Project Link and Brief Description
https://editor.p5js.org/CassieHuang/sketches/qrfwThnh7
This sketch allows users to draw on the canvas using the mouse while controlling the stroke color, thickness, and drawing shape (line or ellipse) with keyboard interactions.
Visual Output
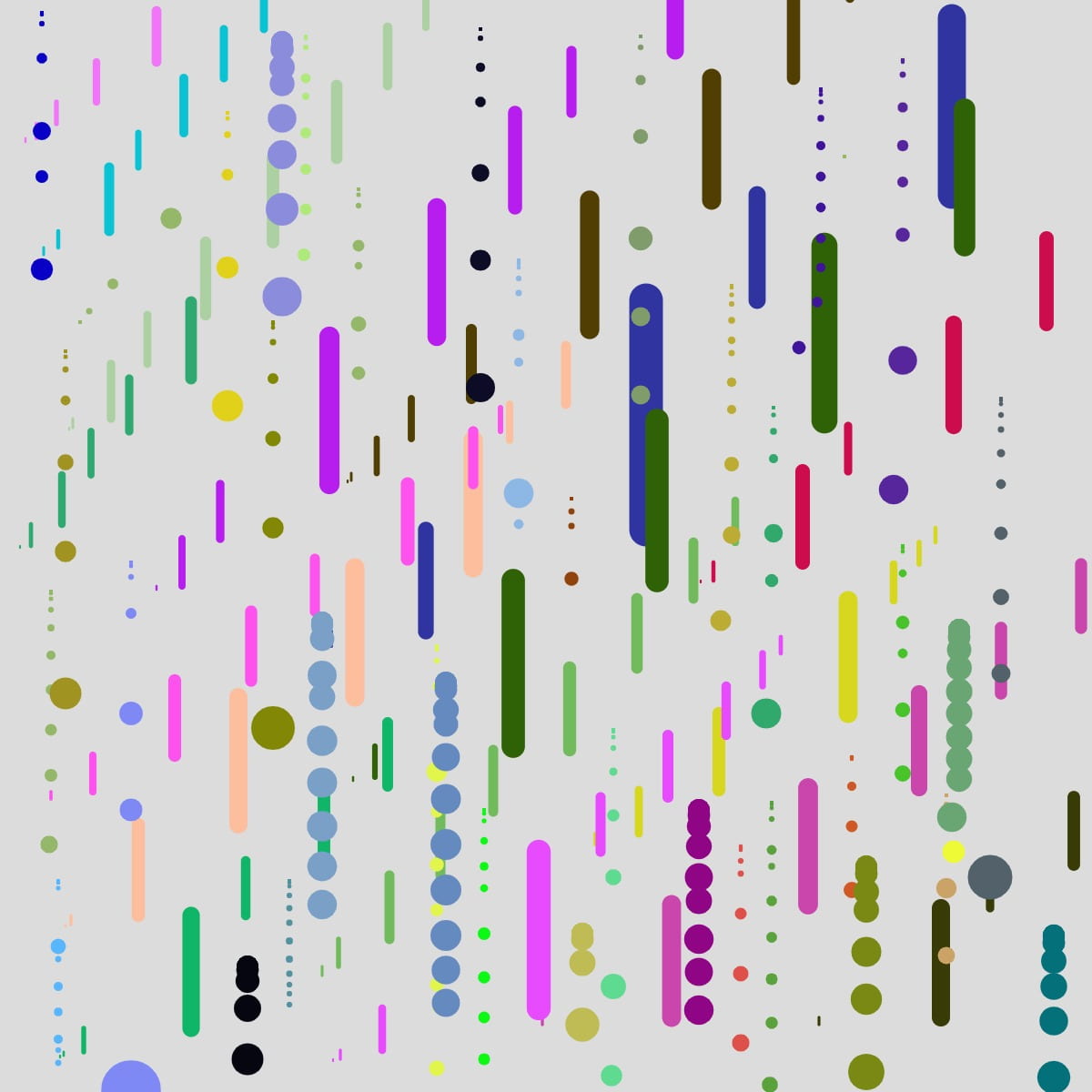
Cassie’s Drawing
Coding
Here is my code:
let r, g, b, thickness, boo, backGroundColor, d, temp function setup() { createCanvas(600, 600); backGroundColor = 220 background(backGroundColor) r = random(255) g = random(255) b = random(255) thickness = 1 temp = 1 boo = true } function draw() { if (keyIsPressed){ r = random(255) g = random(255) b = random(255) if (keyCode == ENTER){ background(backGroundColor) } if (key == " "){ r=g=b=0; } if (keyCode == UP_ARROW){ temp += 1 } else if (keyCode == DOWN_ARROW){ temp -= 1 } if (keyCode == LEFT_ARROW || keyCode == RIGHT_ARROW){ boo = !boo } } if (mouseIsPressed){ stroke(r,g,b) let d = dist(pmouseX, pmouseY, mouseX, mouseY); thickness = temp + map(d, 10, 50, 1, 5); strokeWeight(thickness) if (boo){ line(mouseX,pmouseY,mouseX,mouseY) } else{ fill(r,g,b) ellipse(mouseX,mouseY,thickness) } } }
Control and Unpredictability
I had initially envisioned that every time a user pressed the Left-Arrow or Right-Arrow keys, the shape they were about to draw would transition between a line and a circle. To implement this, I utilized a conditional statement: “If (keyIsPressed){ if (keyCode == LEFT_ARROW || keyCode == RIGHT_ARROW){}}.” My intention was to toggle a Boolean value to achieve this transition seamlessly. However, the results diverged from my expectations. Occasionally, even when I pressed the left or right arrow keys, the primitive shape did not change as anticipated. Upon conducting a thorough exploration and engaging in discussions with a fellow, we discovered that the “If (keyIsPressed)” statement did not consistently execute only once when the key was pressed, contributing to the unexpected behavior.
Reflections
How I use variables and conditions in the project:
- Initialization: The setup() function is used to set up the canvas and initializes various variables like backGroundColor, r, g, b, thickness, temp, and boo.
- Interaction with the Keyboard:
- If the ENTER key is pressed, it resets the canvas to its original background.
- If the spacebar is pressed, it sets the stroke color to black.
- If UP_ARROW or DOWN_ARROW is pressed, it changes the temp variable by 1, and thus changing the strokeWeight.
- If LEFT_ARROW or RIGHT_ARROW is pressed, it toggles the boolean variable boo, and thus the shape users were about to draw would transition between a line and a circle.
- When the mouse is pressed:
- It sets the stroke color to the current values of r, g, and b.
- It calculates the thickness of the stroke based on the velocity of the movement of mouse. The velocity is determined by the distance between the current mouse position and the previous mouse position.
- The temp variable also affects the thickness of strokeWeight.
- Depending on the value of boo, it either draws a line or an ellipse at the current mouse position.
Differences between key and keycode:
- Key variable is the actual character representation of the stored key. This variable is often used when you need to respond to specific character input, such as typing text or creating keyboard-controlled games.
- keyCode variable stores the numeric code of the key that was pressed or released. It is is useful for handling non-character keys, such as arrow keys (e.g. UP_ARROW, DOWN_ARROW) and special keys (e.g. ENTER, SHIFT).