Creative Coding Lab: Mini Project 1
Project Title: Selfie In The Mirror
Link:https://editor.p5js.org/jx2494/sketches/6LBwOM3-3
Visual Documentation:
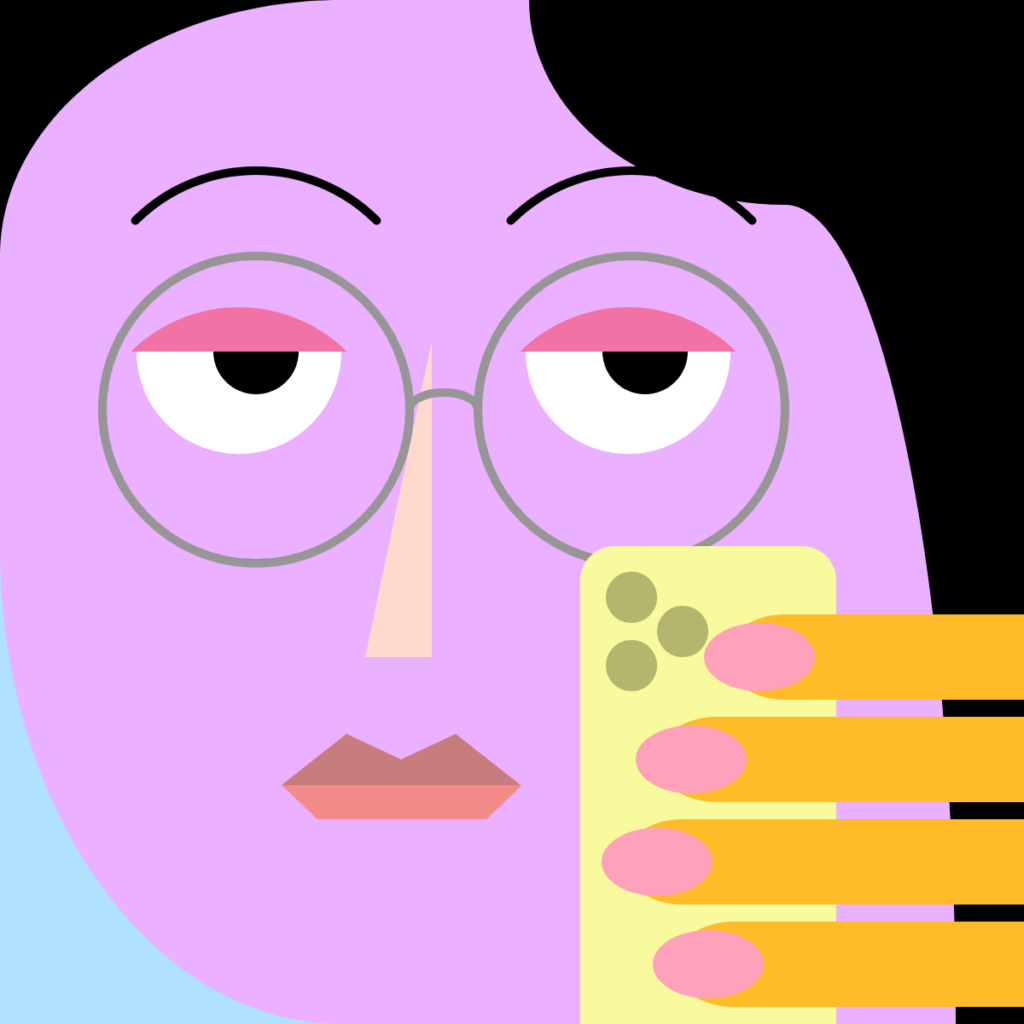
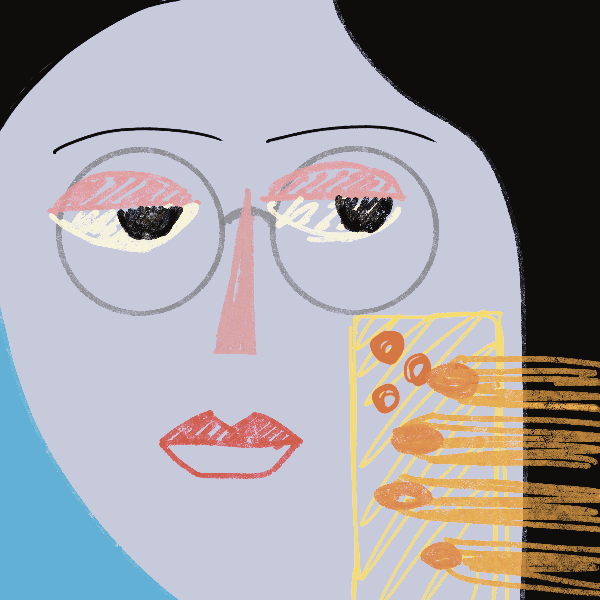
Coding: This portrait is made of basic shapes including rectangles, triangles, circles, curves and arcs. I also used beginShape() function to draw polygons that make up the lips.
I’ve encountered several difficulties. When using the curve function, I found that if I use noStroke() to hide its stroke, the curve would be invisible. After consulting my professor, I changed the color of the stroke to fit the other parts of the portrait.
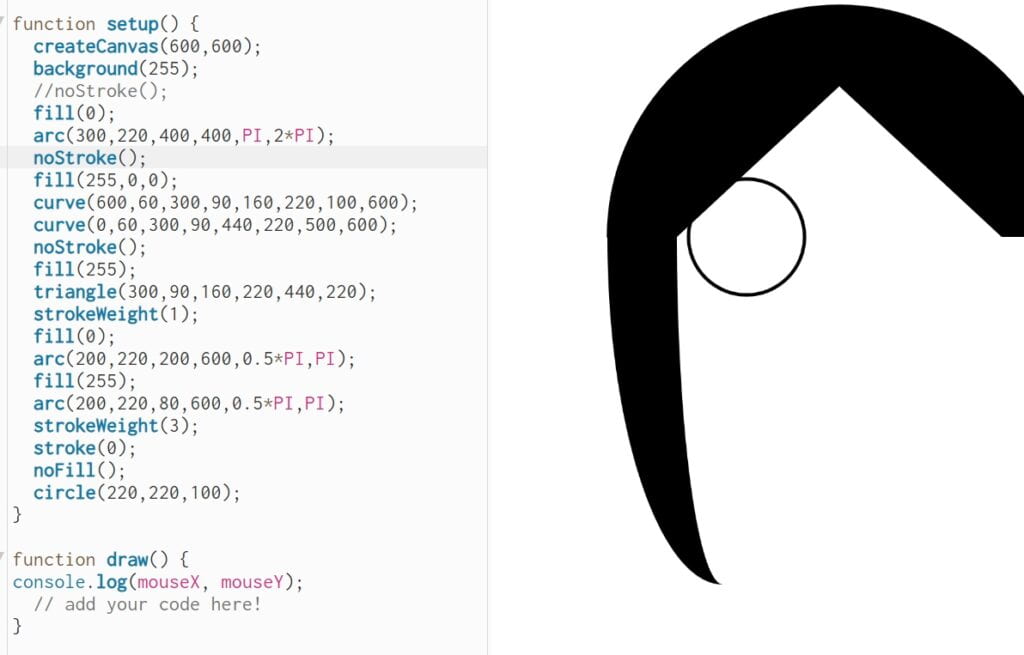
I tried to use for loop to draw fingers, but things didn’t work well. It made all fingers of the same length, so I gave up and drew 4 independent fingers. (Perhaps I can use rectMode(CENTER) and put the center on the right side of the canvas and use absolute value to calculate the length of the fingers.)
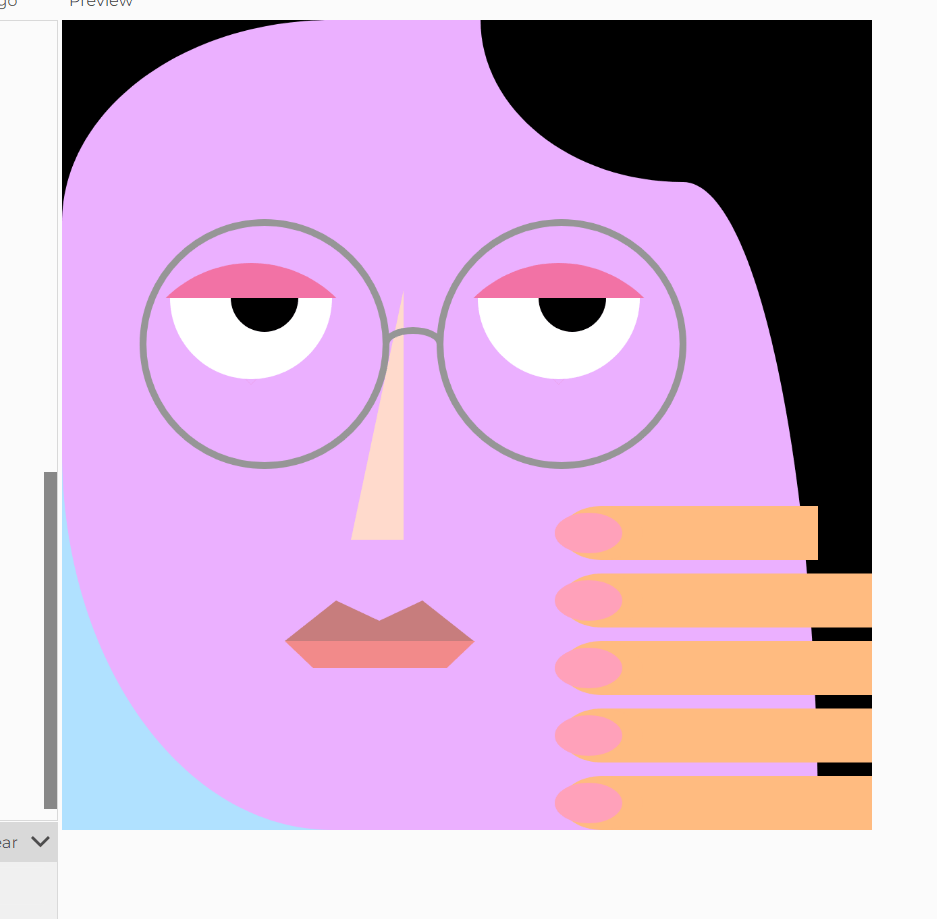
My Code:
function setup() { createCanvas(600, 600); background(235, 176, 255); fill(0); noStroke(); //hair arc(460, 0, 300, 240, 0.5 * PI, PI); rect(0, 0, 200, 150); rect(460, 0, 140, 600); fill(235, 176, 255); arc(460, 600, 200, 960, -0.5 * PI, -1.5 * PI); arc(200, 150, 400, 300, PI, 1.5 * PI); //face noStroke(); fill(176, 225, 255); rect(0, 320, 200, 280); fill(235, 176, 255); arc(200, 320, 400, 560, 0.5 * PI, PI); noStroke(); //nose fill(255, 218, 204); triangle(253, 200, 214, 385, 253, 385); //glasses noFill(); strokeWeight(5); stroke(150); circle(150, 240, 180); circle(370, 240, 180); arc(260, 240, 40, 20, PI, 2 * PI); stroke(0); arc(150, 200, 200, 200, 1.25 * PI, 1.75 * PI); arc(370, 200, 200, 200, 1.25 * PI, 1.75 * PI); //eyes noStroke(); fill(255, 0, 0, 90); arc(140, 270, 180, 180, 1.25 * PI, 1.75 * PI); fill(235, 176, 255); triangle(75, 206, 205, 206, 140, 270); fill(255, 0, 0, 90); arc(368, 270, 180, 180, 1.25 * PI, 1.75 * PI); fill(235, 176, 255); triangle(303, 206, 433, 206, 368, 270); fill(255); arc(140, 206, 120, 120, 0, PI); arc(368, 206, 120, 120, 0, PI); fill(0); arc(150, 206, 50, 50, 0, PI); arc(378, 206, 50, 50, 0, PI); fill(199, 125, 125); //mouth beginShape(); vertex(150 + 15, 460); vertex(188 + 15, 430); vertex(220 + 15, 445); vertex(252 + 15, 430); vertex(290 + 15, 460); endShape(); fill(242, 138, 138); beginShape(); vertex(165, 460); vertex(306, 460); vertex(285, 480); vertex(186, 480); endShape(); //phone fill(248, 250, 157); circle(360, 340, 40); circle(470, 340, 40); rect(360, 320, 110, 40); rect(340, 340, 150, 260); fill(180, 181, 110); circle(370, 350, 30); circle(370, 390, 30); circle(400, 370, 30); //fingers fill(255, 187, 40); rect(460, 360, 140, 50); ellipse(460, 385, 70, 50); rect(420, 420, 200, 50); ellipse(420, 445, 70, 50); rect(400, 480, 200, 50); ellipse(400, 505, 70, 50); rect(430, 540, 200, 50); ellipse(430, 565, 70, 50); fill(255, 161, 186); ellipse(445, 385, 65, 40); ellipse(405, 445, 65, 40); ellipse(385, 505, 65, 40); ellipse(415, 565, 65, 40); } function draw() { console.log(mouseX, mouseY); }
Reflection:
If the target is to save time, sometimes simple but repetitive methods are better than trying to use smart functions but keep failing;
Be more flexible, try alternatives when something doesn’t work.