Creative Coding Lab: Project B Documentation
Project Link:https://rebeccax10ng.github.io/CreativeCodingLab/Rebecca_ProjectB/
A. Design and Composition
As the theme of this project is “Dear Future”, I would like to make a website in memory of the past as well as create new memories, so my final decision is to make an album cover generator as well as an audio recorder.
- Interaction flow:
- The user enters a homepage
- Click “Create my album”
- Manipulate the parameters to customize the filter
- Take a picture and save the customized album filter
- (Optional) Record anything interesting, replay and save
- Close the window or go back to try other filters
- Iterations:
- Generate music notes that play random sounds on the homepage (generative background music)
- Created multiple filters and provided a filter-selecting page
- Created HTML elements that communicate with p5.js script
- Enable the disc to rotate after taking the video
- Enable sound recording, playing and saving (after the presentation)
Design Draft:
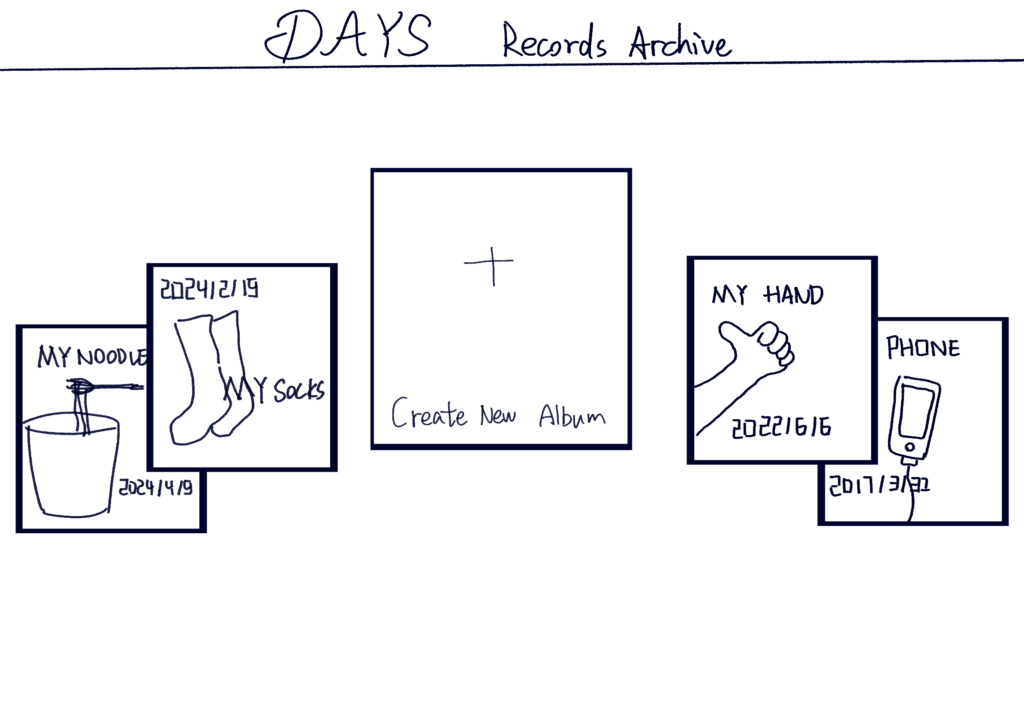
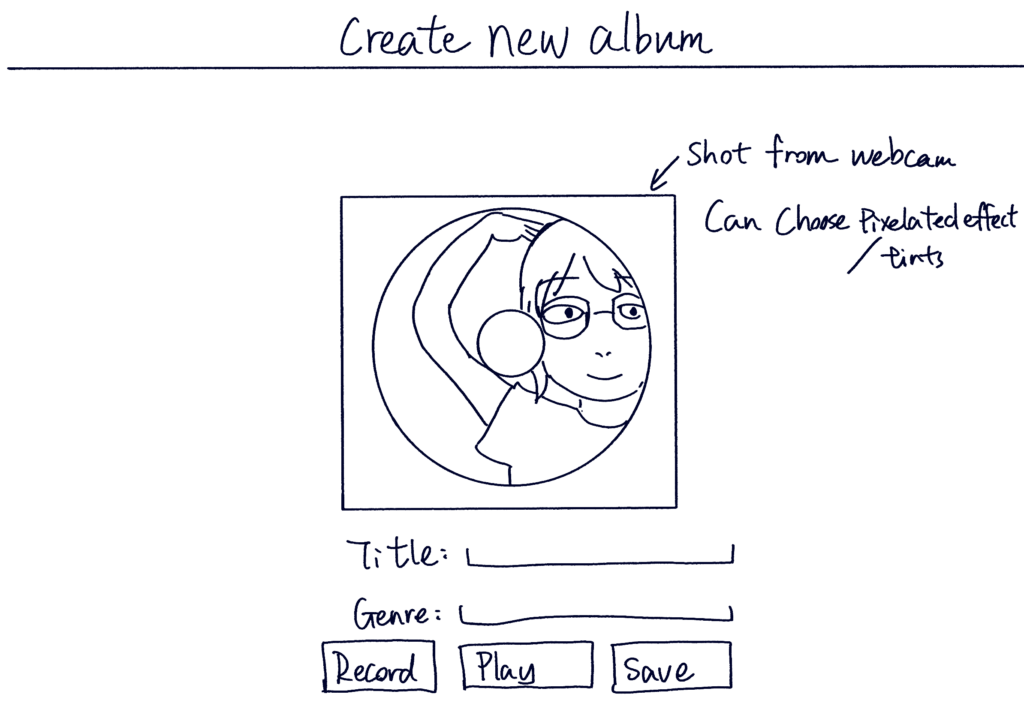
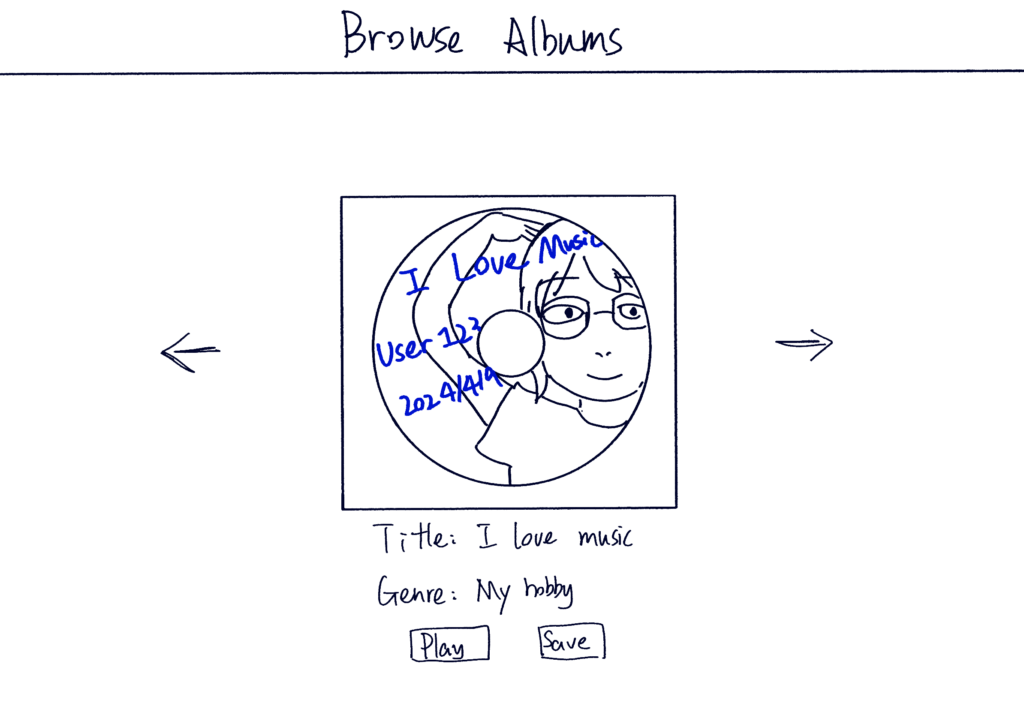
- HTML Draft:
- Main design (demo video):
B. Technical Procedures
- Html & p5.js script communication
- Description: In my project, I would like the users to manipulate the parameters using HTML input functions, like displayed in the demo video. The elements include slide bars, checkboxes, color pickers, buttons and input boxes.
- Difficulties: It is very easy to mistake the div IDs with IDs of the specific input element. Also, because event listener is actually a javascript code that fetches information, putting these codes inside the draw bracket to update every frame may cause errors.
- Code:
- P5.JS:
-
button = document.getElementById('capture'); button.addEventListener('click', function () { clickCount++; if (clickCount % 2 === 1) { frozenImage = get(); frozen = true; saveCanvas("MyAlbum.png"); } else { frozen = false; rotationAngle = 0; } });
HTML:
<div id="captureButton"> <button id="capture" onclick="changeButtonText()" class="btns">Save!</button> </div> <script> function changeButtonText() { var button = document.getElementById("capture"); if (button.innerHTML === "Save!") { button.innerHTML = "Resume"; } else { button.innerHTML = "Save!"; } } </script>
- Audio recording and playing
- Description: Enables the user to record some sounds, then play and save them in the website.
- Difficulties: There are some problems with the browser policies, and button clicking didn’t initiate the audio function, so we have to use
userStartAudio();
- Code:
- p5.js:
function startRecording() {
if (!isRecording) {
isRecording = true;
userStartAudio();
console.log("start recording")
recorder.record(soundFile);
} else {
isRecording = false;
console.log("stop recording")
recorder.stop();
}
}
function playRecording() {
if (soundFile.isPlaying()) {
soundFile.stop();
} else {
soundFile.play();
}
}
function saveAudioRecording() {
saveSound(soundFile, 'myRecording.wav');
}
HTML:
<button id="record" class="btns" onclick="startRecording()">Record Audio</button> <button id="play" class="btns" onclick="playRecording()">Play Audio</button> <button id="save" class="btns" onclick="saveAudioRecording()">Save Audio</button>
- Switch p5.js status
- Description: Use boolean and p5 functions to switch different performances in one p5.js canvas (used to make the rotating canvas effect)
- Code:
function draw(){ //other codes... if (frozen) { rotateCanvas(); } } button = document.getElementById('capture'); button.addEventListener('click', function () { clickCount++; if (clickCount % 2 === 1) { frozenImage = get(); frozen = true; saveCanvas("MyAlbum.png"); } else { frozen = false; rotationAngle = 0; } }); function rotateCanvas() { translate(width / 2, height / 2); rotate(rotationAngle); background(0); image(frozenImage, -width / 2, -height / 2); rotationAngle += 0.01; }
One of the most important technical takeaways that I learned from this project is to try to make clear blocks of codes or complete a universal template before developing multiple similar pages. In my project, after I made six filter templates, I made six copies of JavaScript and HTML scripts. After doing that, I added many new functions, which made it very complicated to modify the five left scripts. Trying to write comments for new codes and write functions that can work independently is very beneficial.
C. Reflection and Future Development
Compared with my original proposal, I added more interesting customizing functions, but there are still something that I was not able to finish so far. For example, in my proposal, I planned to generate unique URLs for users to share with their friends or come back later to view their images and recordings, but it required a database. I tried to use Google Firebase but was not successful. I also tried to do mobile phone adaptions, but the proportion was different and I didn’t have enough time to make a button to switch cameras so users can use their cameras on the back of the phone.
If I planned to polish this project in the future, I would like to add the functions above and learn more about javascript animations to do some switching animation in the homepage, and make an archive that collects all albums made via this website.
D. Credits and References
W3Schools for HTML and CSS coding
P5.js reference for audio recording and playing
The Coding Train for pixel in p5js
Professor Moon for modifying CSS layout, the event listener and audio function
Carrot Liu for CSS layout and Firebase tutorial
Ricci Liu for CSS and event listener tutorial