This week our assignment was to either re-work or create a new script using functions to compartmentalize our code. I like starting from scratch so that I can redo things I’ve already learned while adding new features. So here we go! The leaves are changing here in NYC, so I thought, why not do a Fall theme.
To start, I wanted to make static leaves of varying colors. I created a function called “leaf” within it; I drew the leaf shape, gave them random fall colors, and placed them randomly throughout the frame.
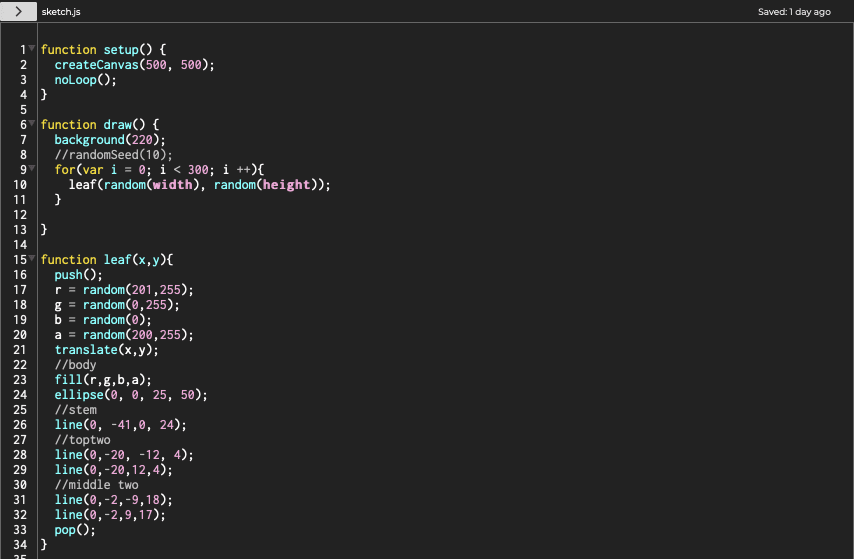
I was happy with what I had so far, but I wanted to add some animation or movement to the piece. Ideally, I wanted the leaves to change color and fall. To tackle this, I started researching how to make the leaves change over time. First, I tried LerpColor. Below is the description from the P5JS reference page.
“Blends two colors to find a third color somewhere between them. The amt parameter is the amount to interpolate between the two values where 0.0 equal to the first color, 0.1 is very near the first color, 0.5 is halfway in between, etc. An amount below 0 will be treated as 0. Likewise, amounts above 1 will be capped at 1. This is different from the behavior of lerp() but necessary because otherwise, numbers outside the range will produce strange and unexpected colors.”
I couldn’t get this to work for me and thought it had to do with the fact that I called noLoop() at the beginning of my script. Next, I tried using an if statement and framerate(). Again, I couldn’t seem to get it to work.
I needed another set of eyes on my problem, so I reached out to Peiling Jiang at the Coding Lab. We had a great hour-long conversation, and since I had already started reading about classes and arrays, he thought we could incorporate them into my project.
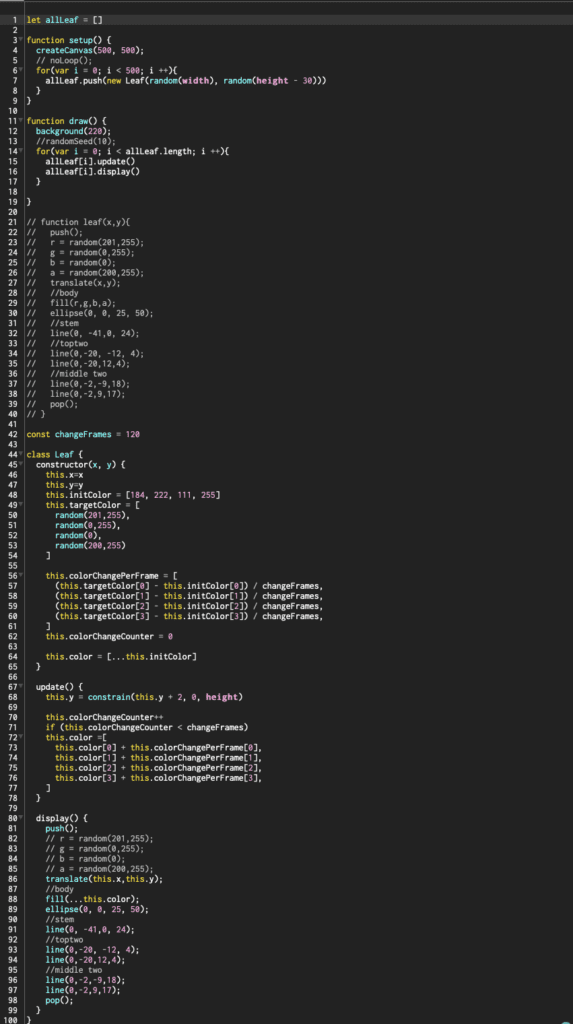
Slowly with his incredible patients, we made our way through the project. First, we made a class for leaf rather than just a function. Inside that class, we could do everything I wanted to do from having the leaves color change to make them fall based on the script’s framerate.
All in all, it was a success, and a big thanks go out to Peiling for help.