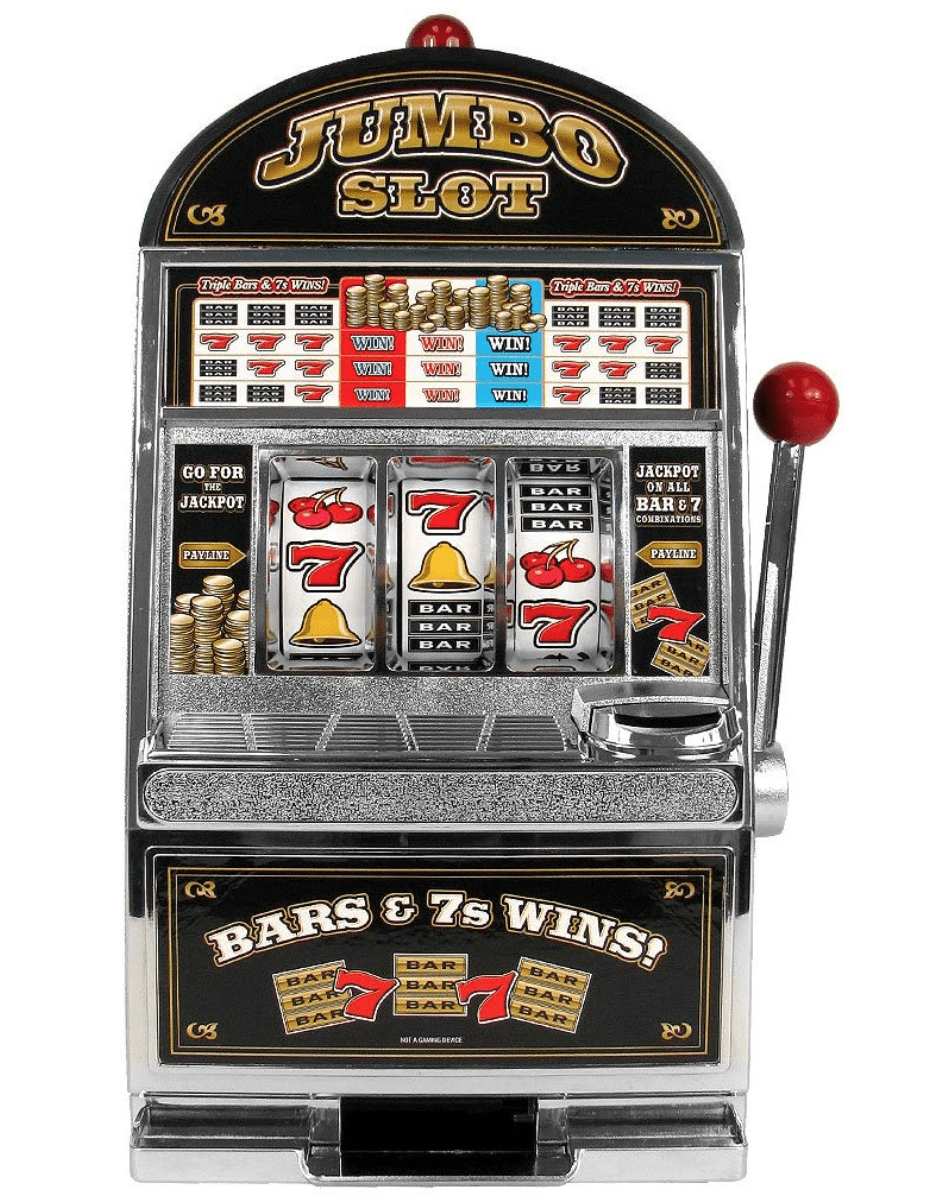
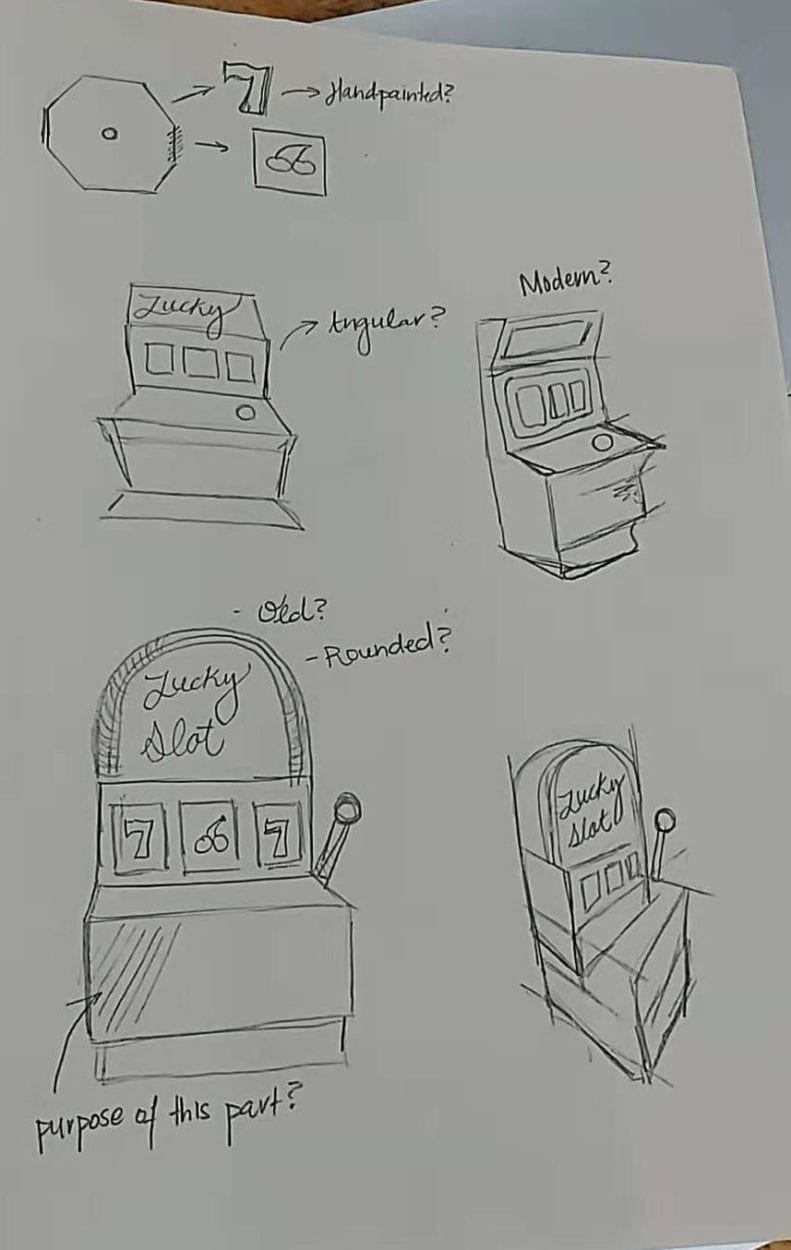
We also wanted to hide some messages that someone interested in looking a little closer at our project could see. People love easter eggs. They like feeling that they’ve noticed something that maybe the person before them did not. It makes them feel smart. We learned this through the user test, when users told us they liked unwrapping the information. What it initially told us was that having the prize be a candy you unwrap as opposed to a token was a good idea for our intended market, but when we explored the concept, we realized it also applied to any information we could give the users. The potential for discovery intrigues people. So we decided to leave hidden meanings in the presentation of the machine too.
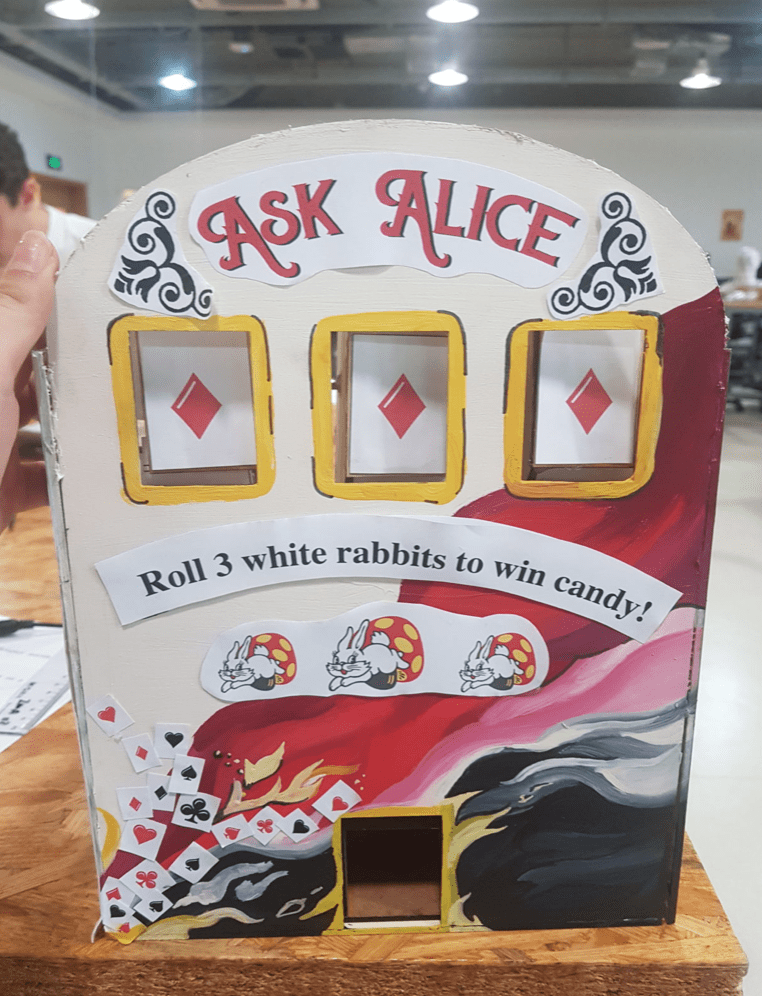
The Presentation- Hidden Meanings
The name of the slot machine “Ask Alice” refers to the book “Alice’s Adventures in Wonderland” by Lewis Carrol. That Alice blindly and foolishly follows the rabbit down a rabbit hole. To fall “down the rabbit hole” is also an English idiom for getting yourself in a chaotic unwanted situation and is sometimes used to describe when someone falls into an addiction. This is a recurring theme in the decoration of our slot machine. For example, on the side of the machine it says “Don’t fall down the rabbit hole!” but in small black letters that camouflage with the background it says “fall” repeatedly. This represents how the gambling industry promises a way to get rich quick, but they are profiting from your losses.
Construction
We had to design three main physical parts: The outer casing, the rotating slots, and the prize dispenser. All were designed on Adobe illustrator. Bellow is a picture that shows the outer casing and the prize dispensing mechanism before we inserted the electrical components. The rotating slots were done by pasting one icon on each side of 3 octagons, which would each be connected to a stepper motor, and thus rotate.
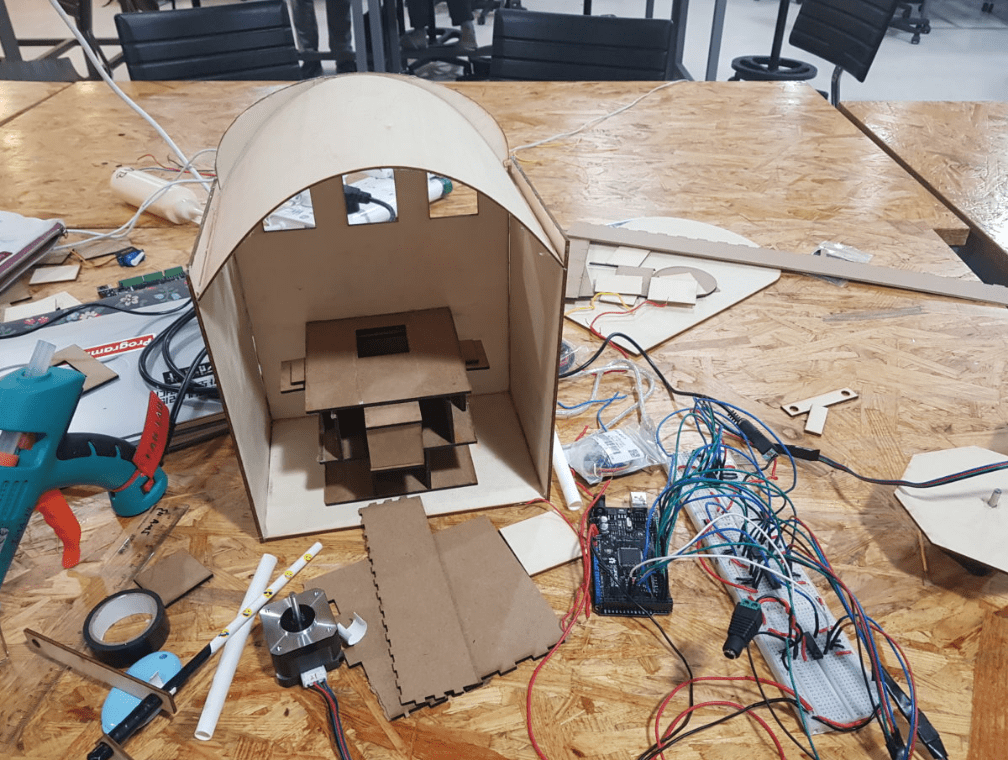
We used Youtube tutorial to learn how to make the candy dispenser. It was very helpful to see how other people had done it before. The one we used in our project is almost exactly like the one in the video linked above, except for the fact that we changed its dimensions, and had to add an extended arm that would allow the stepper motor to control when the candy was dispensed (as opposed to how in the video, candy is dispensed simply with the pressing of a button). Below is our design for the extension, and a video showing how it was supposed to work, once we connected the stepper motor.
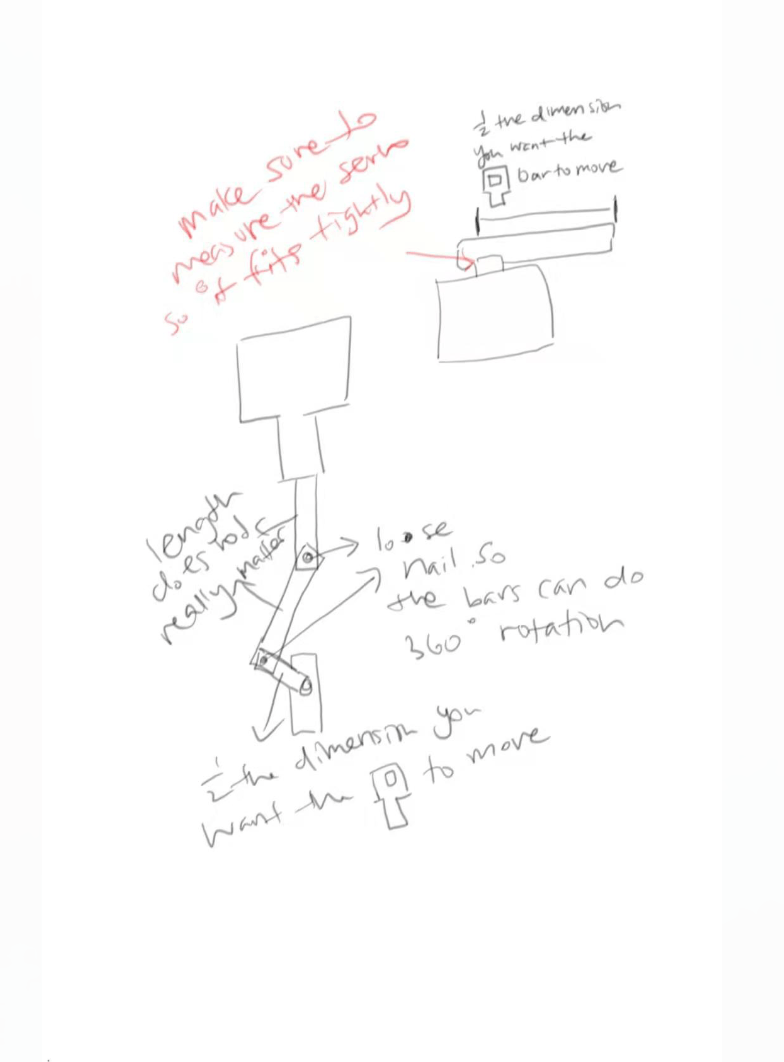
Code
Below is the code we used to:
- Have 3 stepper motors rotate the slots and eventually land on the white rabbits
- Once the slots all showed the white rabbits, the stepper motor that was attached to our candy dispensing mechanism would rotate to allow candy to fall.
#include <Stepper.h>
#include <Servo.h>
// change this to the number of steps on your motor
#define stepsPerRev 200
// create an instance of the stepper class, specifying
// the number of steps of the motor and the pins it’s
// attached to
Stepper hundredthWheel(stepsPerRev, 26, 2, 3, 4);
Stepper tenthWheel(stepsPerRev, 24, 5, 6, 7);
Stepper onethWheel(stepsPerRev, 22, 8, 9, 10);
Stepper handle(stepsPerRev, 40, 41, 42, 43);
//———————————————————————————
// VARIABLE
//———————————————————————————
// Number Variables
// Lucky Number
int luckyNum = 777; // If the user hit this number,
// = jackpot.
// they will receive a candy from the machine
// Slot content variable
int userNumSize = 3;
int userNum[3] = {0, 0, 0}; // initialized digits
int finalNum = 0;
// Degree variables
int numSteps[3] = {0, 0, 0};
// how many times you want to rotate the wheel before it stops
int prevRotate = 4;
//———————————————————————————
// Button / levar variables
// Boolean variables (for button / levar)
bool pressed = false; // the button
bool curPressed = 0; // a variable to limit an event to happen only once.
// Pins
int buttonPin = 30;
//———————————————————————————
// Chance variables
// For how many times the user has FAILED so far
int failedNum = 0;
// At least once (?) time we want the user to win
int enoughFails = 3;
//———————————————————————————
// Chance variables
// the previous reading from the analog input
int hundredthPrevious = 0;
int tenthPrevious = 0;
int onethPrevious = 0;
//———————————————————————————
// FUNCTIONS
//———————————————————————————
void setup() {
Serial.begin(9600);
// button pin
pinMode(buttonPin, INPUT);
// randomSeed() will then shuffle the random function.
randomSeed(analogRead(0));
// set the speed of the motor to 100 RPMs
hundredthWheel.setSpeed(70);
tenthWheel.setSpeed(70);
onethWheel.setSpeed(70);
handle.setSpeed(100);
Serial.println(“Ready to go!”);
}
//———————————————————————————
void loop() {
buttonCheck(); //the loop-function will be calculating whether the button is pressed
delay(10); // for consistency and safety, we are keeping the delay in between
}
void buttonCheck() {
// simply checks whether the button is pressed or not
curPressed = digitalRead(buttonPin); // 0 = button is not pressed; 1 = button is not currently pressed
if (not pressed && curPressed) { // if the button is pressed run the code below.
Serial.println(“————“);
calculateDigit();
turnSteps();
luckyMoment();
if(finalNum == 777){ // if the user hits the lucky number,
// using the stepper to pull on the bar to take out the candy
luckyMoment();
}
pressed = true;
} else if (pressed && not curPressed) { // filters out when the button WAS pressed,
// but the button is not pressed right now
pressed = false;
}
}
void calculateDigit() {
// this function calculates the number for the digits
finalNum = 0; // initialize the number
// if (failedNum == enoughFails){
// Serial.println(“LUCKY!”);
for (int digit = 0; digit < userNumSize; digit++) {
userNum[digit] = 7;
// the actual calcuating part
finalNum += userNum[digit] * pow(10, (2 – digit));
}
//
// failedNum = 0; // initialize the the failedNum
//
// } else {
// for (int digit = 0; digit < userNumSize; digit++) {
// userNum[digit] = random(1, 7);
//
// // the actual calcuating part
// finalNum += userNum[digit] * pow(10, (2 – digit));
// }
// finalNum += 1;
//
if (finalNum == 777){ // if the user hits 777, initialize the failed Num
failedNum = 0; // , initialize the failed Num
} else { // if the user fails to hit 777,
failedNum++; // increment failedNum
}
//
// }
Serial.println(finalNum);
}
void calculateSteps() {
for (int wheel = 0; wheel < userNumSize; wheel++) {
int newVal = map(userNum[wheel], 0, 7, 0, 200);
int steps = (200 / 8) * userNum[wheel];
numSteps[wheel] = steps;
Serial.println(userNum[wheel]);
}
}
void turnSteps() {
calculateSteps();
// move the stepper back to its original position
delay(10);
hundredthWheel.step(hundredthPrevious);
delay(10);
tenthWheel.step(-tenthPrevious);
delay(10);
onethWheel.step(-onethPrevious);
delay(10);
delay(1000);
Serial.println(“starting”);
// Turn the hundredth wheel
for (int i = 0; i < prevRotate; i++) { // First give the anxiety by turning the wheels several times
hundredthWheel.step(-200);
delay(1);
}
hundredthWheel.step(-numSteps[0]); // Turn to the right value
// Turn the tenth wheel
for (int i = 0; i < prevRotate; i++) { // First give the anxiety by turning the wheels several times
tenthWheel.step(200);
delay(1);
}
tenthWheel.step(numSteps[1]); // Turn to the right value
// Turn the oneth wheel
for (int i = 0; i < prevRotate; i++) { // First give the anxiety by turning the wheels several times
onethWheel.step(200);
delay(1);
}
onethWheel.step(numSteps[2]); // Turn to the right value
// renew the previous values
hundredthPrevious = numSteps[0];
tenthPrevious = numSteps[1];
onethPrevious = numSteps[2];
}
void luckyMoment() {
//this controls the stepper to pull on the handle
handle.step(100); // pull out the handle by rotating the stepper
delay(1000); // wait for the handle stepper to finish pulling the bar
handle.step(100); // push it back in
}
Difficulties and aspects to improve
We had a few difficulties in creating this project. Most of them stemmed from our proportions being off- We didn’t design all the parts to be of the correct dimensions, which often led to unwanted friction between the mechanical parts. This friction resulted in malfunctions like the one in the video below.
If we were to repeat this project, we would ensure the parts are proportionate so that the spinning of the slots is more accurate and to lessen the likelihood that the candy dispenser gets stuck. We would also make the slots round and lighter- instead of an octagon with laser-cut rectangles pasted on, we would simple use a reel of icons. This would lessen the chance of the slots hitting a part of the machine and getting stuck. We would also prototype more before laser cutting a final version. Next time, we will first laser-cut the design in cardboard to ensure it is fitting.
Overall, we were happy with the class’ reaction to our message.