Mini Project: Particle World
Project Title: My Notebook
Link: https://rebeccax10ng.github.io/CreativeCodingLab/myNotebook_ParticleWorld/
Breif Description and Concept:
The canvas is a piece of paper from a notebook. The audience can choose where to draw some marks. The marks will float on the paper and stop on the lines. There are some functions apart from displaying and updating:
controlSpeed(): decelerates gradually.
moveUpward(): force the particles to float slowly upwards.
regrowSpeed(): make the speed of the particles change subtlely when they are in the canvas.
increaseSize(): increases the size of objects.
Visual Documentation:
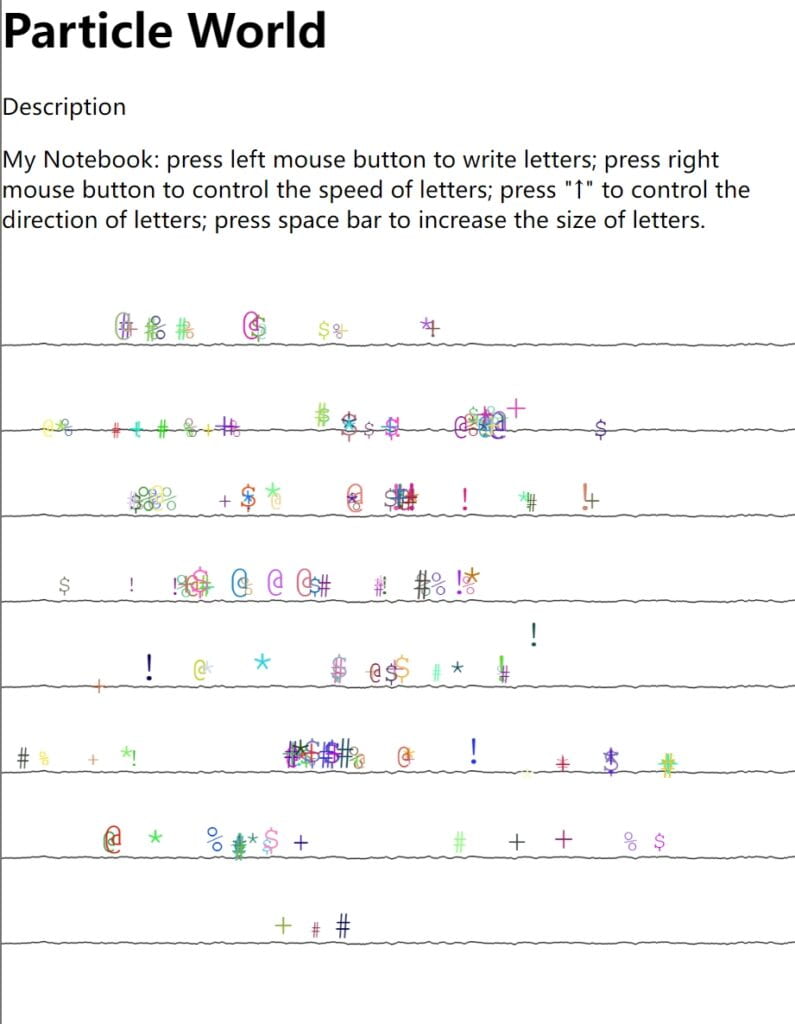
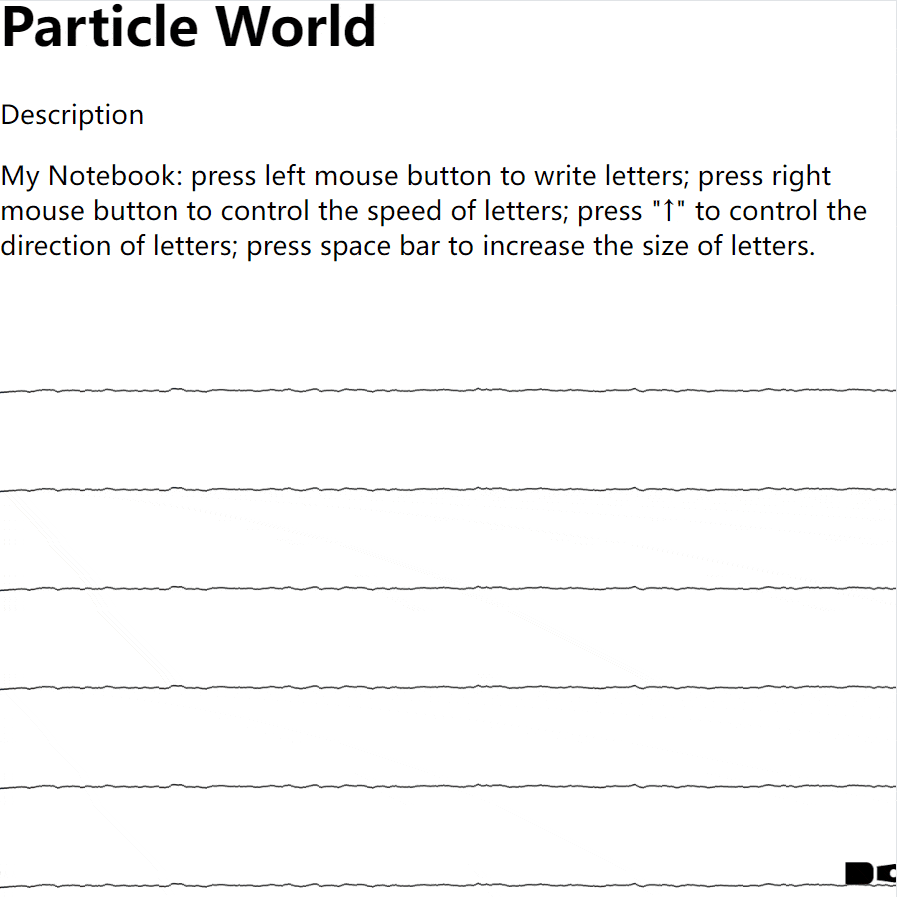
Coding: I explored 2-dimension array in this project, but the outcome is not very ideal. As is shown in the gif above, there are many lags when moving the mouse around because too many data is being processed. I used the 2-dimension array to check the distance between all points on all lines and all particles, which has exceeded the calculation capacity of the server. I think there can be a better solution to reach the ideal visual effect.
let pointPos = []; function setup() { //other code... //set up values for (let i = 0; i < NUM_OF_PARTICLES; i++) { particles[i] = new Particle(random(width), random(height)); } for (let i = 0; i <= width; i++) { pointPos[i] = []; for (let lineY = 60; lineY <= height; lineY += 60) { let pointY = noise(0.2 * i) * 3; pointPos[i].push(createVector(i, lineY + pointY)); } } } function draw() { //other code... //draw lines for (let i = 0; i < pointPos.length; i++) { for (let j = 0; j < pointPos[i].length; j++) { let pointCoord = pointPos[i][j]; point(pointCoord.x, pointCoord.y); } } //check distance for (let i = 0; i < particles.length; i++) { let p = particles[i]; let tooClose = false; for (let j = 0; j < pointPos.length; j++) { for (let k = 0; k < pointPos[j].length; k++) { let distToPoint = dist(p.x, p.y, pointPos[j][k].x, pointPos[j][k].y); if (distToPoint < 5) { tooClose = true; break; } } if (tooClose) { p.controlSpeed(); } }
Reflection:
When using functions to achieve a certain goal, saving up complexity is also important when there are too many data being processed, or it will affect the overall accessibility of program.
Q & A:
What is Object-Oriented Programming (OOP), a Class and an Instance of the Class?
-Object-Oriented Programming is a programming method that focuses on objects and parameters. It optimizes the ability to create replicable objects that share similarities. A class is a way to define an object, using data and code. An instance of the class represents a single object created with the method provided in the class.
Discuss the effectiveness of OOP. When is OOP useful? In what way can it be utilized?
-OOP is useful when we need to create large amount of similar objects or different objects which need to have some shared features. When the project includes many repetitive data or complicated data, it is recommended to use OOP to optimize the data structure and make it easier to modify.
Describe the objects you have created. What properties and methods were implemented? What kind of behaviors did you create by manipulating them?
I created some random characters that can be generated when the mouse is clicked. The type of character, their size, color, moving speed and position are controlled by the class. The user can change their moving direction, sizes and moving speed.
Please share it if there is any challenging and confusing aspect(s) in the OOP concepts and techniques.
I wonder what should be put in the OOP frame and what should not. When some movement involves checking the status of a single instant, is it better to write this code directly in the draw function?