Q1. Loop in setup() make the image appear once. Then it is still. While loop in draw() will repeat infinitely.
Q2. arrays help us to arrange and control multiple variables. I may use arrayes to create an image with multiple graphics.
int numbers = 50; float[] x=new float[numbers]; float[] y=new float[numbers]; color[] c=new color[numbers]; float[] speedX =new float[50] ; float[] speedY = new float[50]; float[] x1=new float[numbers]; float[] y1=new float[numbers]; float[] speedX1 =new float[50] ; float[] speedY1 = new float[50]; color[] c1=new color[numbers]; float[] x2=new float[numbers]; float[] y2=new float[numbers]; float[] speedX2 =new float[50] ; float[] speedY2 = new float[50]; color[] c2=new color[numbers]; color[] c3=new color[numbers]; void setup(){ size(1000,1000); for(int i=0; i<x.length; i++){ x[i] = random(width); y[i] = random(height); c[i] = color(255,0, 0,random(255)); x1[i] = random(width); y1[i] = random(height); speedX[i]= random(-10, 10); speedY[i]= random(-10, 10); speedX1[i]= random(-10, 10); speedY1[i]= random(-10, 10); c1[i] = color(255,255, 0,random(255)); x2[i] = random(width); y2[i] = random(height); speedX2[i]= random(-10, 10); speedY2[i]= random(-10, 10); c2[i] = color(121,180, 255,random(255)); c3[i] = color(178,130, 247,random(255)); } } void draw(){ background(255); for(int i=0;i<x.length;i++){ display(x[i],y[i],c[i]); x[i] = x[i] + speedX[i]; y[i] = y[i] + speedY[i]; display3(x[i],y[i],c3[i]); x[i] = x[i] + speedX[i]; y[i] = y[i] + speedY[i]; display1(x1[i],y1[i],c1[i]); x1[i] = x1[i] + speedX1[i]; y1[i] = y1[i] + speedY1[i]; display2(x2[i],y2[i],c2[i]); x2[i] = x2[i] + speedX2[i]; y2[i] = y2[i] + speedY2[i]; if (y[i] > height || y[i]< 0) { speedY[i] = -speedY[i]; } if (x[i] > width || x[i]< 0) { speedX[i] = -speedX[i]; } if (y1[i] > height || y1[i]< 0) { speedY1[i] = -speedY1[i]; } if (x1[i] > width || x1[i]< 0) { speedX1[i] = -speedX1[i]; } if (y2[i] > height || y2[i]< 0) { speedY2[i] = -speedY2[i]; } if (x2[i] > width || x2[i]< 0) { speedX2[i] = -speedX2[i]; } } } void display(float x,float y, color c){ noStroke(); fill(c); ellipse(x, y, 30, 30); } void display3(float x,float y, color c3){ beginShape(); fill(c3); quad(x-25,y-18,x-25,y+18, x-13,y+5,x-13,y-5); quad(x+25,y-18,x+25,y+18, x+13,y+5,x+13,y-5); endShape(); } void display1(float x1,float y1, color c1){ fill(c1); beginShape(); vertex(x1,y1); vertex(x1+10,y1); vertex(x1+15,y1-10); vertex(x1+20,y1); vertex(x1+30,y1); vertex(x1+20,y1+5); vertex(x1+18,y1+15); vertex(x1+13,y1+10); vertex(x1+5,y1+10); vertex(x1+10,y1+5); endShape(); } void display2(float x2,float y2, color c2){ fill(c2); arc(x2+60,y2+60, 40,40, PI,PI*2, CHORD); noFill(); stroke(0); curve(x2+60,y2+60,x2+60,y2+ 60, x2+60, y2+90, x2+50,y2+90); arc(x2+60,y2+60, 45,45, PI,PI*2, CHORD); }
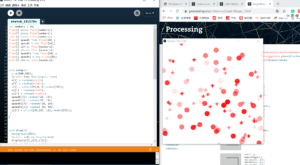
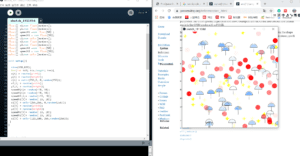