In this recitation, we focused on creating functions and arrays.
Step 1:
I created an upside-down Android shaped object in this step with a semicircle on the bottom, a square for the body and two rectangles for the legs. So it is like an Android thing falling.
The code for the buggy (with x and y values because I was testing out how it looked) is:
void buggy() {
arc(200,200,75,75,0,PI,CHORD);
rect(162,125,75,70);
rect(167,70,20,50);
rect(212,70,20,50);
And it looks like:
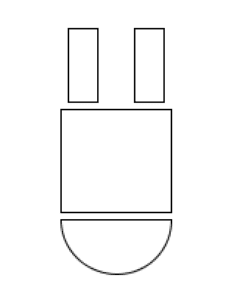
Step 2:
To allow it to fit in the for loop by having paramaters, I changed all the x and y values for the void buggy, added a strokeWeight(1); and stroke(c); and added the paramaters to the void parentheses so the code looked like:
void buggy(float x, float y, color c) {
strokeWeight(1);
stroke(c);
arc(x,y,75,75,0,PI,CHORD);
rect(x-38,y-75,75,70);
rect(x-33,x-130,20,50);
rect(x+12,x-130,20,50);
}
With that, I created the for loop which looked like:
for (int i=0;i<100;i++){
buggy(random(width),random(height),color(random(255),random(255),random(255)));
}}
When I put it inside void setup, it did not work and the screen was completely white with no figures on it.
And I copied and pasted it onto void draw and my figure kept flashing across the screen in different directions. And this was fixed by adding noLoop(); to the end of void draw.
At this point it looked like this:
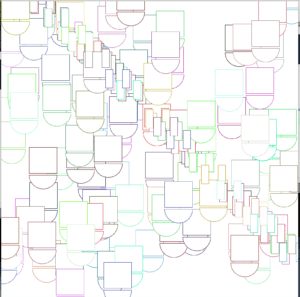
Step 3:
For this step, we had to create three arrays, store their data in setup, and display them in void draw.
I started off by creating the three universal arrays:
float[] xVal = new float[100];
float[] yVal = new float[100];
float[] cVal = new float[255];
This means that there will be a total of 100 random x and y values created for the x and y value arrays, and 255 random colors.
To store the values in setup I create two for loops (one for the x and y values and one for the color), I wrote the code:
for(int i=0; i<xVal.length; i++) {
xVal[i] = random(width);
yVal[i] = random(height);
}
for(int i=0; i<cVal.length; i++) {
cVal[i] = color(random(0,255),random(0,255),random(0,255));
}
This stores the values for x and y to have a random width and height 100 times (which is the xVal.length). xVal.length and yVal.length are pretty much the same thing (because they have 100 values).
For the last part of this step, I changed the for loop I had earlier for the buggy by switching 100 to xVal.length and inserted the stored parameters into the buggy so the void draw looks like:
void draw(){
background(255);
for (int i=0;i<100;i++){
buggy(xVal[i],yVal[i],cVal[i]);
}
noLoop();
}
This was when I came into my first error that showed:
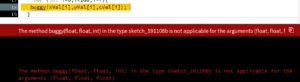
After asking a learning assistant, I found out that at the top,
float[] cVal = new float[255]; is wrong.
It should be color[] cVal = new color[255]; instead.
And everything seems fine until I looked at the final product again and..
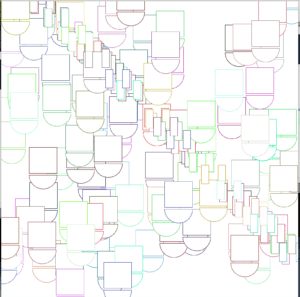
The legs are not connected with the body…., they are scattered in a diagonal line from the top left of the screen to the bottom right.
And I found out the code for the buggy was:
void buggy(float x, float y, color c) {
strokeWeight(1);
stroke(c);
arc(x,y,75,75,0,PI,CHORD);
rect(x-38,y-75,75,70);
rect(x-33,x-130,20,50);
rect(x+12,x-130,20,50);
}
And…. for the last two rect();s, I put in x-130 instead of y-130.
After changing that, everything was back to normal as shown here:
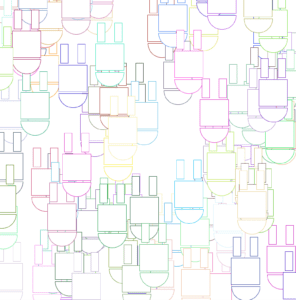
As of now the code looks like (before I change it more):
float[] xVal = new float[100];
float[] yVal = new float[100];
color[] cVal = new color[255];
void setup(){
size(800,800);
for(int i=0; i<xVal.length; i++) {
xVal[i] = random(width);
yVal[i] = random(height);
}
for(int i=0; i<cVal.length; i++) {
cVal[i] = color(random(0,255),random(0,255),random(0,255));
}
}
void draw(){
background(255);
for (int i=0;i<100;i++){
buggy(xVal[i],yVal[i],cVal[i]);
}
noLoop();
}
void buggy(float x, float y, color c) {
strokeWeight(1);
stroke(c);
arc(x,y,75,75,0,PI,CHORD);
rect(x-38,y-75,75,70);
rect(x-33,y-130,20,50);
rect(x+12,y-130,20,50);
}
Step 4:
To animate it and create individual movement, I wanted to move the buggys from left to right at a small amount (between -1 and 1 x and y). So inside the for loop, I changed the code to:
buggy(xVal[i]+random(-1,1),yVal[i]+random(-1,1),cVal[i]);
Therefore having the x and y value increase or decrease by any number between -1 and 1.
Also for this to happen I would have to have it move around, so I took out noLoop();
Next, I created an integer of i to equal 0, to match all the i’s in the buggy an elsewhere. So it would be int i = 0;
After that, I created an if command (I had to make sure it was outside the for loop and not inside by asking a friend). And the if command incorporated the integer i = 0, and it said that if i was 0 or greater the buggy would move around. I tried putting int i == 0, but it didn’t work so only i >= 0 worked.
So after everything, the whole code looked like:
float[] xVal = new float[100];
float[] yVal = new float[100];
color[] cVal = new color[255];
int i = 0;
void setup(){
size(800,800);
for(int i=0; i<xVal.length; i++) {
xVal[i] = random(width);
yVal[i] = random(height);
}
for(int i=0; i<cVal.length; i++) {
cVal[i] = color(random(0,255),random(0,255),random(0,255));
}
}
void draw(){
background(255);
if(i>=0){
for (int i=0;i<100;i++){
buggy(xVal[i]+random(-1,1),yVal[i]+random(-1,1),cVal[i]);
}
}
//noLoop();
}
void buggy(float x, float y, color c) {
strokeWeight(1);
stroke(c);
arc(x,y,75,75,0,PI,CHORD);
rect(x-38,y-75,75,70);
rect(x-33,y-130,20,50);
rect(x+12,y-130,20,50);
}
And here is a video of it:
Question 1:
So the for loop worked in void draw instead of void setup. I think this is because void setup only runs the code from top to bottom once, while void draw continuously runs it from top to bottom. Therefore void draw fits the for loop better because the for loop needs to keep drawing until a certain number, which is described after the first semicolon in the for loop function. For example in this function it was i<50, so the for loop had to run 50 times in total, and void setup cannot do that, only void draw can.
Question 2:
Arrays can store values that you can bring up later in coding. I think the benefit is that you can store all the values you think you need earlier on in the code so you don’t have to bring it up one by one later on using int = x. Another benefit could be to store a random array of numbers and use it later on in your code in a random number provider, being that those numbers are specific to the range you want.