Research
A cellular automaton is a system of items on a grid that are either turned on or off, depending on specific rules that determine the next generation of items. These items are referred to as âcellsâ. In the simplest version of this system, a binary one-dimensional cellular automaton, each generation is represented as a horizontal line filled with squares that are either colored white (which means the cell is dead) or black (which means the cell is alive). Since the line is only a one-dimensional space, each cell will only have two neighbors, and the state of all three cells in a group (I.E. the cell in question, and its neighbor to the left and right) will be taken into account when determining the next iteration, of cells, which is seen in the next line. Dead cells are represented by a 0, and live cells are represented by a 1. Every set of 3 cells (Iâll refer to them as âparentsâ), through its combination of states, of which there are 8 possible, will determine the state of a cell below, which will always be either a 0 or a 1. A rule can be established to determine the next iteration in an âif this then thatâ fashion. In this case, âthisâ will be one of the 8 possible combination of parents, and âthatâ will be either 1 or 0. A single takes into account all 8 possible combinations of parents, and gives an output in the form of a combination of eight 0s and 1s. The eight different inputs in each rule are ordered as follows: 111, 110, 101, 100, 011, 010, 001, 000. There are 256 possible rules, categorized by their output, which will coincidentally be the binary form of a number between 0 and 255 (before 128, the first number represented with 8 digits in binary, a zero fills in the blanks until the numberâs binary representation begins). For example, rule 30, the most famous rule, is 00011110 (30 in binary is 11110), and rule 220 is 11011100. These cellular automata often will exhibit a pattern when executed with a non-random starting point. Depending on the rule, we may see patterns like fractal-like structures or combinations of lines and triangles we could find a trend for, except for rule 30, for which I couldnât find a pattern when I tested it, which is the reason why it is the most famous of the rules.
For this lab, given that as of the moment I find myself unable to code and program, I found a code on scratch.mit that was able to run al 256 rules on both random and non-random starting points, or seeds, the reference to which can be found under âreferencesâ. I also found on Wolfram MathWorld visual examples of some of the rules, which allowed me to explain their structure earlier in this document, as well as pictures of the 256 rules. I could not analyze in depth all the 256 pictures, but was unable to find a pattern in rules 86, 135, 149, 169 and 225. Since I was unable to modify the code I found, I chose to tinker with it by taking 3 different rules (rule 30, and two rules obtained through a random number generator, which were 114 and 163), and testing them on both a random and non-random seed. Because I saw the pictures of the 256 rules, I knew what I would find with a non-random seed; however, I wanted to see if I could find the same pattern when using a random seed.
Codes used
The code I used, which was able to display all the 256 rules, can be found here: https://scratch.mit.edu/projects/44786142/
Tinkering:
Rule 30 with a non-random seed:
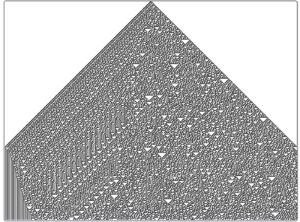
Rule 30 with a random seed:
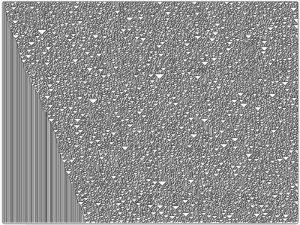
Rule 114 with a non-random seed:
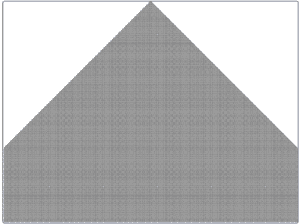
Rule 114 with a random seed:
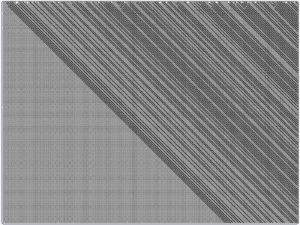
Rule 163 with a non-random seed:
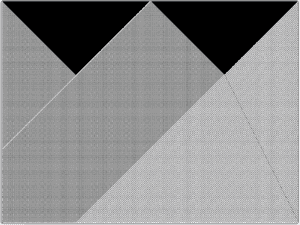
Rule 163 with a random seed:
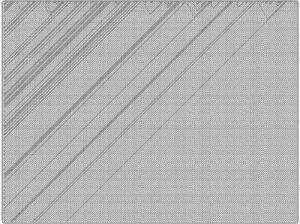
Reflection
I was able to find patterns in both rule 163 and 114 when I tried them with a random seed, and did not find one with rule 30, as I thought. With a random seed, I.E. the first line arranged randomly, we will see similar iterations for each rule, except for rule 30 and the other rules I could not find a pattern for when I looked at the 256 pictures. This shows that, just like in nature, we can find patterns in randomness. If we extrapolate this into a more complicated cellular automaton, like âGame of Lifeâ, we will see that most patterns of cells tend to stabilize and get into a repetitive pattern relatively quickly, and we will always be able to predict the next iteration, no matter how complicated, which leads me to question the existence of true random behaviors.
References:
âCellular Automaton.â From Wolfram MathWorld, 18 Feb. 2018, mathworld.wolfram.com/CellularAutomaton.html.
âElementary Cellular Automaton.â From Wolfram MathWorld, 18 Feb. 2018, mathworld.wolfram.com/ElementaryCellularAutomaton.html.
âThe 256 Rules.â
Stanford Encyclopedia of Philosophy, Stanford University, 12 Dec. 2018, plato.stanford.edu/entries/cellular-automata/supplement.html.