Last Tree Standing
— — Project Report
A.
Our project is called “Last Tree Standing.” The instructor is our excellent teacher, Rodolfo Cossovich.
This story tells about a world engulfed in desert during the apocalypse. As the savior of humanity, you are tasked with opening a time-space tunnel to travel back 1000 years and save the last remaining plant from destruction.
B.
Our definition of interaction is to allow the users to understand how to engage without direct prompts. We aim for the users to have authentic and meaningful experiences through this interaction. By chance, I came across an article about environmental conservation. Inspired by it, we decided to use our midterm project as a means to convey environmental protection concepts to others. During our research, we came across an existing interactive installation:
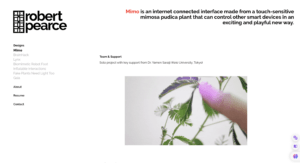
We found the interactive form of plant interaction fascinating. Initially, we had an idea about triggering leaf movement through touch, but it was later rejected due to technical reasons (which I will explain later). Then, I came up with the second idea of the time-travel tunnel. The tunnel requires crawling, and I believe it is an implied interaction that users will understand without direct instructions, reflecting my understanding of interaction. Additionally, I believe that a “large-scale” crawling installation adds uniqueness to my project.
C.
This is our initial sketch, which was later replaced due to the unstable operation of the electromagnet.
This is our second sketch.
Because we want interaction to have a subtle impact without direct prompts, our designs aim to achieve this as much as possible. For example, our lighting design. The tunnel is a dark environment, and we have covered all the areas where light could leak with black tape, making the entire tunnel pitch black without any additional light sources. We designed a light strip along the walls to direct the light into the interior of the tunnel, guiding participants to crawl forward. We believe that humans are phototactic, meaning that in a dark environment, the instinct is to move towards the light. The light strip provides a continuous source of light compared to individual LEDs, so we chose the light strip. Additionally, since this is a “high-tech” tunnel, we believe that cool-colored light, such as a light blue, can evoke a sense of technology. Therefore, we selected a pale blue light strip.
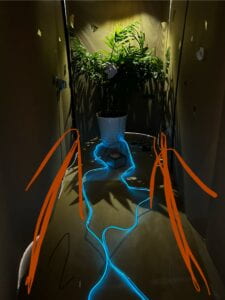
Moreover, why did we choose to use a real plant instead of constructing a paper one? We believe that a real plant is more authentic, something that a cardboard-made plant cannot replicate. Although our current form of interaction does not involve direct interaction with the real plant but rather with a button, we still believe that by illuminating the real plant from above, we can deepen its sense of sanctity and evoke a sense of saving it in the minds of the participants. We do not want the entire project to be based on a virtual environment. Even though the time-space tunnel itself may be surreal, we want to convey to the participants that this endeavor is not devoid of meaning by incorporating elements of reality.
D.
In the first half of this project, our main focus was on coding the plant interaction and dealing with the electromagnet. For the plant interaction, we defined a resistor as a capacity sensor, where touching the plant would change the capacitance. We used this to control the if statements. The code is as follows:
//Libraries
#include //https://github.com/PaulStoffregen/CapacitiveSensor
//Parameters
CapacitiveSensor s1 = CapacitiveSensor(2, 6);
long val1;
void setup() {
//Init Serial USB
Serial.begin(9600);
pinMode(4,OUTPUT);
}
void loop() {
digitalWrite(4, HIGH);
val1 = s1.capacitiveSensor(30);
Serial.println(val1);
if (val1 > 1000 )
{
digitalWrite(4, LOW);
}
else
{
digitalWrite(4,HIGH);
}
}
However, the electromagnet was the most challenging part. Its operation was highly unpredictable. Sometimes it would work during experiments but fail when connected to the circuit, which was incredibly frustrating.
This is the code for the electromagnet:
//Libraries
#include //https://github.com/PaulStoffregen/CapacitiveSensor
//Parameters
bool autocal = 0;
const int numReadings = 10;
long readings [numReadings];
int readIndex = 0;
long total = 0;
const int sensitivity = 1000;
const int thresh = 200;
const int csStep = 10000;
CapacitiveSensor cs = CapacitiveSensor(2, 6);
void setup() {
//Init Serial USB
Serial.begin(9600);
Serial.println(F("Initialize System"));
//Init cs
if (autocal == 0) {
{
cs.set_CS_AutocaL_Millis(0xFFFFFFFF);
}
}
pinMode(4,OUTPUT);
}
void loop() {
digitalWrite(4, HIGH);
Serial.println(smooth());
if (smooth() > 9000 )
{
digitalWrite(4, LOW);
}
else
{
digitalWrite(4,HIGH);
}
}
long smooth() { /* function smooth */
////Perform average on sensor readings
long average;
// subtract the last reading:
total = total - readings[readIndex];
// read the sensor:
readings[readIndex] = cs.capacitiveSensor(sensitivity);
// add value to total:
total = total + readings[readIndex];
// handle index
readIndex = readIndex + 1;
if (readIndex >= numReadings) {
readIndex = 0;
}
// calculate the average:
average = total / numReadings;
return average;
}
After realizing the instability of the electromagnet, we attempted to find alternative solutions. During the user testing process, our classmates and Rudi mentioned that they wanted more feedback. They didn’t understand why they needed to touch the plant, and after the paper leaves fell, they were unsure of what to do next and what it signified. We also noticed that, as Rudi pointed out, there wasn’t a strong connection between a real plant and a tree made of cardboard. Therefore, we needed to establish a connection. In this process, the idea of the time-space tunnel to save the plant was born.
In this new project, the most challenging part was controlling the process of the flower wilting. I wanted the effect where when a person enters the tunnel, they would see the flower gradually wilting, and when successfully rescued, the flower would stand upright again. The solution I chose was to use a fine thread motor for manipulation. I got inspiration for this from the fifth recitation.
Here is my overall code:
#include
#include
Servo myservo;
int buttonPin = 3;
int buttonPressCount = 0;
int prevButtonState = LOW;
bool motorRunning = true;
unsigned long motorStartTime = 0;
int greenLedPin = 4;
int redLedPin = 5;
int buzzerPin = 6;
void setup() {
Serial.begin(9600);
myservo.attach(9);
pinMode(buttonPin, INPUT_PULLUP);
pinMode(greenLedPin, OUTPUT);
pinMode(redLedPin, OUTPUT);
pinMode(buzzerPin, OUTPUT);
}
void loop() {
if (motorRunning) {
if (millis() - motorStartTime >= 2000) {
// 舵机运动的代码
static int motorAngle = 0;
if (motorAngle <= 160) { myservo.write(motorAngle); motorAngle += 20; } else { motorRunning = false; // 停止舵机运动 } motorStartTime = millis(); } } int buttonState = digitalRead(buttonPin); if (buttonState != prevButtonState && buttonState == HIGH) { delay(50); // 软件去抖动延迟 buttonState = digitalRead(buttonPin); // 重新读取按钮状态 if (buttonState == HIGH) { buttonPressCount++; Serial.print("Button pressed: "); Serial.println(buttonPressCount); if (buttonPressCount >= 11) {
motorRunning = false; // 停止舵机运动
digitalWrite(greenLedPin, HIGH); // 点亮绿灯
digitalWrite(redLedPin, LOW); // 熄灭红灯
playFailMusic();
}
}
}
if (!motorRunning && buttonPressCount < 11) {
digitalWrite(redLedPin, HIGH); // 点亮红灯
digitalWrite(greenLedPin, LOW); // 熄灭绿灯
playFailMusic();
}
prevButtonState = buttonState;
delay(100);
}
void playFailMusic() {
tone(buzzerPin, 247, 200); // 发出音调(B3),持续200毫秒
delay(250); // 等待250毫秒
tone(buzzerPin, 233, 200); // 发出音调(降B3),持续200毫秒
delay(250); // 等待250毫秒
tone(buzzerPin, 220, 200); // 发出音调(A3),持续200毫秒
delay(250); // 等待250毫秒
tone(buzzerPin, 208, 200); // 发出音调(降A3),持续200毫秒
delay(250); // 等待250毫秒
tone(buzzerPin, 196, 200); // 发出音调(G3),持续200毫秒
delay(250); // 等待250毫秒
tone(buzzerPin, 185, 200); // 发出音调(降G3),持续200毫秒
delay(250); // 等待250毫秒
noTone(buzzerPin); // 停止播放音调
}
To align with our overall topic, humans need to have love for plants in order to save them. We designed the button in the shape of a heart to symbolize this love.
To make the entire process more seamless, I designed a door curtain that opens the “space-time tunnel door” when the button is pressed. My partner selected the material for the door curtain, and I took charge of connecting the entire circuit and programming the motor.
Here is the code:
#include
const int servo2Pin = 6;
const int servo3Pin = 5;
const int buttonPin = 2;
Servo servo2;
Servo servo3;
void setup() {
Serial.begin(9600);
pinMode(buttonPin, INPUT_PULLUP);
servo2.attach(servo2Pin);
servo3.attach(servo3Pin);
servo2.write(0);
servo3.write(90);
delay(1000);
servo2.detach();
servo3.detach();
}
void loop() {
//Serial.println(digitalRead(buttonPin));
if (digitalRead(buttonPin) == LOW) {
servo2.attach(servo2Pin);
servo3.attach(servo3Pin);
servo2.write(90);
servo3.write(0);
delay(1000);
servo2.detach();
servo3.detach();
}
delay(100);
}
After we completed the overall construction, we conducted user tests with eight individuals. They provided feedback that the tunnel may be a bit too narrow, making it inconvenient for people with larger body sizes to move around. Additionally, due to the tight seals, the lack of ventilation inside the tunnel made it feel stuffy and uncomfortable. After experiencing both failed and successful rescue attempts, they provided feedback that they were expecting more feedback. The two small LED lights were not sufficient to meet their expectations.
Therefore, I slightly widened the space. However, I still wanted the tunnel to create a sense of urgency for them, as they are “saving humanity” after all.Due to time constraints, I was unable to address the feedback regarding feedback after successful and failed attempts. However, I did relocate the LED lights to a more prominent position to make them more visible.
Due to my partner falling ill, I took on the responsibility of handling most of the construction and coding tasks. My partner, despite being unwell, contributed significantly to the decoration aspects of the project.
E.
Since the entire tunnel is enclosed, the following videos are all filmed from a first-person perspective by my users.
Our project’s goal is to raise awareness about the importance of environmental conservation, so that events like saving plants by traveling through time don’t have to happen in the future. We are delighted to see that without any prompting, people automatically crawl into the tunnel and understand the need to press the buttons. In this regard, it aligns perfectly with our definition of interaction. However, there are several issues that could be addressed if we had more time. We need more visual feedback; currently, the results are only indicated by small LED lights and a buzzer. Given more time, a possible improvement would be to change the entire lighting environment, turning it green for success and red for failure.
Throughout the project, I have learned a lot. For instance, during circuit construction, I struggled with the messiness, which made it difficult to differentiate between components. Therefore, in future projects, I plan to use methods like color coding to distinguish between wires. From the failure of the electromagnetic coil, I learned that if something doesn’t work, it’s important not to get too fixated on it. Instead, either find an immediate replacement or “fake it till you make it.” It’s crucial not to waste excessive time on a single issue.
F.
The above parts are the ones I took apart, and I will return them to the teachers who lent them to me.There are two buttons, a string of LED lights, 10 electromagnets, 3 Arduino boards, four transistors, four diodes, four servos, and two motors.
G.
I would like to take this opportunity to once again express my deep gratitude to Professor Rodolfo Cossovich, LAs, and fellows who have provided me with tremendous help and ideas. I would also like to give a special thanks to my friends: Jenny, Isabel, Arial, Benjamin, Kitty, and Emily. I am incredibly grateful for their assistance throughout my project, whether it was through words of encouragement, guidance with coding, or providing insightful perspectives. Thank you so much to all of them!