Mini Project#5: Object Dancers
Project Title: beibingyang (北冰洋) polar bear
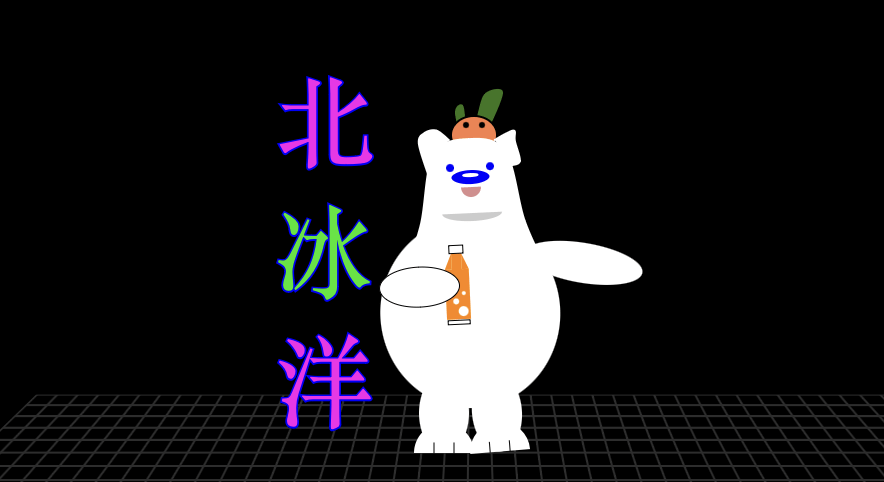
Goals
- Apply the concept of Object-Oriented Programming (OOP) to your project.
- Design and implement your own, self-contained classes; and
- Construct objects and manipulate their properties (variables) utilizing the methods (functions) that are defined in the Class(es).
Description and Concept
This is the beibingyang polar bear! The mascot (I think?) of beibingyang soda from Beijing. Growing up, whenever I went back to Beijing (my family’s hometown) to visit, I looked forward to drinking it with my relatives. I’m not really sure why it came to mind when we were assigned object dancer. Here are some photos I used for inspiration:
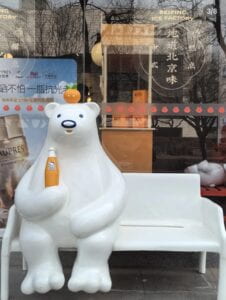
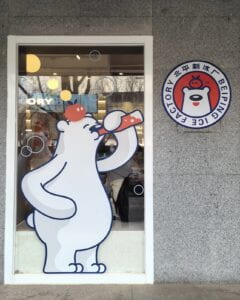
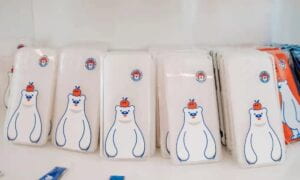
The sketch: I coded the polar bear pretty much as you see in the above photos. In my sketch it’s waving with a jumping orange on its head and holding a bottle of beibingyang.
Demo/Visual Documentation
Some parts to highlight:
(randomly generated colors)
jumping orange (that is a little screwed up)
Coding Process
As with my other sketches so far, I started just drawing the polar bear exactly how I wanted it to look! I think it’s still kinda hard for me to get out of my comfort zone–I still think coding static designs are easiest but I’m slowly learning. Anyway I felt more comfortable going ahead after I had a design to work with.
The polar bear is made up of: a head, ears, eyes, nose, mouth, body, arms, and feet. I just started with an ellipse (the body) and went from there.
Moon showed me a basic movement I could add using push and pop, translate, and rotate. Here’s a quick sketch of what it originally looked like.
Eventually I adjusted it so the shapes wouldn’t rotate endlessly 360 degrees.
For the soda bottle, I changed this code around!
Honestly when it came to referring to the object, rereading the p5.js chapter helped, but I still kind of didn’t know where to start when actually coding it. So I just started with creating properties for the colors, even though I can just directly name them, to get used to it.
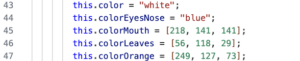

The first motion element I tried to code was the jumping orange. I don’t think I coded many variations of the same thing, but moreso it just took more time to think and try to visualize what I read in the textbook and how OOP is different from what we’ve done before. For the orange I just named this.orangeY, this.orangeW, and this.orangeH. I didn’t write one for x because I didn’t want to change the x position. I updated those coordinates after.
I used an if else statement to make it jump. Originally I had the logic reversed I think, I had -= 0.5 and += 1 instead.
To make the eyes jump along with the orange, I added them to the above if else statement. However the problem I have now-which I didn’t really end up resolving, is that the eyes jump up higher than the orange so they leave the orange’s face. You can see it in the video above. This is because the eyes and orange’s initial positions are different. I adjusted this a little by tweaking the speeds so it looked less obvious, but didn’t really do anything else. If I could fix it I’d fix it by calculating the eyes position relative to the orange’s.
These are the main properties I had for the rotation.
I used the this.angle for the head, all facial features, and body. The only great difference in speed is with the left arm rotation honestly. Because the body and head were coded separately, at first I had an issue where the head would be rotating past the ellipse (body) in a way where it was really obvious they weren’t “connected.” I think I fixed this by adjusting the anchor spot of the head/neck and the radians value.
If I could change things with this drawing:
- I had difficulty figuring out how to write code so that the left foot would stop on the way back from its rotation (at 180 degrees), and then the right foot would rotate in the other direction. I would implement this next time
- Add more variety–my dancing pretty much just comes from rotation
Reflection
- What is the benefit of your class not relying on any code outside of its own definition?
You can bundle data together. At first I was really confused at how things would work if you don’t declare any global variables, but I’m starting to see how it works. I think it also helps with conciseness.
- What make it challenging to write code that has to harmonize with the code other people have written?
I guess if you’re looking at another person’s code, you’d have to seek some information out for yourself by looking at the original variables. So this is an added step. There’s also the issue of keeping boundaries (like the square we had) in mind.
- Describe the terms modularity and reusability using the example of your dancer class.
For modularity, the class is broken up into smaller components (like move right leg, move head), and then reusability, you can reuse methods across different instances of the class. For ex, I used rotate.thisAngle a lot.
See my project here
See the code here
Sources
https://www.thebeijinger.com/blog/2021/09/23/capital-fizz-sipping-arctic-ocean-beijings-iconic-orange-soda-brand