Nov 1
I developed interaction based on my last project from Recitation 5. Since there are two versions of the last project, two versions of interaction are also imagined and developed here. The difference is realized by merely changing the filling color and limiting the area in “while” function.
MilkyWayNormal Part 1
MilkyWayNormal Part 2: It’s just too long and I had to trim the video and divide it into two parts. So it’s the same animation here.
MilkyWayNormal Interaction Two : Yellow arc appears with the same radius as the white arc. The mousePressed function is put in the void draw() rather than separated as void mousePressed, which helps realize another kind of interaction. Long press mouse and the spectator will see a different picture.
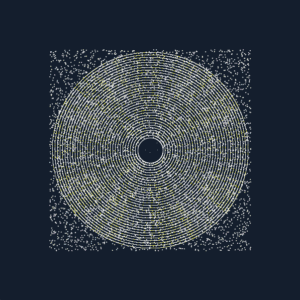
One Frame Screenshot
MilkyWayNormal Interaction Two: Arc in background color creates another effect.
MilkyWayIntense Part 1: Normal animation and interaction – the spectator presses the mouse to see random yellow/ red arcs appearing and covering the original white circle consisted of tiny arcs.
MilkyWayIntense Part 2: Since “mousePressed” is void, so one press only enacts one group of random arcs to appear. As long as the spectator is not intentionally pressing the mouse, we will see the initial animation of white arcs (enabled by void draw()) start to “engulf” the yellow and red arcs.
MilkyWayNormal Code
void setup(){
size(600,600);
background(20,30,45);
int r = 10;
while (r < 400) {
float rad1=random(TWO_PI);
float radspeed=rad1+0.001;
float start= rad1;
float stop= radspeed;
noFill();
strokeWeight(1);
stroke(255);
arc(300,300, r, r, start, stop);
radspeed=radspeed+0.0001;;
r = r + 10;
}
}
void draw(){
fill(20,30,45);
circle(300,300,50);
float red=random(200,255);
float green=random(200,255);
float blue=random(200,255);
float x= random(100,500);
float y= random(100,500);
strokeWeight(1);
stroke(red,green,blue);
ellipse(x,y,1,1);
int r = 10;
while (r < 400) {
float rad1=random(TWO_PI);
float radspeed=rad1+0.001;
float start= rad1;
float stop= radspeed;
noFill();
strokeWeight(1);
stroke(255);
arc(300,300, r, r, start, stop);
radspeed=radspeed+0.0001;;
r = r + 10;
POSSIBILITY TWO – YELLOW WITH CONTINUOUS INTERACTION IF MOUSE LONG PRESSED; ARC IN THE SAME RADIUS
// if (mousePressed){
// //int r=10;
// while (r < 400) {
// //float rad1=random(TWO_PI);
// radspeed=rad1+0.1;
// noFill();
// strokeWeight(1);
// stroke(243,238,0);
// arc(300,300, r, r, start, stop);
// radspeed=radspeed+0.0001;;
// r = r + 10;
//}
//}
}
//POSSIBILITY ONE – YELLOW AND RED WITH RANDOM SPEED
//INTERACT ONLY WHEN MOUSE PRESSED
void mousePressed(){
if (mousePressed){
int r=10;
while (r<200) {
float rad1=random(TWO_PI);
float radspeed=rad1+1;
float start= rad1;
float stop= radspeed;
noFill();
strokeWeight(1);
stroke(255,0,0);
arc(300,300, r, r, start, stop);
radspeed=radspeed+0.0001;;
r = r + 10;
}
while ( r < 400) {
float rad1=random(TWO_PI);
float radspeed=rad1+1;
float start= rad1;
float stop= radspeed;
noFill();
strokeWeight(1);
stroke(245,238,0);
arc(300,300, r, r, start, stop);
radspeed=radspeed+0.0001;;
r = r + 10;
}
}
}
Caution: Due to the logic of language in Processing, I need to put this part of code
“while (r<200) {
…
}”
before
“while (r<400) {
…
}”
to let processing recognize what I want it to happen. If the order is reversed, then processing won’t be able to read the narrower restrictment, so the differentiated color effect won’t show neither.
MikyWayIntense Animation + Interaction Code
void setup(){
size(600,600);
background(20,30,45);
int r = 10;
while (r < 400) {
float rad1=random(TWO_PI);
float radspeed=rad1+0.001;
float start= rad1;
float stop= radspeed;
noFill();
strokeWeight(1);
stroke(255);
arc(300,300, r, r, start, stop);
radspeed=radspeed+0.0001;;
r = r + 10;
}
}
void draw(){
//fill(20,30,45);
//circle(300,300,50);
float red=random(200,255);
float green=random(200,255);
float blue=random(200,255);
float x= random(100,500);
float y= random(100,500);
strokeWeight(1);
stroke(red,green,blue);
ellipse(x,y,1,1);
int r = 1;
while (r < 400) {
float rad1=random(TWO_PI);
float radspeed=rad1+0.001;
float start= rad1;
float stop= radspeed;
noFill();
strokeWeight(1);
stroke(255);
arc(300,300, r, r, start, stop);
radspeed=radspeed+0.0001;;
r = r + 1;
void mousePressed(){
if (mousePressed){
int r=10;
while (r<200) {
float rad1=random(TWO_PI);
float radspeed=rad1+1;
float start= rad1;
float stop= radspeed;
noFill();
strokeWeight(1);
stroke(255,0,0);
arc(300,300, r, r, start, stop);
radspeed=radspeed+0.0001;;
r = r + 10;
}
while ( r < 400) {
float rad1=random(TWO_PI);
float radspeed=rad1+1;
float start= rad1;
float stop= radspeed;
noFill();
strokeWeight(1);
stroke(245,238,0);
arc(300,300, r, r, start, stop);
radspeed=radspeed+0.0001;
r = r + 10;
}
}
}