Part 1: Grid Pattern
I started the design of my patterns by creating a rectangle with four 90-degree arcs on each corner. Creating the function and filling the window with the same pattern was successful, but I encountered the first problem with drawing the arcs. I spent a lot of time trying the angles and center point positions. I also created an array of the color of the arcs. The color of the four arcs will be randomly selected between the three pre-set colors in the array.
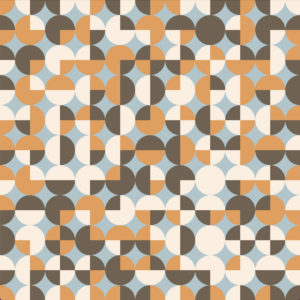
Here is my code:
int s = 100; void setup() { size(1000, 1000); background(255); for (int y=0; y<height; y=y+s) { println("y =" +y); for (int x=0; x<width; x=x+s) { println("x =" +x); push(); //push and pop should be in the for loop translate(x, y); //first translate and then draw my pattern flower(); pop(); } } } void draw() { } void flower() { noStroke(); fill(#B1C5CB); rect(0, 0, s, s); color[] arcColor = new color[3]; arcColor[0] = #DE9F60; arcColor[1] = #FAEFE1; arcColor[2] = #6F6252; noStroke(); fill(arcColor[int(random(0, 3))]); arc(0, 0, 100, 100, 0, HALF_PI); noStroke(); fill(arcColor[int(random(0, 3))]); //I don't need to set all the previous new ints arc(100, 100, 100, 100, PI, PI+HALF_PI); noStroke(); fill(arcColor[int(random(0, 3))]); arc(0, 100, 100, 100, PI+HALF_PI, 2*PI); noStroke(); fill(arcColor[int(random(0, 3))]); arc(100, 0, 100, 100, HALF_PI, PI); }
Part 2: Moving Shapes
Then I created an array of the color of the arcs. The color of the four arcs will be randomly selected between the three pre-set colors in the array. The colors will also change between orange, cream-white, and brown.
Here is how it finally looks like:
Here is my code:
int s = 100; int n = height/s*width/2; int centralC = 60; //float[] x1 = new float[n]; //float[] y1 = new float[n]; void setup() { size(900, 900); background(#FAEFE1); //patter(); } void draw() { for (int y=0; y<height; y=y+s) { println("y =" +y); for (int x=0; x<width; x=x+s) { push(); //push and pop should be in the for loop translate(x, y); //first translate and then draw my pattern patternBase(); pop(); } } delay(300); for (int y1=0; y1<1000; y1=y1+s) { for (int x1=0; x1<1000; x1=x1+s) { push(); println("x1 =" +x1); translate(x1, y1); patternTop(); pop(); } } } void patternBase() { noStroke(); fill(#B1C5CB); //blue rectangle rect(10, 10, s-20, s-20); color[] arcColor = new color[3]; arcColor[0] = #DE9F60; //orange arcColor[1] = #FAEFE1; //white arcColor[2] = #6F6252; //brown noStroke(); fill(arcColor[int(random(0, 3))]); arc(10, 10, 80, 80, 0, HALF_PI); noStroke(); fill(arcColor[int(random(0, 3))]); arc(90, 90, 80, 80, PI, PI+HALF_PI); noStroke(); fill(arcColor[int(random(0, 3))]); arc(10, 90, 80, 80, PI+HALF_PI, 2*PI); noStroke(); fill(arcColor[int(random(0, 3))]); arc(90, 10, 80, 80, HALF_PI, PI); } void patternTop() { //float[] d1 = new float[ noStroke(); fill(#FAEFE1); circle(0, 0, centralC); middleStar(); } void middleStar() { push(); translate(0, -30); rotate(radians(45)); noStroke(); fill(#EAC89F); rect(0, 0, 40, 40); noStroke(); fill(#FAEFE1); arc(20, 40, 40, 20, PI, 2*PI); noStroke(); fill(#FAEFE1); arc(0, 20, 20, 40, PI+HALF_PI, 2*PI+HALF_PI); noStroke(); fill(#FAEFE1); arc(20, 0, 40, 20, 0, PI); noStroke(); fill(#FAEFE1); arc(40, 20, 20, 40, HALF_PI, PI+HALF_PI); pop(); }
Questions
Q1: In the reading “Art, Interaction and Engagement” by Ernest Edmonds, he identifies four situations in an interactive artwork: ‘Static’, ‘Dynamic-Passive’, ‘Dynamic-Interactive’ and ‘Dynamic-Interactive(Varying)’. From the exercise you did today which situations you identify in every part you executed? Explain.
In the exercise, part 1 lay in the situation of “Static” since “the art object does not change” (Edmonds). There was no interaction between the audience and the object. My non-moving patterns were just like the nonmoving artworks hung in the galleries that can only be viewed by the visitors despite “the viewer may be experiencing personal psychological or emotional reactions” (Edmonds). When I moved on to Part 2, the work also got into the situation of “Dynamic-Interactive”. In this part, the human still does not influence the work. Yet, the work contains internal passive variations. In my work, the patterns change colors only in response to the “internal mechanisms” (Edmonds) of the coding instructions. We are still externally observing the internal interactions of the work itself. The interaction of my work in this exercise ceases by “Dynamic-Interactive”.
Q2: What is the benefit of using arrays? How might you use arrays in a potential project?
By using arrays, we can create and hold any data like the drawers with a lot of small space to store things. Each element, or each drawer, can be individually and separately assigned with information. This will eliminate a lot of unnecessary and repetitive lines of code. When we need any of the data, we can just simply take out and assign the different information from the drawers that we stored in the array before. For example, we can assign arrays with the list of different speeds to control the ten circles moving at different speeds as well. In future projects, I might use arrays when I want Processing to produce images of different animals walking across the screen automatically. I could assign each animal picture in each element [ ] and make the Processing randomly select one to enter the screen.