In our second recitation, we built three different circuits and ran them with code to practice our use of Arduino. I worked with Stephen to make these.
Circuit 1: Fade
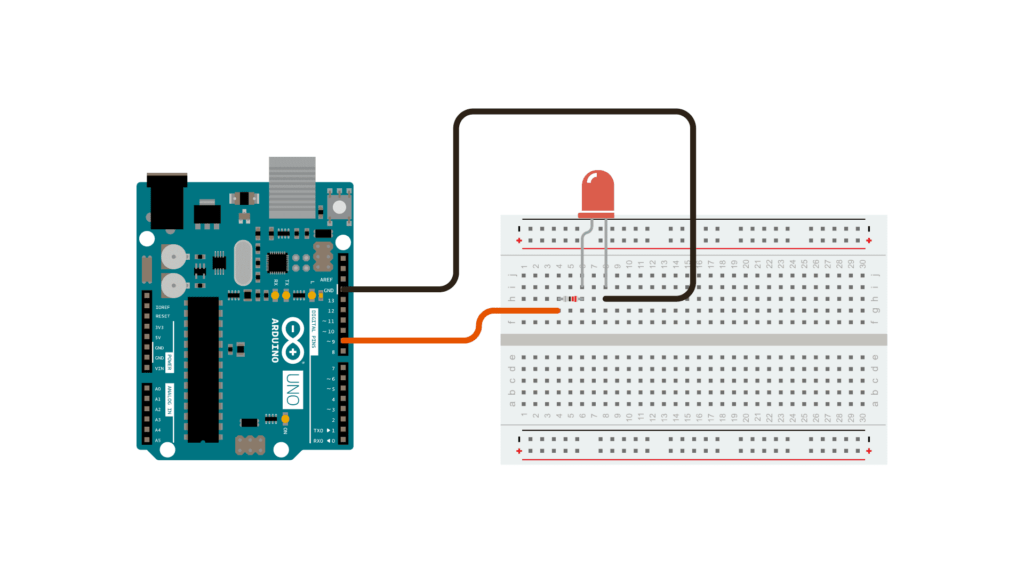
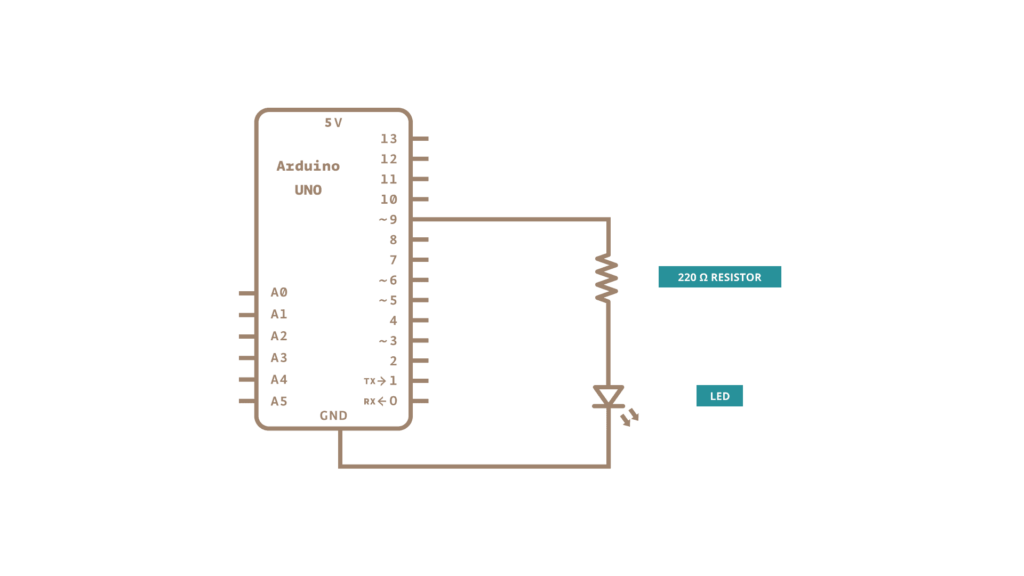
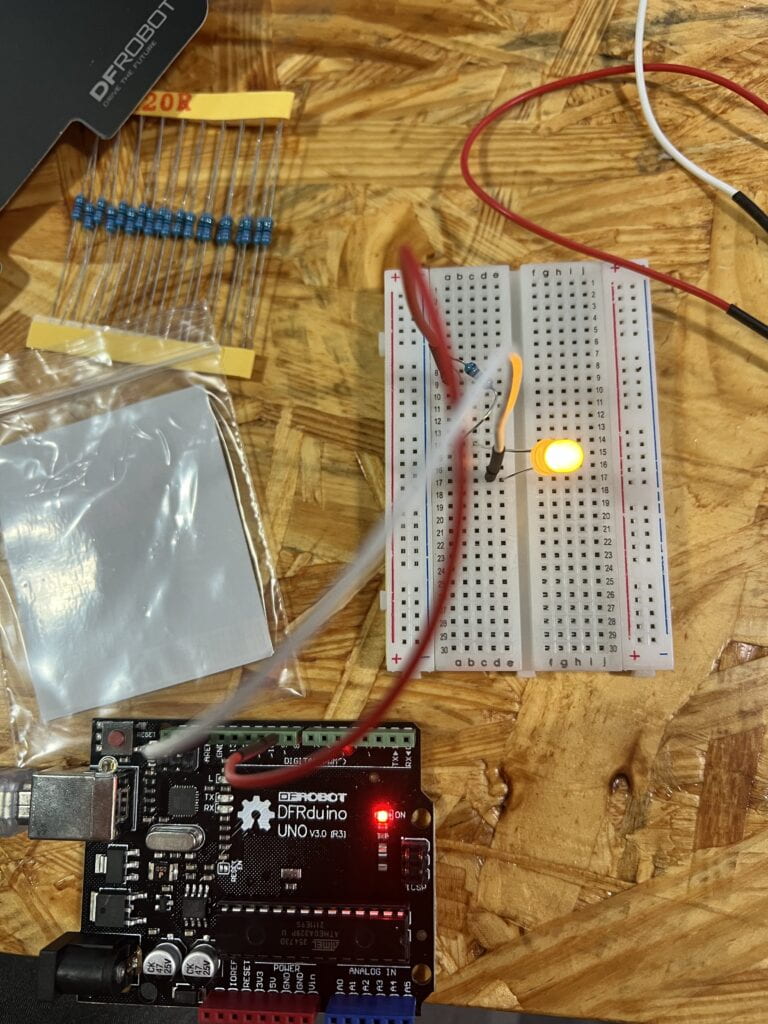
The code for circuit 1. Select from Arduino IDE > File > Examples > 03.Analog > Fading and upload it to your Arduino:
/* Fade This example shows how to fade an LED on pin 9 using the analogWrite() function. The analogWrite() function uses PWM, so if you want to change the pin you're using, be sure to use another PWM capable pin. On most Arduino, the PWM pins are identified with a "~" sign, like ~3, ~5, ~6, ~9, ~10 and ~11. This example code is in the public domain. https://www.arduino.cc/en/Tutorial/BuiltInExamples/Fade */ int led = 9; // the PWM pin the LED is attached to int brightness = 0; // how bright the LED is int fadeAmount = 5; // how many points to fade the LED by // the setup routine runs once when you press reset: void setup() { // declare pin 9 to be an output: pinMode(led, OUTPUT); } // the loop routine runs over and over again forever: void loop() { // set the brightness of pin 9: analogWrite(led, brightness); // change the brightness for next time through the loop: brightness = brightness + fadeAmount; // reverse the direction of the fading at the ends of the fade: if (brightness <= 0 || brightness >= 255) { fadeAmount = -fadeAmount; } // wait for 30 milliseconds to see the dimming effect delay(30); }
This circuit used the analogWrite() function to fade an LED light on and off. I originally made my circuit more complicated than it needed to be. I had the red wire connecting to pin 9, and the black wire connecting to ground, both connecting to extra cables (that had both ends attached to the breadboard) that then connected to the LED. I think I had gotten used to practicing with somewhat more complex circuits, and didn’t think think to follow the schematic exactly as shown until my partner commented that my circuit seemed too busy. The schematic instructed to connect the ground cable directly to the cathode, and the pin 9 cable to the anode through a resistor. After revising the circuit, I uploaded the code, which ran without problems immediately.
Circuit 2: toneMelody
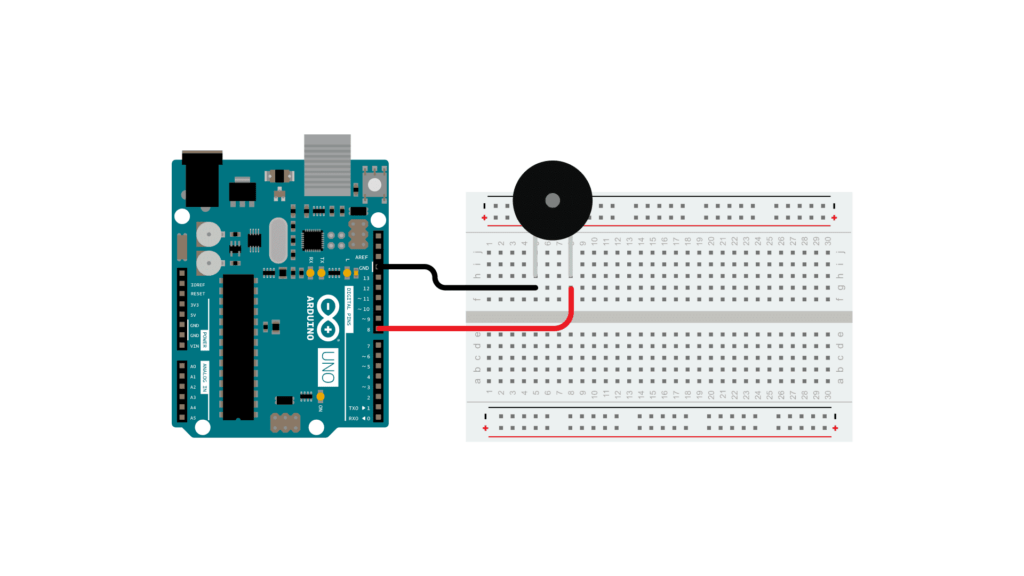
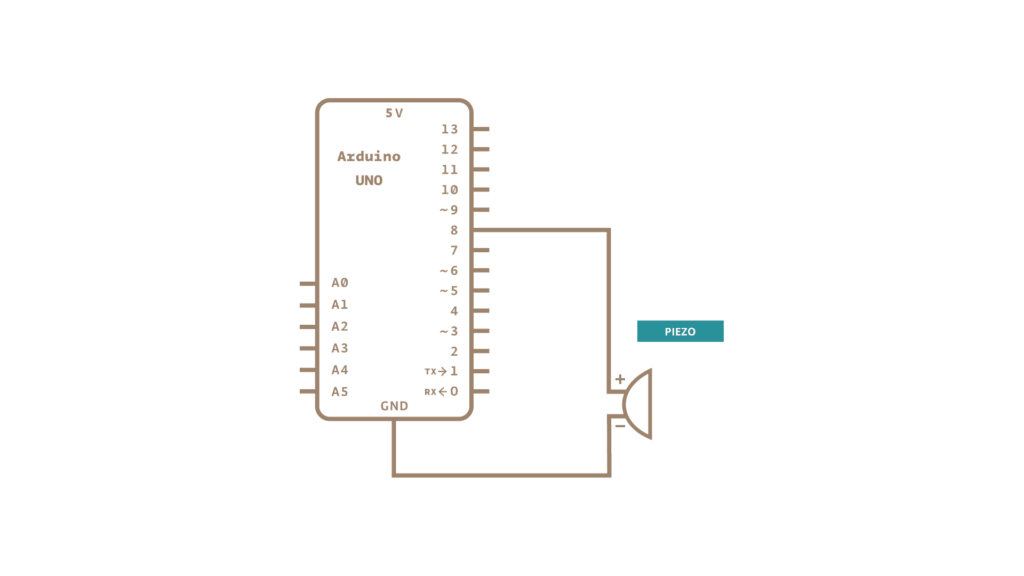
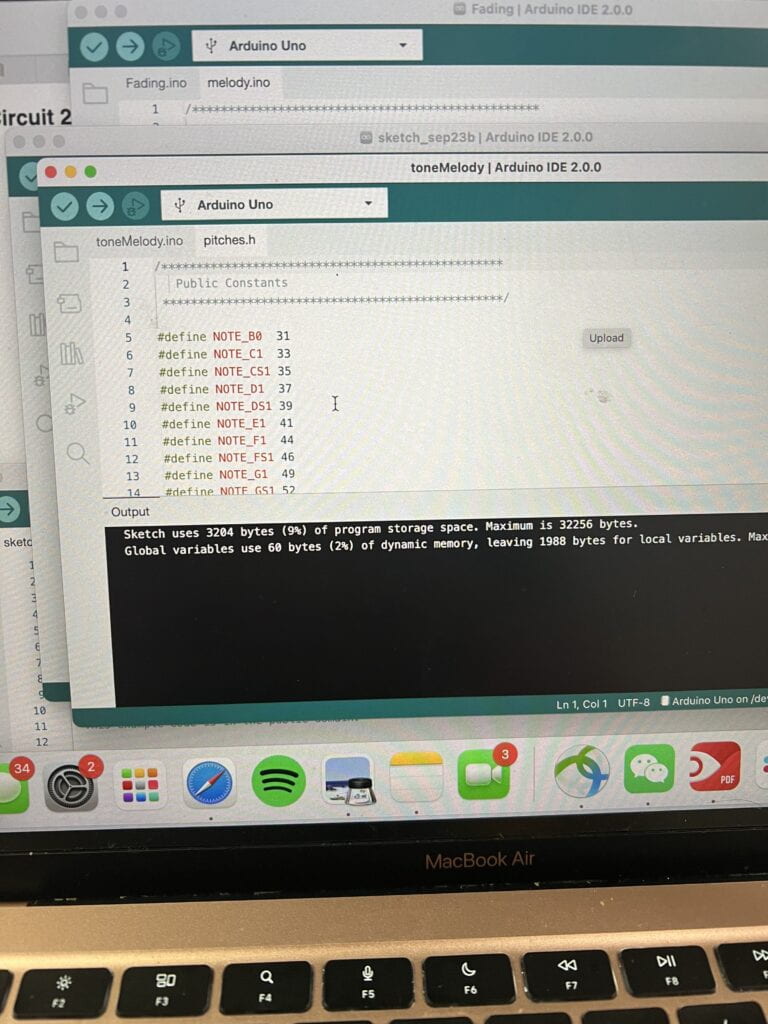
‘
Main code sketch
/* Melody Plays a melody circuit: - 8 ohm speaker on digital pin 8 created 21 Jan 2010 modified 30 Aug 2011 by Tom Igoe This example code is in the public domain. http://www.arduino.cchttps://www.arduino.cc/en/Tutorial/Tone */ #include "pitches.h" // notes in the melody: int melody[] = { NOTE_C4, NOTE_G3, NOTE_G3, NOTE_A3, NOTE_G3, 0, NOTE_B3, NOTE_C4 }; // note durations: 4 = quarter note, 8 = eighth note, etc.: int noteDurations[] = { 4, 8, 8, 4, 4, 4, 4, 4 }; void setup() { // iterate over the notes of the melody: for (int thisNote = 0; thisNote < 8; thisNote++) { // to calculate the note duration, take one second divided by the note type. //e.g. quarter note = 1000 / 4, eighth note = 1000/8, etc. int noteDuration = 1000 / noteDurations[thisNote]; tone(8, melody[thisNote], noteDuration); // to distinguish the notes, set a minimum time between them. // the note's duration + 30% seems to work well: int pauseBetweenNotes = noteDuration * 1.30; delay(pauseBetweenNotes); // stop the tone playing: noTone(8); } } void loop() { // no need to repeat the melody. }
Additional Code. The code for circuit 2. Access the code from Arduino IDE > File > Examples > 02.Digital > toneMelodyand upload it to your Arduino.
/************************************************* * Public Constants *************************************************/ #define NOTE_B0 31 #define NOTE_C1 33 #define NOTE_CS1 35 #define NOTE_D1 37 #define NOTE_DS1 39 #define NOTE_E1 41 #define NOTE_F1 44 #define NOTE_FS1 46 #define NOTE_G1 49 #define NOTE_GS1 52 #define NOTE_A1 55 #define NOTE_AS1 58 #define NOTE_B1 62 #define NOTE_C2 65 #define NOTE_CS2 69 #define NOTE_D2 73 #define NOTE_DS2 78 #define NOTE_E2 82 #define NOTE_F2 87 #define NOTE_FS2 93 #define NOTE_G2 98 #define NOTE_GS2 104 #define NOTE_A2 110 #define NOTE_AS2 117 #define NOTE_B2 123 #define NOTE_C3 131 #define NOTE_CS3 139 #define NOTE_D3 147 #define NOTE_DS3 156 #define NOTE_E3 165 #define NOTE_F3 175 #define NOTE_FS3 185 #define NOTE_G3 196 #define NOTE_GS3 208 #define NOTE_A3 220 #define NOTE_AS3 233 #define NOTE_B3 247 #define NOTE_C4 262 #define NOTE_CS4 277 #define NOTE_D4 294 #define NOTE_DS4 311 #define NOTE_E4 330 #define NOTE_F4 349 #define NOTE_FS4 370 #define NOTE_G4 392 #define NOTE_GS4 415 #define NOTE_A4 440 #define NOTE_AS4 466 #define NOTE_B4 494 #define NOTE_C5 523 #define NOTE_CS5 554 #define NOTE_D5 587 #define NOTE_DS5 622 #define NOTE_E5 659 #define NOTE_F5 698 #define NOTE_FS5 740 #define NOTE_G5 784 #define NOTE_GS5 831 #define NOTE_A5 880 #define NOTE_AS5 932 #define NOTE_B5 988 #define NOTE_C6 1047 #define NOTE_CS6 1109 #define NOTE_D6 1175 #define NOTE_DS6 1245 #define NOTE_E6 1319 #define NOTE_F6 1397 #define NOTE_FS6 1480 #define NOTE_G6 1568 #define NOTE_GS6 1661 #define NOTE_A6 1760 #define NOTE_AS6 1865 #define NOTE_B6 1976 #define NOTE_C7 2093 #define NOTE_CS7 2217 #define NOTE_D7 2349 #define NOTE_DS7 2489 #define NOTE_E7 2637 #define NOTE_F7 2794 #define NOTE_FS7 2960 #define NOTE_G7 3136 #define NOTE_GS7 3322 #define NOTE_A7 3520 #define NOTE_AS7 3729 #define NOTE_B7 3951 #define NOTE_C8 4186 #define NOTE_CS8 4435 #define NOTE_D8 4699 #define NOTE_DS8 4978
This circuit used the tone() command to generate notes, which in succession, played a melody.
This circuit was straightforward to build, as it just involved replacing the LED with a buzzer, making sure the positive leg was connected to pin 8 and the negative leg was connected to GND. The resistor was also removed.
At first, the buzzer would sound properly, but I couldn’t get it to sound the melody. I asked an LA for help, and after confirming that my circuit had no issues, she looked at my code. I had only entered the second set of code that defines the notes, and not the first set. After including all of it, the melody played.
Circuit 3: Speed Game
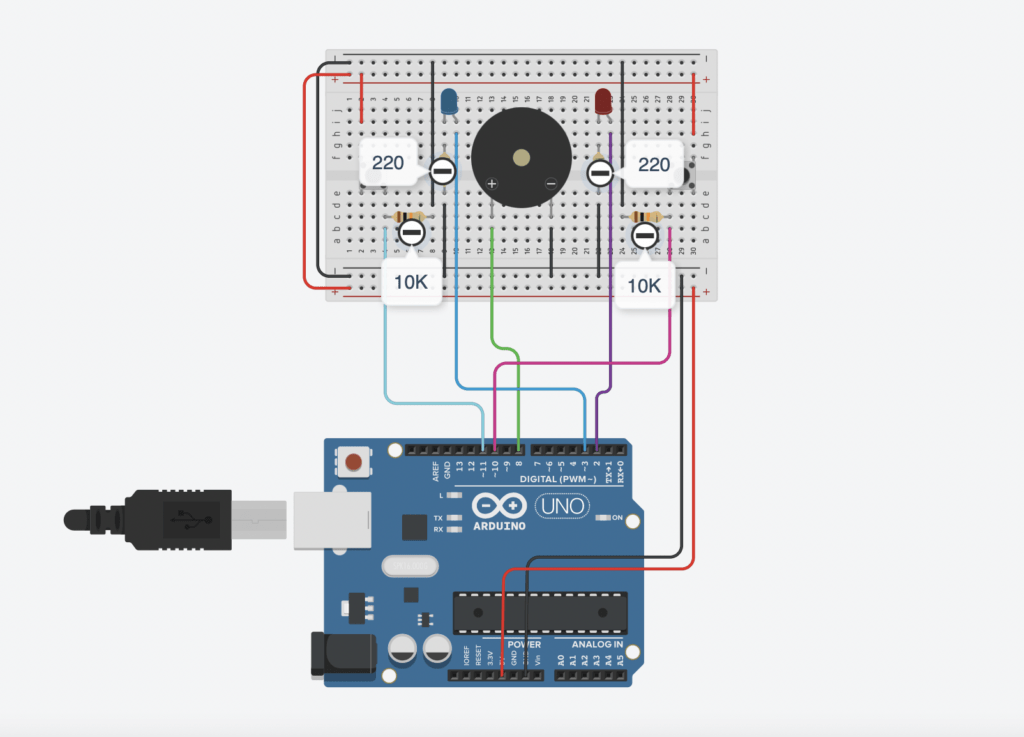
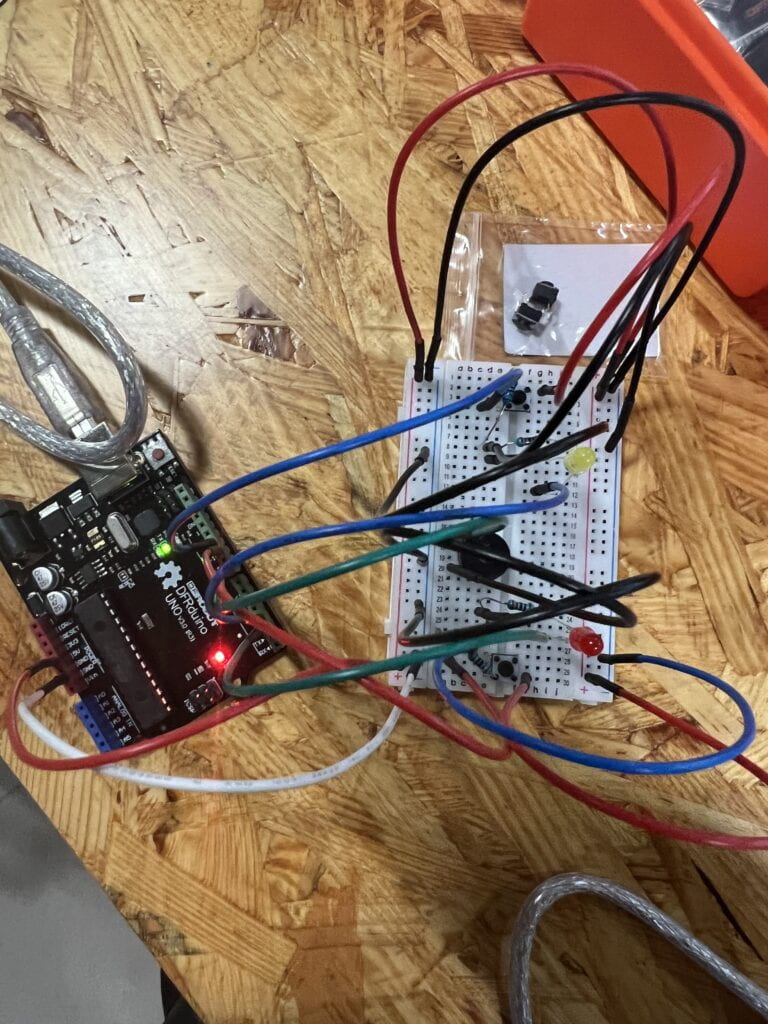
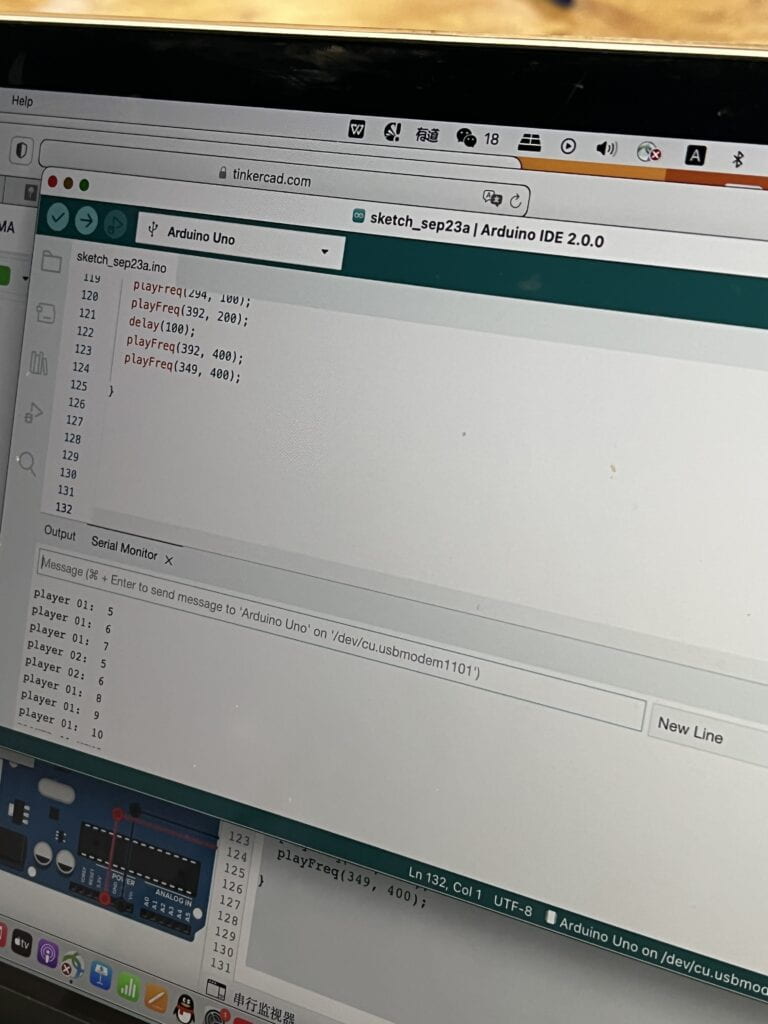
Code:
int buzzerPin = 8; int button1 = 11; int button2 = 10; int led1 = 3; int led2 = 2; int goal = 10; int buttonState1 = LOW; int previousState1 = LOW; int buttonState2 = LOW; int previousState2 = LOW; int counter1 = 0; int counter2 = 0; boolean winner1 = false; boolean winner2 = false; // the follow variables are long's because the time, measured in miliseconds, // will quickly become a bigger number than can be stored in an int. long time = 0; // the last time the output pin was toggled long debounce = 200; // the debounce time, increase if the output flickers void setup() { // put your setup code here, to run once: Serial.begin(9600); pinMode(buzzerPin, OUTPUT); pinMode(button1, INPUT); pinMode(button2, INPUT); pinMode(led1, OUTPUT); pinMode(led2, OUTPUT); Serial.println("******************* RACE THE LED *******************"); delay(1000); Serial.println("READY"); delay(1000); Serial.println("SET"); delay(1500); Serial.println("GO!!!!!!!!!!!!!!!!"); } void loop() { // put your main code here, to run repeatedly: buttonState1 = digitalRead(button1); buttonState2 = digitalRead(button2); //Serial.println(buttonState1); //this checks the times player 01 has pressed the button if (counter1 < goal && winner2 == false) { if (buttonState1 != previousState1 && millis() - time > debounce) { if (buttonState1 == HIGH) { counter1++; Serial.print("player 01: "); Serial.println(counter1); time = millis(); } } previousState1 = buttonState1; if (counter1 == goal && winner2 == false) { winner1 = true; digitalWrite(led1, HIGH); Serial.println("PLAYER 01 WINS"); playMelody(); } } //this checks the times player 02 has pressed the button if (counter2 < goal && winner1 == false) { if (buttonState2 != previousState2 && millis() - time > debounce) { if (buttonState2 == HIGH) { counter2++; Serial.print("player 02: "); Serial.println(counter2); time = millis(); } } previousState2 = buttonState2; if (counter2 == goal && winner2 == false) { winner2 = true; digitalWrite(led2, HIGH); Serial.println("PLAYER 02 WINS"); playMelody(); } } } void playFreq(double freqHz, int durationMs) { //Calculate the period in microseconds int periodMicro = int((1 / freqHz) * 1000000); int halfPeriod = periodMicro / 2; //store start time long startTime = millis(); //(millis() - startTime) is elapsed play time while ((millis() - startTime) < durationMs) { digitalWrite(buzzerPin, HIGH); delayMicroseconds(halfPeriod); digitalWrite(buzzerPin, LOW); delayMicroseconds(halfPeriod); } } void playMelody() { playFreq(262, 100); playFreq(294, 100); playFreq(349, 100); playFreq(294, 100); playFreq(440, 200); delay(100); playFreq(440, 400); playFreq(392, 400); delay(300); playFreq(262, 100); playFreq(294, 100); playFreq(349, 100); playFreq(294, 100); playFreq(392, 200); delay(100); playFreq(392, 400); playFreq(349, 400); }
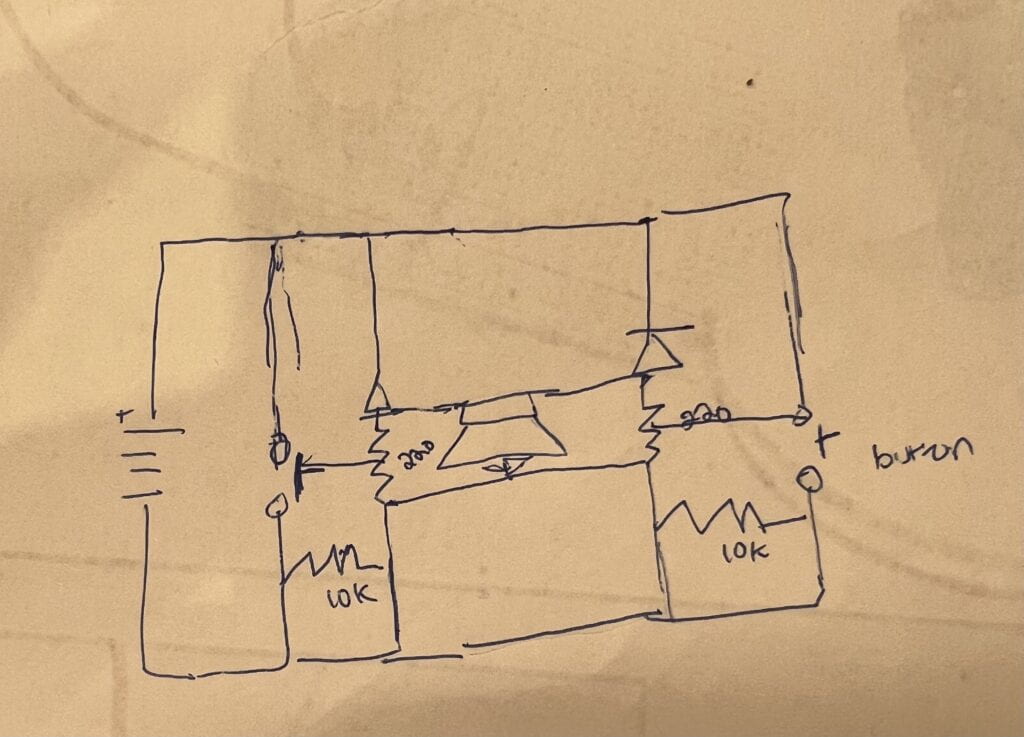
This circuit, when finished, is a two-player speed game where you race to click the button a total of 10 times, and faster than your partner. If you win, your LED flashes. I think this is my favorite circuit we’ve made so far.
We built the circuit using my equipment and following the diagram on TinkerCAD. I think I’m the type of person to be meticulous and worried that something bad will happen if I don’t follow instructions or a diagram completely, but Stephen said we didn’t need to follow the diagram completely (when it came to certain placements, like placement of some wires). We worked from left to right, adding to the circuit starting from the left hand side of the diagram and ending with the right. This circuit is mostly symmetrical, sort of like any two-player game platform like a ping pong table or tennis court. The basic structure of this circuit is: buzzer in the center across the two ravines, and one LED and button per person connected to power through a resistor and wire.
We connected the finish circuit and uploaded the code in TinkerCAD into Arduino. I found it really cool to see the game pop up in the serial monitor. I was relieved and surprised that we got it to work on the first try. Stephen won the first time and I won the second time. We unfortunately didn’t have time to try using the DIY paddles or combining the circuit with another team.
I can’t remember, but I think we made a mistake by not using two 10 k Ohm resistors, only four 220 ones.
Reflection
Q1: Propose another kind of creative button you could use in Circuit 3 to make the game more interactive. Read and use some material from the Physical Computing, Introduction Chapter (p. xvii – p. xxix) to explain why this button would make this game more interactive.
The quotations on how we can still use computers a medium for expression to its full potential resonated with me. Tom Igor and Dan Sullivan offer that “we need to think about
computers that sense more of your body, serve you in more places, and convey physical expression in addition to information” (16). I think something like making a button pad, where the person has to jump 10 times as fast as they could, could be even more fun and challenging. Another alternative would be a weight sensitive jump pad, where a level of force has to be reached. Something that might be harder to conceive is a device that can sense subtle changes in pitch, and the person who sings the highest or lowest note wins. The authors also write how intent is important to imagination–“we need to decide why it [the computer needs to take new forms. We need to take a better look at ourselves to see our full range of expression. This includes everything from the spontaneous expression on your face to the more deliberate
expression of a trained artist… humans have amazing abilities
for reading into this body language the interior state of another person” (19). I think this is an important mindset to have, and a mindset that can allow the creation of activities more accessible and that can provide joy to more groups of people, like those who might not be physically able to press a button.
Q2: Why did we use a 10 kOhm resistor with each push button?
This type of resistor is a variable resistor. 10k ohm refers to its resistance. A 10k resistor is important in acting as a pull up/down resistor for the switch. When the switch is open, the resistor connects the pin to the ground, so it reads LOW when there is no voltage flowing through, and HIGH when there is. This keeps the pin in a known state. With the 220 resistor, there is a stronger current flowing from power to ground, so the LED might blow up.
Q3: In the book Getting Started with Arduino there is a clear description about the “Arduino Way” in chapter 2. Find a project that you find interesting that can be used as an example for these kind of projects. Cite it in adequate manner, include a picture, and explain the reasons that you chose it.
The “Arduino Way” is getting comfortable in getting lost and engineering something in an unconventional way.
"I have always been fascinated by modularity and the ability to build complex systems by connect- ing together simple devices. This process is very well represented by Robert Moog and his ana- logue synthesizers. Musicians constructed sounds, trying endless combinations by patch- ing together different modules with cables. This approach made the synthesizer look like an old telephone switch, but combined with the numerous knobs, that was the perfect platform for tinkering with sound and innovating music. Moog described it as a process between “wit- nessing and discovering”. I’m sure most musi- cians at first didn’t know what all those hun- dreds of knobs did, but they tried and tried, refining their own style with no interruptions in the flow" (Banzi, 7). —Massimo
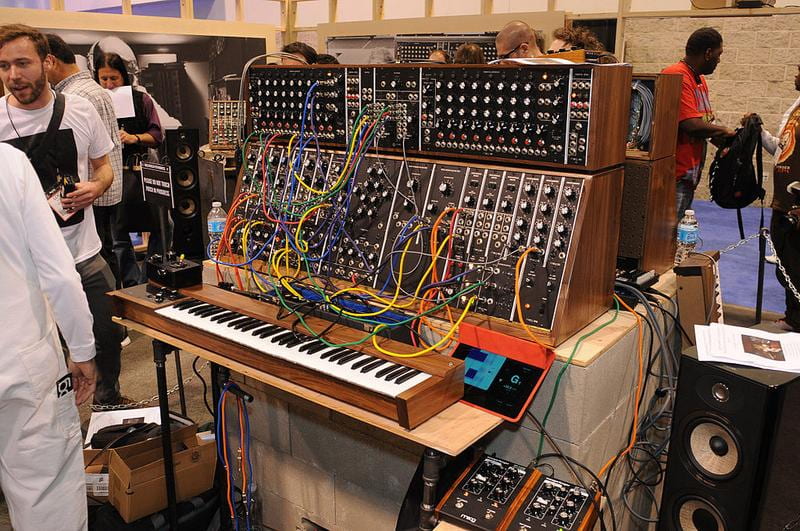
This quote stood out to me. What if we could replicate something like this, where not only professionals and creatives contribute to it, but also amateurs and regular people? The result would be something that has the identity of many people, who also have the challenge of making it cohesive in the end.