Step 1
In step 1, we are asked to create some graphic of our own design using parameters such as x position, y position, and color. What I drew was a little winking face
Code:
float x = 0; float y = 0; float c = 0; float a = 0; float b = 0; void setup(){ size(800,800); background(255); } void draw(){ winkingface(400,400,247,236,17); } void winkingface(float x , float y, float a , float b , float c ){ fill(a,b,c); ellipse(x,y,200,200); bezier(x-75,y+30,x-25,y+100,x+25,y+100,x+75,y+30); bezier(x-75,y+30,x-20,y+40,x+20,y+40,x+75,y+30); bezier(x-75,y-10,x-60,y-25,x-40,y-25,x-25,y-10); fill(255); ellipse(x+50,y-10,50,50); fill(#D60D43); bezier(x-20,y+40,x-20,y+110,x+20,y+110,x+20,y+40);//tongue fill(0); ellipse(x+50,y-10,25,25); }
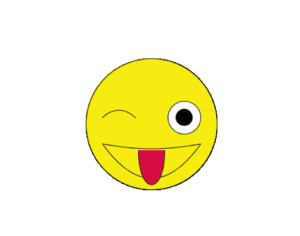
Step 2:
(1)
If the for loop is placed in setup():
Code:
float x = 0; float y = 0; float c = 0; float a = 0; float b = 0; void setup(){ size(800,800); background(255); for(int i = 0; i < 100; i++){ x = int (random(width)); y = int (random(height)); c = int(random(255)); a = int(random(255)); b = int(random(255)); winkingface(x,y,a,b,c); } } void draw(){ } void winkingface(float x , float y, float a , float b , float c ){ fill(a,b,c); ellipse(x,y,200,200); bezier(x-75,y+30,x-25,y+100,x+25,y+100,x+75,y+30); bezier(x-75,y+30,x-20,y+40,x+20,y+40,x+75,y+30); bezier(x-75,y-10,x-60,y-25,x-40,y-25,x-25,y-10); fill(255); ellipse(x+50,y-10,50,50); fill(#D60D43); bezier(x-20,y+40,x-20,y+110,x+20,y+110,x+20,y+40);//tongue fill(0); ellipse(x+50,y-10,25,25); }
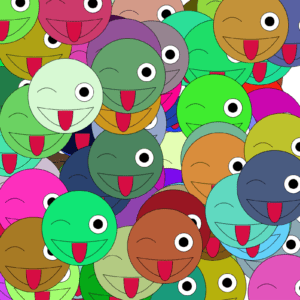
(2)
If the for loop is placed in draw():
Code:
float x = 0; float y = 0; float c = 0; float a = 0; float b = 0; void setup(){ size(800,800); background(255); } void draw(){ for(int i = 0; i < 100; i++){ x = int (random(width)); y = int (random(height)); c = int(random(255)); a = int(random(255)); b = int(random(255)); winkingface(x,y,a,b,c); } } void winkingface(float x , float y, float a , float b , float c ){ fill(a,b,c); ellipse(x,y,200,200); bezier(x-75,y+30,x-25,y+100,x+25,y+100,x+75,y+30); bezier(x-75,y+30,x-20,y+40,x+20,y+40,x+75,y+30); bezier(x-75,y-10,x-60,y-25,x-40,y-25,x-25,y-10); fill(255); ellipse(x+50,y-10,50,50); fill(#D60D43); bezier(x-20,y+40,x-20,y+110,x+20,y+110,x+20,y+40);//tongue fill(0); ellipse(x+50,y-10,25,25); }
Step 3:
Create three Arrays to store the x, y, and color data. In setup()
, fill the arrays with data using a for loop, then in draw()
use them in another for loop to display 100 instances of your graphic
float[] x = new float[100]; float[] y = new float[100]; float[] a = new float[100]; float[] b = new float[100]; float[] c = new float[100]; void setup(){ size(800,800); background(255); for(int i=0; i<x.length; i++){ x[i] =random(width); y[i] =random(height); a[i] =random(230,255); b[i] =random(255); } } void draw(){ for(int i = 0; i < 100; i++){ winkingface(x[i],y[i],a[i],b[i]); } } void winkingface(float x , float y, float a , float b ){ fill(255,a,b); ellipse(x,y,200,200); bezier(x-75,y+30,x-25,y+100,x+25,y+100,x+75,y+30); bezier(x-75,y+30,x-20,y+40,x+20,y+40,x+75,y+30); bezier(x-75,y-10,x-60,y-25,x-40,y-25,x-25,y-10); fill(255); ellipse(x+50,y-10,50,50); fill(#D60D43); bezier(x-20,y+40,x-20,y+110,x+20,y+110,x+20,y+40);//tongue fill(0); ellipse(x+50,y-10,25,25); }
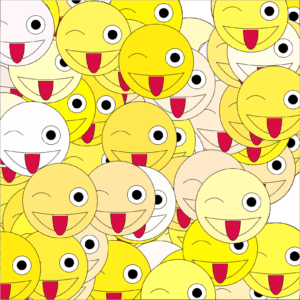
Step 4: Add individual movement to each instance of your graphic by modifying the content of the x and y arrays.
float[] x = new float[100]; float[] y = new float[100]; float[] a = new float[100]; float[] b = new float[100]; float[] c = new float[100]; void setup(){ size(800,800); background(255); for(int i=0; i<x.length; i++){ x[i] =random(width); y[i] =random(height); a[i] =random(230,255); b[i] =random(255); } } void draw(){ for(int i = 0; i < 100; i++){ winkingface(x[i],y[i],a[i],b[i]); x[i] += random(-5, 5); y[i] += random(-5, 5); } } void winkingface(float x , float y, float a , float b ){ fill(255,a,b); ellipse(x,y,200,200); bezier(x-75,y+30,x-25,y+100,x+25,y+100,x+75,y+30); bezier(x-75,y+30,x-20,y+40,x+20,y+40,x+75,y+30); bezier(x-75,y-10,x-60,y-25,x-40,y-25,x-25,y-10); fill(255); ellipse(x+50,y-10,50,50); fill(#D60D43); bezier(x-20,y+40,x-20,y+110,x+20,y+110,x+20,y+40);//tongue fill(0); ellipse(x+50,y-10,25,25); }
Question 1: In your own words, please explain the difference between having your for loop from Step 2 in setup( ) as opposed to in draw( ).
If the for loop is in the setup(), It would only run once. Therefore the variables are fixed, leading to the static graphic. While if the for loop is in draw(), it would run over and over again, every time it runs, the variables change as well, leading to the moving graphic.
Question 2: What is the benefit of using arrays? How might you use arrays in a potential project?
Using arrays allows us to store a great number of variables, and it could potentially save our time from doing tedious work like setting the variables over and over again. I could use array in my project if I want to draw numerous shapes like I did in the recitation. It could also be used when I try to choose one number as an output of a game among a certain group of numbers.