Ultrasonic Ranger: [1] The Ultrasonic Ranger (AKA Ultrasonic Sensor OR HC-SR04) can be used to detect distance. To program the Arduino to work with this sensor, you can use this post or use the Ping example .
[1] (Arduino>File>Examples>0.6Sensors>Ping). In order for the HC-SR04 to work with the Ping code, you MUST connect both Trig and Echo to the same Digital Pin in your Arduino.
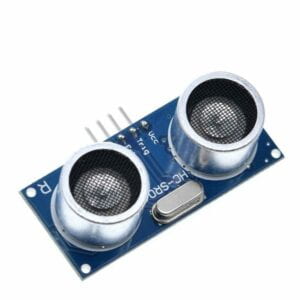
Picture from sparkfun.com
Question 1: What did you intend to assemble in the recitation exercise? If your sensor/actuator combination were to be used for pragmatic purposes, who would use it, why would they use it, and how could it be used?
We want to connect the led lights to the ultrasonic rangefinder to create a light that varies in response to the object’s distance. This device is suitable for use on a deep-sea sounder. The stronger the light is, the closer the object in front of you is. This device has the potential to act as a watchdog.
Question 2: Code is often compared to following a recipe or tutorial. Why do you think that is?
Code is similar to a recipe or tutorial in that it instructs a machine on what to do with its physical components in order to create outcomes as a function. In comparison, newborns have the instruments, in the shape of body parts, to conduct motor actions such as movement if their parents tell or teach them how. Similarly, computers and other types of hardware, such as the arduino, can transmit electricity via different hard components to achieve diverse functions, but they can’t unless someone, in this instance code, teaches them how to direct their signals and electricity.
Question 3: In The Language of New Media, Manovich describes the influence of computers on new media. In what ways do you believe the computer influences our human behaviors?
I feel that the computer’s fast-forward features, as well as its access to social media, have introduced new hobbies and interests to computer users. Furthermore, improved computation enables people to build more algorithms and applications, allowing for greater creativity.
<pre>
<font color=”#434f54″>// —————————————————————- //</font>
<font color=”#434f54″>// Arduino Ultrasoninc Sensor HC-SR04</font>
<font color=”#434f54″>// Re-writed by Arbi Abdul Jabbaar</font>
<font color=”#434f54″>// Using Arduino IDE 1.8.7</font>
<font color=”#434f54″>// Using HC-SR04 Module</font>
<font color=”#434f54″>// Tested on 17 September 2019</font>
<font color=”#434f54″>// —————————————————————- //</font>
<font color=”#5e6d03″>#define</font> <font color=”#000000″>echoPin</font> <font color=”#000000″>2</font> <font color=”#434f54″>// attach pin D2 Arduino to pin Echo of HC-SR04</font>
<font color=”#5e6d03″>#define</font> <font color=”#000000″>trigPin</font> <font color=”#000000″>3</font> <font color=”#434f54″>//attach pin D3 Arduino to pin Trig of HC-SR04</font>
<font color=”#00979c”>int</font> <font color=”#000000″>outputValue</font> <font color=”#434f54″>=</font> <font color=”#000000″>9</font><font color=”#000000″>;</font> <font color=”#434f54″>// value output to the PWM (analog out)</font>
<font color=”#00979c”>int</font> <font color=”#000000″>led</font> <font color=”#434f54″>=</font> <font color=”#000000″>9</font><font color=”#000000″>;</font>
<font color=”#434f54″>// defines variables</font>
<font color=”#00979c”>long</font> <font color=”#000000″>duration</font><font color=”#000000″>;</font> <font color=”#434f54″>// variable for the duration of sound wave travel</font>
<font color=”#00979c”>int</font> <font color=”#000000″>distance</font><font color=”#000000″>;</font> <font color=”#434f54″>// variable for the distance measurement</font>
<font color=”#00979c”>int</font> <font color=”#000000″>analogOutPin</font><font color=”#000000″>;</font>
<font color=”#00979c”>void</font> <font color=”#5e6d03″>setup</font><font color=”#000000″>(</font><font color=”#000000″>)</font> <font color=”#000000″>{</font>
<font color=”#d35400″>pinMode</font><font color=”#000000″>(</font><font color=”#000000″>led</font><font color=”#434f54″>,</font> <font color=”#00979c”>OUTPUT</font><font color=”#000000″>)</font><font color=”#000000″>;</font>
<font color=”#d35400″>pinMode</font><font color=”#000000″>(</font><font color=”#000000″>trigPin</font><font color=”#434f54″>,</font> <font color=”#00979c”>OUTPUT</font><font color=”#000000″>)</font><font color=”#000000″>;</font> <font color=”#434f54″>// Sets the trigPin as an OUTPUT</font>
<font color=”#d35400″>pinMode</font><font color=”#000000″>(</font><font color=”#000000″>echoPin</font><font color=”#434f54″>,</font> <font color=”#00979c”>INPUT</font><font color=”#000000″>)</font><font color=”#000000″>;</font> <font color=”#434f54″>// Sets the echoPin as an INPUT</font>
<b><font color=”#d35400″>Serial</font></b><font color=”#434f54″>.</font><font color=”#d35400″>begin</font><font color=”#000000″>(</font><font color=”#000000″>9600</font><font color=”#000000″>)</font><font color=”#000000″>;</font> <font color=”#434f54″>// // Serial Communication is starting with 9600 of baudrate speed</font>
<b><font color=”#d35400″>Serial</font></b><font color=”#434f54″>.</font><font color=”#d35400″>println</font><font color=”#000000″>(</font><font color=”#005c5f”>”Ultrasonic Sensor HC-SR04 Test”</font><font color=”#000000″>)</font><font color=”#000000″>;</font> <font color=”#434f54″>// print some text in Serial Monitor</font>
<b><font color=”#d35400″>Serial</font></b><font color=”#434f54″>.</font><font color=”#d35400″>println</font><font color=”#000000″>(</font><font color=”#005c5f”>”with Arduino UNO R3″</font><font color=”#000000″>)</font><font color=”#000000″>;</font>
<font color=”#000000″>}</font>
<font color=”#00979c”>void</font> <font color=”#5e6d03″>loop</font><font color=”#000000″>(</font><font color=”#000000″>)</font> <font color=”#000000″>{</font>
<font color=”#434f54″>// Clears the trigPin condition</font>
<font color=”#d35400″>digitalWrite</font><font color=”#000000″>(</font><font color=”#000000″>trigPin</font><font color=”#434f54″>,</font> <font color=”#00979c”>LOW</font><font color=”#000000″>)</font><font color=”#000000″>;</font>
<font color=”#d35400″>delayMicroseconds</font><font color=”#000000″>(</font><font color=”#000000″>2</font><font color=”#000000″>)</font><font color=”#000000″>;</font>
<font color=”#434f54″>// Sets the trigPin HIGH (ACTIVE) for 10 microseconds</font>
<font color=”#d35400″>digitalWrite</font><font color=”#000000″>(</font><font color=”#000000″>trigPin</font><font color=”#434f54″>,</font> <font color=”#00979c”>HIGH</font><font color=”#000000″>)</font><font color=”#000000″>;</font>
<font color=”#d35400″>delayMicroseconds</font><font color=”#000000″>(</font><font color=”#000000″>10</font><font color=”#000000″>)</font><font color=”#000000″>;</font>
<font color=”#d35400″>digitalWrite</font><font color=”#000000″>(</font><font color=”#000000″>trigPin</font><font color=”#434f54″>,</font> <font color=”#00979c”>LOW</font><font color=”#000000″>)</font><font color=”#000000″>;</font>
<font color=”#434f54″>// Reads the echoPin, returns the sound wave travel time in microseconds</font>
<font color=”#000000″>duration</font> <font color=”#434f54″>=</font> <font color=”#d35400″>pulseIn</font><font color=”#000000″>(</font><font color=”#000000″>echoPin</font><font color=”#434f54″>,</font> <font color=”#00979c”>HIGH</font><font color=”#000000″>)</font><font color=”#000000″>;</font>
<font color=”#434f54″>// Calculating the distance</font>
<font color=”#000000″>distance</font> <font color=”#434f54″>=</font> <font color=”#000000″>duration</font> <font color=”#434f54″>*</font> <font color=”#000000″>0.034</font> <font color=”#434f54″>/</font> <font color=”#000000″>2</font><font color=”#000000″>;</font> <font color=”#434f54″>// Speed of sound wave divided by 2 (go and back)</font>
<font color=”#434f54″>// Displays the distance on the Serial Monitor</font>
<font color=”#434f54″>//Serial.print(“Distance: “);</font>
<font color=”#434f54″>//Serial.print(distance);</font>
<font color=”#434f54″>//Serial.println(” cm”);</font>
<font color=”#434f54″>// map it to the range of the analog out:</font>
<font color=”#000000″>outputValue</font> <font color=”#434f54″>=</font> <font color=”#d35400″>map</font><font color=”#000000″>(</font><font color=”#000000″>distance</font><font color=”#434f54″>,</font> <font color=”#000000″>0</font><font color=”#434f54″>,</font> <font color=”#000000″>20</font><font color=”#434f54″>,</font> <font color=”#000000″>255</font><font color=”#434f54″>,</font> <font color=”#000000″>0</font><font color=”#000000″>)</font><font color=”#000000″>;</font>
<b><font color=”#d35400″>Serial</font></b><font color=”#434f54″>.</font><font color=”#d35400″>println</font><font color=”#000000″>(</font><font color=”#000000″>outputValue</font><font color=”#000000″>)</font><font color=”#000000″>;</font>
<font color=”#434f54″>// change the analog out value:</font>
<font color=”#434f54″>//analogWrite(analogOutPin, outputValue);</font>
<font color=”#d35400″>analogWrite</font><font color=”#000000″>(</font><font color=”#000000″>led</font><font color=”#434f54″>,</font><font color=”#000000″>outputValue</font><font color=”#000000″>)</font><font color=”#000000″>;</font>
<font color=”#000000″>}</font>
<font color=”#000000″>}</font>
</pre>
// —————————————————————- //
// Arduino Ultrasoninc Sensor HC-SR04
// Re-writed by Arbi Abdul Jabbaar
// Using Arduino IDE 1.8.7
// Using HC-SR04 Module
// Tested on 17 September 2019
// —————————————————————- //
#define echoPin 2 // attach pin D2 Arduino to pin Echo of HC-SR04
#define trigPin 3 //attach pin D3 Arduino to pin Trig of HC-SR04
int outputValue = 9; // value output to the PWM (analog out)
int led = 9;
// defines variables
long duration; // variable for the duration of sound wave travel
int distance; // variable for the distance measurement
int analogOutPin;
void setup() {
pinMode(led, OUTPUT);
pinMode(trigPin, OUTPUT); // Sets the trigPin as an OUTPUT
pinMode(echoPin, INPUT); // Sets the echoPin as an INPUT
Serial.begin(9600); // // Serial Communication is starting with 9600 of baudrate speed
Serial.println(“Ultrasonic Sensor HC-SR04 Test”); // print some text in Serial Monitor
Serial.println(“with Arduino UNO R3”);
}
void loop() {
// Clears the trigPin condition
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
// Sets the trigPin HIGH (ACTIVE) for 10 microseconds
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Reads the echoPin, returns the sound wave travel time in microseconds
duration = pulseIn(echoPin, HIGH);
// Calculating the distance
distance = duration * 0.034 / 2; // Speed of sound wave divided by 2 (go and back)
// Displays the distance on the Serial Monitor
//Serial.print(“Distance: “);
//Serial.print(distance);
//Serial.println(” cm”);
// map it to the range of the analog out:
outputValue = map(distance, 0, 20, 255, 0);
Serial.println(outputValue);
// change the analog out value:
//analogWrite(analogOutPin, outputValue);
analogWrite(led,outputValue);
}
}
IMG_3721IMG_3722