Mini Project: Object Dancer
Link to the project: Rebecca’s Dancer
Brief Description and Concept: This project aims to make a dancing object within an object-orientated programming frame. My dancer is a small dachshund whose body is connected by a bouncing spring.
Visual Documentation:
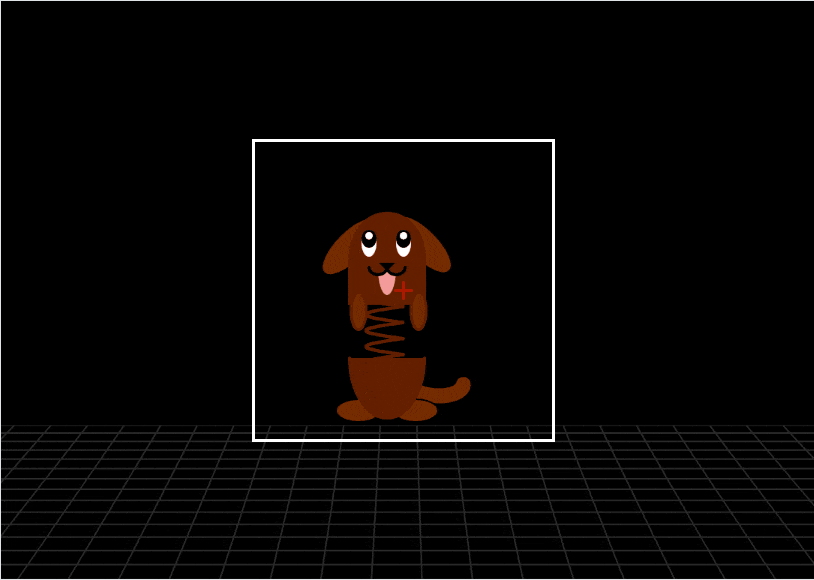
Update on March 25:
Added movement.
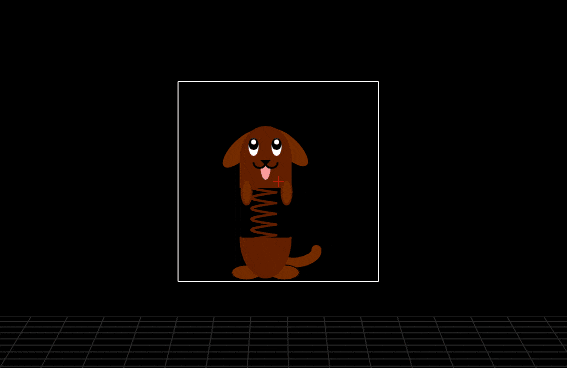
Coding:
The draft of the project is done in p5js, which enables a more instant try and error process.
let freqSpring = 3.14; let freqSpringSpeed = 0.1; let scaleIndex = 3; let headY = -30; let headYspeed = 0.5; let rotateAngle=0; let rotateSpeed=0.01 function setup() { createCanvas(200, 200); } function draw() { background(255); stroke(99, 31, 0); fill(99, 31, 0); push(); translate(width / 2, height / 2); scale(1, scaleIndex / 3); rotate(PI / 2); rectMode(CENTER); for (let springX = -30; springX < 50; springX += 0.1) { let mappedFreqSpring = map(sin(freqSpring), -1, 1, 2.5, 3.5); let springY = abs(sin(springX / mappedFreqSpring)) * 25; point(springX, springY); }scaleIndex += freqSpringSpeed; if (scaleIndex < 2.5) { freqSpringSpeed = 0.1; } else if (scaleIndex > 3.5) { freqSpringSpeed = -0.1; } console.log(mouseX - width / 2, mouseY - height / 2); pop(); //ears push(); //scale(1, scaleIndex/4); fill(117, 45, 0); translate(width / 2 - 7, height / 2 - 5 + headY); rotate(-PI / 4); ellipse(-25, -15, 50, 20); pop(); push(); //scale(1, scaleIndex/4); fill(117, 45, 0); translate(width / 2 + 20, height / 2 + 29 + headY); rotate(PI / 4); ellipse(-25, -15, 50, 20); pop(); push(); //scale(1, scaleIndex/4); translate(width / 2, height / 2 + headY); rect(-36, 10, 50, 30); arc(-11, 10, 50, 60, -PI, 0); if (headY < -60) { headYspeed = 1; } if (headY > -20) { headYspeed = -1; } //frontlegs fill(117, 45, 0); ellipse(-30, 40, 10, 25); ellipse(10, 40, 10, 25); //eyes fill(255); ellipse(-23, 0, 10, 20); ellipse(0, 0, 10, 20); fill(0); ellipse(-23, -3, 10, 12); ellipse(0, -3, 10, 12); fill(255); circle(-23,-5,5) circle(-0,-5,5) //mouth fill(255, 156, 156); arc(-11, 20 - 3, 12, 35, 0, -PI); fill(99, 31, 0); stroke(0); arc(-17, 19 - 3, 12, 10, 0, -PI); arc(-5, 19 - 3, 12, 10, 0, -PI); fill(0); triangle(-11, 20 - 3, -8, 17 - 3, -14, 17 - 3); headY += headYspeed; pop(); push(); //tail push() stroke(117, 45, 0); translate(width/2+20,height/2+60) rotate(rotateAngle) rotateAngle+=rotateSpeed if(rotateAngle>=0.2) { rotateSpeed=-0.01 } if(rotateAngle<=-0.2){ rotateSpeed=0.01 } noFill() strokeWeight(10) arc(0,0,40,20,0,PI) pop() //lowerBody translate(width / 2, height / 2); fill(117, 45, 0); ellipse(-30,80,30,15) ellipse(8,80,30,15) fill(99, 31, 0); arc(-11, 45, 50, 80, 0, -PI); pop();}
Later, the code was intergrated into the template in vscode.
Some technical problems:
How to draw a bouncing spring: My original plan was to draw several arcs and link them together, using a function to manipulate their height. However, it was very complicated and the visual outcome was not ideal. Professor Moon give me an idea of using sine function to draw a wave that looked like a spring, and using scaling fuction to change the height of the wave.
Changing the format of code: because I drew the sketch in p5js, it took me a lot of work to adjust all valuable names and positions.
Arrange the movement speed: I tried to fix this problem. The moving speed of spring and the dog’s head were different, but I couldn’t figure out how to fix that.
How to add overall movement: I added oscillation motion to the dog. However, the process was very difficult with many problems. I turned to our learning assistant Ricci, and she helped me work it out. The position of the dog is updated every frame and it moves around an ellipse in the buttom of the canvas.
Reflections:
Put updating positions in the position of every single object instead of using lots of push and pop functions.
Remember to check the spelling! Any small mistake in spelling may cause the whole program to crash;
Check if all push() and pop() are in pairs;
There are many ways to draw a shape.