Project Link and Brief Description
Here is the p5 link: https://editor.p5js.org/CassieHuang/sketches/YxHUzLxXi.
This mini-project presents an engaging and self-contained animated character that draws inspiration from the beloved Patrick Star character from SpongeBob SquarePants. The character is designed to exhibit rhythmic movements in its arms and legs, imparting a sense of dynamism and entertainment.
Key Features:
- Dynamic Movements: The character is programmed to perform rhythmic arm and leg movements, adding a playful and lively dimension to its presence.
- Interactive Element: The project includes an interactive component. By simply clicking the mouse, users can trigger a change in the character’s facial expression. Specifically, the character’s eyes change in size, conveying an expression of surprise.
- Object-Oriented Programming (OOP): The codebase of this project adheres to the principles of Object-Oriented Programming (OOP), ensuring a well-structured and organized framework. This makes it easy to extend and maintain the project, and allows the character to be seamlessly integrated into larger scenarios later.
Demo
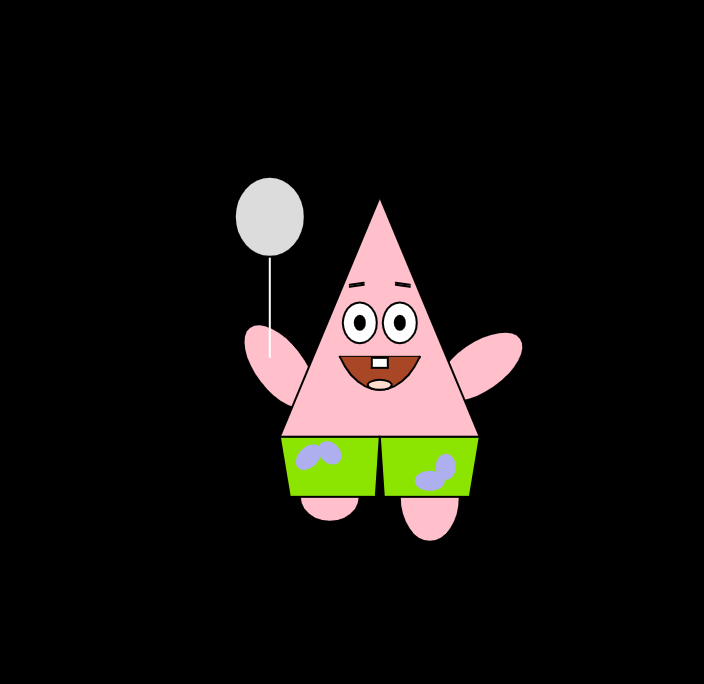
Dancing Patrick Star
Coding Snippet
In the project, the properties and attributes of the Patrick Star have been meticulously defined. Various properties, such as ‘this.yMov’ and ‘this.leftArmR,’ have been assigned specific roles and behaviors, enabling precise control over different aspects of Patrick’s appearance and dance movements.
To achieve the character’s engaging dance movements and the interactive feature, the code relies on a strategic utilization of mathematical functions. The ‘sin(frameCount/num)’ function plays a central role in generating rhythmic and repetitive motions. These movements, including Patrick’s arm and leg swings, are carefully synchronized with the passage of time, creating an animated and lively dance performance.
class Patrick{ constructor(startX, startY){ this.x = startX; this.y = startY; this.yMov= random(5,20); this.leftArmR = 5; this.Boo = false; this.rightArmR = 175; this.eyeSize = 15; this.legLeft = 25; this.legRight = 45; } update(){ this.x += 2*sin(frameCount/20) this.y += 2*cos(frameCount/this.yMov) if (this.leftArmR>70){ this.Boo = true } else if (this.leftArmR<5){ this.Boo = false } if (this.Boo) { this.leftArmR = this.leftArmR - noise(6 * sin(frameCount / 50)); this.rightArmR = this.rightArmR + noise(6 * sin(frameCount / 30)); } else{ this.leftArmR = this.leftArmR + noise(6 * sin(frameCount / 50)); this.rightArmR = this.rightArmR - noise(6 * sin(frameCount / 30)); } if (frameCount % 10 == 0){ if (this.legLeft == 25 ){ this.legLeft += 15 this.legRight -= 15 } else{ this.legLeft -= 15 this.legRight += 15 } } if (mouseIsPressed){ this.eyeSize += sin(frameCount / 2) } } } }
Reflection
- A self-contained class operates as an independent unit, with all its logic (like properties and methods) encapsulated in its own definition. This isolation reduces the risk of external factors affecting its behavior, making it more predictable and easier to maintain. As a result, an individual class can be reused in different projects without worrying about dependencies on external code. When we introduce this Patrick Star Dancer into the Grand Dance Party project, we will minimize the mess and trouble.
- Ensuring that my dancer’s code works seamlessly with code from a different source, such as the Grand Dance Party, requires a deep understanding of the structure and functionality of the external code in order to effectively integrate my code. Lack of comprehensive documentation or unclear code can make coordination challenging.
- Modularity: The Patrick class is an example of modularity, encapsulating the full properties and functionality of the Dancer character in a well-defined class. It separates the character’s properties, appearances, and movements into separate modules, making it easy to manage and maintain different aspects of the character independently.
- Reusability: The design of the Dancer class promotes reusability. People can instantiate multiple instances of the class in different scenarios without worrying about external dependencies. It is a self-contained, reusable unit that ensures that the character’s dance animations and interactions can be effortlessly integrated into different contexts.