Labs with Tone Output: This week worked with analog output. I used tone as an output as well as different sensors such as a light sensitive sensor to change the tone’s frequency.
Below is a video from using tone output with a potentiometer to vary the frequency change.
I then changed the potentiometer to a light sensor to vary the frequency on the tone output, also seen below:
My hand covering the light resistor, lowered the frequency (covering light from coming in).
Creative Project: I decided to create a box that opens with a servomotor controlled by a potentiometer:
For people too lazy to open the tea box themselves, or maybe a candy dispenser.
Materials: Lipton tea box, two Q tips, Arduino Uno, servomotor, potentiometer.
Problems: The box lid was too light, so it was always slightly open making the servomotor’s effect not as visible. I tried fixing this by taping a penny onto the lid to make it slightly heavier.
The servomotor responded to the direction of movement by the potentiometer, but sometimes it would also jerk quickly in the opposite direction. I don’t know if this was a problem with my servomotor? However, the next day, it didn’t repeat this problem (code stayed the same).
I had to keep tweaking my values with mapping in the code while also adjusting the servomotor correctly so it would open and close correctly. I think what confuses me the most is knowing where the 180 degree angle starts and ends on the servomotor?
Code: Code was taken and adapted from Arduino.cc
//first include servo library
#include <Servo.h>
Servo servoMotor;
void setup() {
//Servomotor is attached at pin 9 on Arduino
servoMotor.attach(9);
}
void loop() {
int sensor = analogRead(A0);
//mapping servomotor angle to 180 from sensor
int servoAngle = map(sensor, 0, 1023, 70, 180);
if(millis() %20 < 2) {
servoMotor.write(servoAngle);
}
}
Schematic Diagram of Phase 1 (before tone was added):
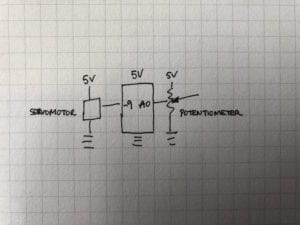
Schematic Diagram for microcontroller and potentiometer on Arduino
Building Process:
Phase 1: I Connected the servomotor and potentiometer to the Arduino with the correct wiring and put the servomotor in the tea box.
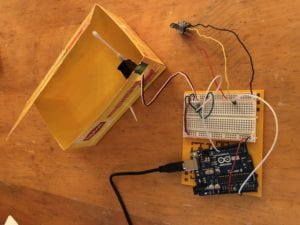
Phase 1 of Opening Box with Servomotor
Phase 2: Below is a video of opening and closing the box with the servomotor. I was tweaking the numbers in my map function in the code to get the angle right. There was a problem with my paper and coin getting in the way – I’ll have to smooth out the surface.
Phase 3: I covered the box in paper, to smooth out the surface so nothing interfered with the servomotor from opening the box smoothly. I then tried adding an alarm system (tone output) to my project.
I added to my code to make it so that when the servomotor was at an angle (so that the lid was open), the alarm would sound. I made the tone to sound anything above 170 (out of 180), but when I tested it, the sound was constantly buzzing. I didn’t know what was wrong until wrote: Serial.println(servoAngle) and looked at the serial monitor and realized when the servoAngle was at 0, it was opening the box, so I had flipped it (thinking 180 was the servoAngle opening the box). Knowing this, I adjusted the numbers and got it to work!
New code bit added below:
if (servoAngle >=5 && servoAngle <=30) {
tone(5, 700);
} else {
noTone(5);
}
Serial.println(servoAngle);
}
Final Phase: Below is a video of the box opening and the alarm sounding when it is fully opened:
I still experienced a lot of jittering with the servomotor, even when I wasn’t touching the potentiometer and also when I added code to delay it:
Leave a Reply