- Prototype:
- From the very beginning of the project I already had a clear sense of how the spotlight will be structured, it will have two potentiometers that move along with the turning of the spotlight and they will provide input for the location of the light.
-
- With some cardboard, wires, hot glue and some real difficult welding the prototype of the spotlight is done! (But it was not working……
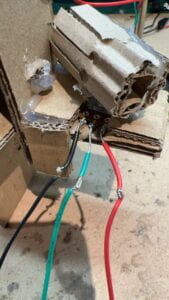
- For the final result with the suggestion from Andy, I decided to use lazier cut wood for the base and 3D print the body and the handle of the spotlight.
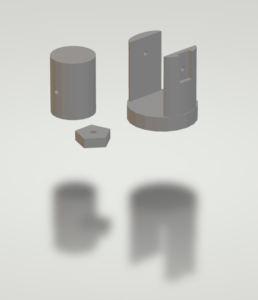
- After printing all the parts are put together with hot glue.
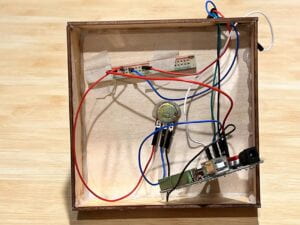
- Coding
- The major part of this project is on the coding, it involves image processing, audio and visual affects.
- Fist of all, as described in the design the project is in stages, therefore a timing system is crucial, so millis is crucial, all steps are built around the timeline.
- To display the spotlight affect I begin with filters I wished to apply the background with a dark filter and where my light shine the filter will be removed. However, in practice I realized this is beyond my reach and for a more blurred affect I chooses to use the pixels get to create a bubble visual affect.
- To have an immersive environment sound is a must, that’s why I incorporated four sound tracks in the program.
import processing.serial.*;
PImage photo;
import processing.sound.*;
SoundFile sound0;
SoundFile sound1;
SoundFile sound2;
SoundFile sound3;
float disx;
float disy;
float dis;
float n;
int nr =1;
int ns =1;
float xr;
float yr;
float press;
float timeS;
float xc;
float yc;
Serial serialPort;
int NUM_OF_VALUES_FROM_ARDUINO = 3; /* CHANGE THIS ACCORDING TO YOUR PROJECT */
/* This array stores values from Arduino */
int arduino_values[] = new int[NUM_OF_VALUES_FROM_ARDUINO];
void setup() {
size(1280, 645);
background(255);
photo = loadImage("background.jpg");
sound0 = new SoundFile(this, "40k Titan Sound.MP3");
sound1 = new SoundFile(this, "Helicopter Chopper Sound Effect.mp3");
sound2 = new SoundFile(this, "Fleeing from the War.mp3");
sound3 = new SoundFile(this, "gun fire.MP3");
printArray(Serial.list());
// put the name of the serial port your Arduino is connected
// to in the line below - this should be the same as you're
// using in the "Port" menu in the Arduino IDE
serialPort = new Serial(this, "COM10", 9600);
//delay(2000);
//int xr = int(random(photo.width));
//int yr = int(random(photo.height));
//circle(xr,yr,100);
//fill(255,0,0);
//delay(3000);//red signal dot
sound2.loop();
}
void draw() {
getSerialData();
int m = millis();
if (m>= 1000 && m<= 4000) {
for (int i=0; i<10000; i++) {
int x = int(random(photo.width));
int y = int(random(photo.height));
noStroke();
color c = photo.get(x, y);
fill(c, 255);
float size = random(7, 12);
ellipse(x, y, size, size);
}
}
if (m>= 4000 && m<= 8000) {
if (ns == 1) {
sound0.play();
sound3.play();
ns = ns+1;
}
for (int i=0; i<10000; i++) {
int xo = int(random(photo.width));
int yo = int(random(300, height));
int x = int(random(photo.width));
int y = int(random(photo.height));
noStroke();
color c = photo.get(x, y);
disx = sq(xo-x);
disy = sq(yo-y);
dis = disx + disy;
if (dis <= 1000 ) {
fill(255, 115, 0);
} else {
fill(c, 255);
}
float size = random(7, 12);
ellipse(x, y, size, size);
}
}
if (m>= 8000 && m<= 12000) {
n = map(m, 8000, 12000, 0, 255);
textSize(55);
fill (255, 255-n, 255-n);
text("In these troubled times, peace is a rare treasure.", 40, 645/7-20);
text("Amidst suffering, heroes like you emerge,", 40, 645/6*2-20);
text("bringing hope to those in need.", 40, 645/6*3-20);
}
if (m>=12000 && m<= 16000) {
n = map(m, 12000, 16000, 0, 255);
textSize(55);
fill (255, 255-n, 255-n);
text("Attention, rescue team.", 40, 645/6*4-20);
text("You're in a helicopter, scanning for survivors' flares.", 40, 645/6*5-20);
text("Stay sharp, every second counts.", 40, 645/6*6-20);
}
if (m>=16000 && m<= 20000) {
if (nr == 1) {
xr = int(random(photo.width));
yr = int(random(300, height));
nr = nr+1;
}
for (int i=0; i<10000; i++) {
int x = int(random(photo.width));
int y = int(random(photo.height));
noStroke();
color c = photo.get(x, y);
disx = sq(xr-x);
disy = sq(yr-y);
dis = disx + disy;
if (dis <= 100 ) {
fill(255, 0, 0);
} else {
fill(c, 255);
}
float size = random(7, 12);
ellipse(x, y, size, size);
}
}
if (m>=20000 && m<= 24000) {
n = map(m, 4000, 8000, 0, 255);
textSize(170);
fill (255, 255, 255);
text("SPOTLIGHT ON!", 40, height/3);
text("FIND THEM!", 40, height/3*2);
}
if (m>= 20000) {
if (sound1.isPlaying() == false) {
sound1.loop();
}
}
if (m >= 24000 && arduino_values[2] >= 1022 ) {
press = 1;
if (press ==1){
timeS = m;
press=2;
}
for (int i=0; i<10000; i++) {
// int() is needed to turn the result from random
// into a whole number
xc = map(arduino_values[1], 0, 258, 0, width);
yc = map(arduino_values[0], 309, 488, 0, height);
int x = int(random(photo.width));
int y = int(random(photo.height));
// get the pixel color
noStroke();
color c = photo.get(x, y);
// draw a circle with the color
disx = sq(xc-x);
disy = sq(yc-y);
dis = disx + disy;
if (dis <= 1000 ) {
fill(255, 255, 255);
} else {
fill(c, 255);
}
float size = random(7, 12);
ellipse(x, y, size, size);
}
}
if (m >= 24000 && arduino_values[2] <= 1000 && press ==2 ) {
float timeC = m-timeS;
float acu = sqrt(sq(xc-xr)+sq(yc-yr));
if (timeC <= 5000 && acu <= 30){
textSize(55);
fill (255, 255, 255);
text("Outstanding! All of the people are saved!", 40, 645/6-20);
text("They are now safe and in good hands,", 40, 645/6*2-20);
text("thanks to your bravery and skill.", 40, 645/6*3-20);
press =3;
}
else if (timeC <= 10000 && acu <= 90){
textSize(55);
fill (255, 100, 100);
text("We lost some along the way.", 40, 645/6-20);
text("Keep honing your skills for next time,", 40, 645/6*2-20);
text("You're making a difference, even when it's tough.", 40, 645/6*3-20);
text("Every second counts. Lives hang in the balance.", 40, 645/6*4-20);
press =3;
}
else {
textSize(55);
fill (255, 0, 0);
text("Mission failed.", 40, 645/6-20);
text("Another heart-wrenching loss in the unforgiving reality of war.", 40, 645/6*2-20);
text("Let's keep searching, to save every life we can.", 40, 645/6*3-20);
press =3;
}
}
// the helper function below receives the values from Arduino
// in the "arduino_values" array from a connected Arduino
// running the "serial_AtoP_arduino" sketch
// (You won't need to change this code.)
}
void getSerialData() {
while (serialPort.available() > 0) {
String in = serialPort.readStringUntil( 10 ); // 10 = '\n' Linefeed in ASCII
if (in != null) {
print("From Arduino: " + in);
String[] serialInArray = split(trim(in), ",");
if (serialInArray.length == NUM_OF_VALUES_FROM_ARDUINO) {
for (int i=0; i<serialInArray.length; i++) {
arduino_values[i] = int(serialInArray[i]);
}
}
}
}
}
Arduino:
void setup() {
Serial.begin(9600);
}
void loop() {
int sensor0 = analogRead(A0);
int sensor1 = analogRead(A1);
int sensor2 = analogRead(A5);
Serial.print(sensor0);
Serial.print(",");
Serial.print(sensor1);
Serial.print(",");
Serial.print(sensor2);
Serial.println();
delay(20);
}