CONCEPT
The concept of my project was to make a coin sorting and counting machine. I started off with the idea of there being only one open slot where you could in any coin. Once inside the mechanism, the coins would get sorted based on their size and fall into their respective columns. Each column has a sensor that would detect the falling coin and add it up to the total on the LCD screen. While executing this idea, however, I faced a lot of problems in sorting the coins based solely on their size as there were a few instances where the coins would fall into the wrong column and that would mess up everything. Therefore, I decided to remove the coin sorting aspect of it and only make a mechanism to count the coins and calculate the total amount of money that has been counted. There are now two slots, one for 50 fils coins and one for 1 dirham coins and the user is supposed to put each coin in its respective slot.
SCHEMATIC
PROGRAM
#include <LiquidCrystal.h> // initialize the library by associating any needed LCD //interface pin with the arduino pin number it is connected //to const int rs = 12, en = 11, d4 = 5, d5 = 4, d6 = 3, d7 = 2; LiquidCrystal lcd(rs, en, d4, d5, d6, d7); // defining counters int one_dirham_count = 0; int big_fil_count = 0; float total = 0; // defining sensor pins const int one_dirham_sensor = 7; const int big_fil_sensor = 8; void setup() { Serial.begin (9600); pinMode (one_dirham_sensor, INPUT); pinMode (big_fil_sensor, INPUT); pinMode (dirham_button, INPUT); pinMode (fil_button, INPUT); Serial.begin(9600); // LCD Screen // set up the LCD's number of columns and rows: lcd.begin(16, 2); // Print a message to the LCD. lcd.print("You have: "); } void loop() { if (digitalRead(one_dirham_sensor) == 0) { one_dirham_count += 1; total += 1; delay(100); } else { one_dirham_count += 0; total += 0; } if (digitalRead(big_fil_sensor) == 0) { big_fil_count += 1; total += 0.50; delay(100); } else { big_fil_count += 0; total += 0; } // Practice code used while setting up the sensor // Serial.print ("One Dirham Sensor: "); // Serial.println (digitalRead(one_dirham_sensor)); // Serial.print ("Fifty Fils Sensor: "); // Serial.println (digitalRead(big_fil_sensor)); // Serial.print ("Twenty Five Fils Sensor: "); // Serial.println (digitalRead(small_fil_sensor)); // Serial.println (one_dirham_count); // Serial.print ("You have AED "); // Serial.println(total); // delay (300); // LCD Screen Printing // set the cursor to the second line lcd.setCursor(0, 1); // print the total amount: lcd.print("AED "); lcd.print(total); }
BEHAVIOUR
I have used two infrared obstacle avoidance sensors to read a coin falling down. Whenever any of the sensors read something (when a coin falls down in front of it), it adds the respective amount (1AED or 50 fils) to the counter which gets displayed on the LCD screen as the total amount counted.
PROBLEMS AND SOLUTIONS
There were three major problems that I faced during this project. The first one was building the correct mechanism to separate coins based solely on their size. I had a rectangular piece of cardboard with different sized cut out for three different coins. However, the 25 fils and 50 fils coins are too similar in size for such a mechanism to work. I then decided to keep away the 25 fils coin and only work with two coins of very different sizes. Even though the sorting worked most of the times, there were a few instances where the one dirham coin would sway a little to the left while going downwards and fall into the wrong slot. I wanted to build something that would be full-proof and therefore decided to discard the idea of sorting the coins. The other two problems that I faced was adjusting the range of the sensor so that it doesn’t sense any other part of the mechanism and having the coin fall exactly in front of the sensor. It took a lot of trial and error to solve these two problems but it worked out at the end.
PHOTOGRAPHS
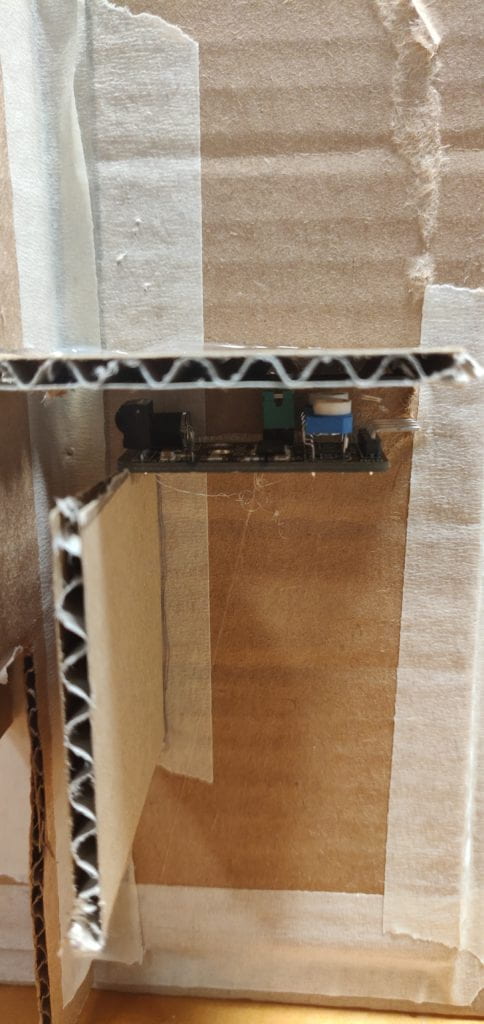
Sensor used
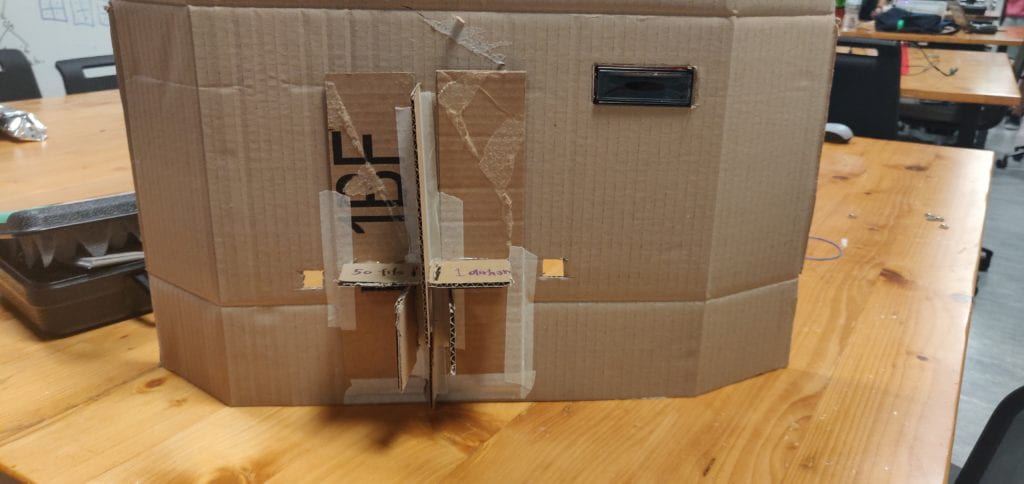
The coin counter
VIDEO
LESSONS LEARNED
One major lesson that I learnt through this project was that I need to give a lot more time to making the physical machine especially if I want to make it look aesthetically pleasing. For this project, I spent a few hours everyday for four days but even that was not enough. At every step of making it, I faced some unexpected problems and it would take a lot of time to solve them.