Recitation day!
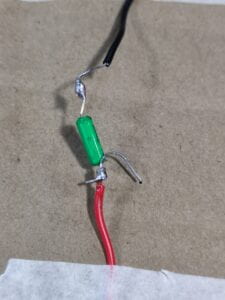
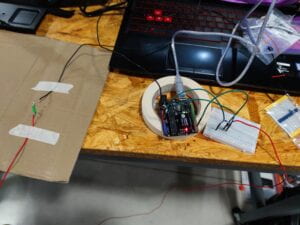
First,I soldered the sensor to the two wires to make a switch.Next, I built the circuit with the switch,a 10k ohm resistor and a capacitor. Then I sticked the switch to my right arm and began to type the codes.
Step 4 was basically divided into 3 tasks.
For task1,I used a conditional that had the criteria so that the sensor only detected transitions LOW to HIGH, showing only when the user had raised their arm completely. Here is the code and its demonstration.
int SENSOR_PIN = 2;
int tiltVal;
int prevTiltVal;
void setup() {
pinMode(SENSOR_PIN, INPUT); // Set sensor pin as an INPUT pin
Serial.begin(9600);
}
void loop() {
// read the state of the sensor
tiltVal = digitalRead(SENSOR_PIN);
// if the tilt sensor value changed, print the new value
if (tiltVal != prevTiltVal) {
if(tiltVal == 1){
Serial.println(tiltVal);
prevTiltVal = tiltVal;
}
}
delay(10);
}
For task 2, I needed to add a variable called”count” to count the curls.Here is how it works.
int SENSOR_PIN = 2;
int tiltVal;
int prevTiltVal;
int count=0;
void setup() {
pinMode(SENSOR_PIN, INPUT); // Set sensor pin as an INPUT pin
Serial.begin(9600);
}
void loop() {
// read the state of the sensor
tiltVal = digitalRead(SENSOR_PIN);
// if the tilt sensor value changed, print the new value
if (tiltVal != prevTiltVal) {
if(tiltVal == 1){
count=count+1;
Serial.println(count);
delay(1000);
;
}
}
delay(10);
}
prevTiltVal = tiltVal;
}
When it comes to task 3,I had to set a limit and reset the curls.Here is the code and its demonstration.
int SENSOR_PIN = 2;
int tiltVal;
int prevTiltVal;
int count=0;
void setup() {
pinMode(SENSOR_PIN, INPUT); // Set sensor pin as an INPUT pin
Serial.begin(9600);
}
void loop() {
// read the state of the sensor
tiltVal = digitalRead(SENSOR_PIN);
// if the tilt sensor value changed, print the new value
if (tiltVal != prevTiltVal) {
if(tiltVal == 1){
count=count+1;
Serial.println(count);
if(count>=8){
Serial.println(“Yay, you have done one set of curls”);}
if(count>=8){
count=0;
delay(1000);
;
}
}
delay(10);
}
prevTiltVal = tiltVal;
}
For step 5,I set the time interval between two groups of training and added the words””It’s time to workout!Let’s go!” to remind the user that a new group of training was about to start.
int SENSOR_PIN = 2;
int tiltVal;
int prevTiltVal;
int count=0;
void setup() {
pinMode(SENSOR_PIN, INPUT); // Set sensor pin as an INPUT pin
Serial.begin(9600);
}
void loop() {
// read the state of the sensor
tiltVal = digitalRead(SENSOR_PIN);
// if the tilt sensor value changed, print the new value
if (tiltVal != prevTiltVal) {
if(tiltVal == 1){
count=count+1;
Serial.println(count);
if(count>=8){
Serial.println(“Yay, you have done one set of curls”);}
if(count>=8){
count=0;
delay(10000);
Serial.println(“It’s time to workout!Let’s go!”);
;
}
}
delay(10);
}
prevTiltVal = tiltVal;
}
(Since there was something wrong with my sensor,the poor contact might affect the demonstration.)
And this was how the device worked:
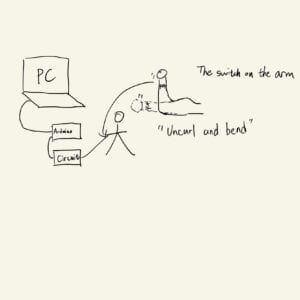
Some reflections:
1.Be careful not to mistake the positive side with the negative side when building the circuit.
2.When typing the code, I can click the line of the brackets to check whether it has a start combined with an end.It’s also useful to use “blank space” and “TAB” to make the structure clearer.
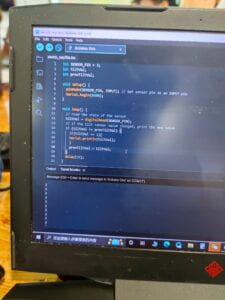
3.The transition between high and low occurs when the angle reaches 50-60 degrees,which is not so precise and needs to be improved.
4.If I rotate the arm with the sensor, it will count as one curl as long as the sensor feels the transition from horizontal to vertical.But if I shake it,the result is uncertain.Therefore,we should design a bigger device so that it will keep the position of the arm stable and avoid the mentioned situations.
5.Thanks to the device’s simplicity and convenience , I think it can be used by any user,from the young to the old.
6.What’s more,it may indicate a new way to work out—-the”interactive gym” which allows everybody to work out with the device,no matter where they are.